How to Check Box onChange in React
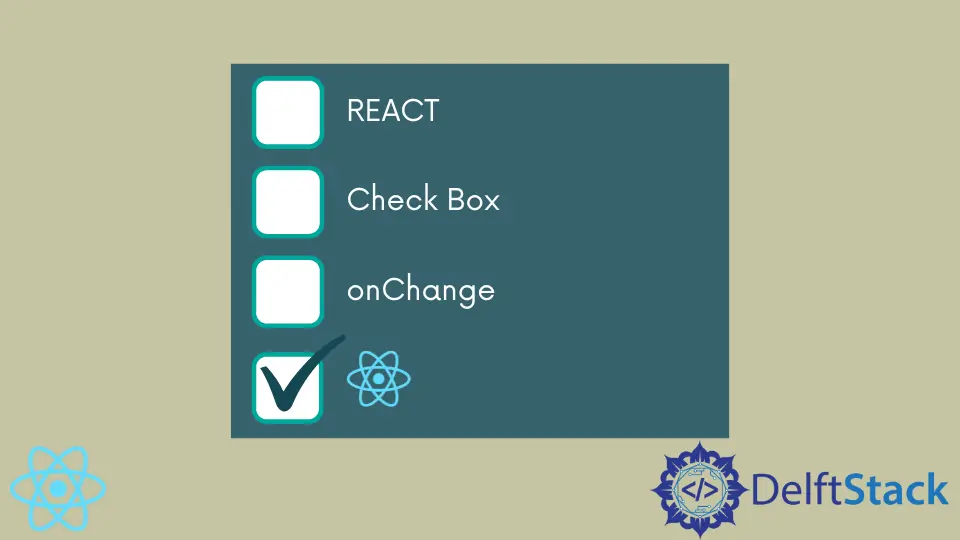
We will introduce how to send values from the checkbox on the onChange
event in React.
Send Values From the Checkbox on the onChange
Event in React
When developing a web application or commercial software, we need to use checkboxes to display a list of options, and the users can select from those number of choices.
Users can select one to several options from the lists of options, and there is no limit to only one checkbox can be selected, but users can even select all of the checkboxes.
Each checkbox is independent of all other checkboxes in the list, so checking one box doesn’t uncheck the other checked checkboxes.
This tutorial will create a list of checkboxes and send them to the getValue
function to console.log
their values. Let’s create a new application using the command below.
# react
npx create-react-app my-app
After creating the new React application, we will go to the application directory using this command.
# react
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# react
npm start
Let’s create a list of checkboxes with different values with an onChange
method calling the function checkValue
.
# react
<div className="App">
<h1>Select What You Want</h1>
<div>
<input id="box1" onChange={checkValue} type="checkbox" value="Box1"/>
<label htmlFor="#box1">Box 1</label>
</div>
<div>
<input id="box2" onChange={checkValue} type="checkbox" value="Box2"/>
<label htmlFor="#box2">Box 2</label>
</div>
<div>
<input id="box3" onChange={checkValue} type="checkbox" value="Box3"/>
<label htmlFor="#box3">Box 3</label>
</div>
<div>
<input id="box4" onChange={checkValue} type="checkbox" value="Box4"/>
<label htmlFor="#box4">Box 4</label>
</div>
</div>
Once we have created a list of checkboxes with the method of onChange
calling the function checkValue
, we will create the function checkValue
that will take the parameter e
containing the value of the checked checkbox, and we will console.log
the checkbox value checked by the user.
# react
function checkValue(e) {
var value = e.target.value;
console.log("You selected " + value);
}
Output:
As shown above, when we check any checkbox, it console.log
the value of that checkbox.
If we use onChange
to send checkbox values, there is one issue. If we uncheck any of the checkboxes, the function checkValue
will again be called, and we will receive the value of the checkbox unchecked by the user, as shown below.
Output:
As shown above, when we uncheck box 1, the value of box 1 is sent again to the function. If we are working with multiple checkboxes and want to save the values of the checkboxes, it will not be the best way to get and save values.
The best way to get and save the values of the checkboxes will be through a form.
But if we are working with something that will work with one checkbox and entirely depends on the state change of that checkbox, then it can be a good option to use the onChange
method to execute certain functions.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn