How to Render Component via onClick Event Handler in React
- Understanding the onClick Event Handler
- Rendering Multiple Components Conditionally
- Managing State with Complex Components
- Conclusion
- FAQ
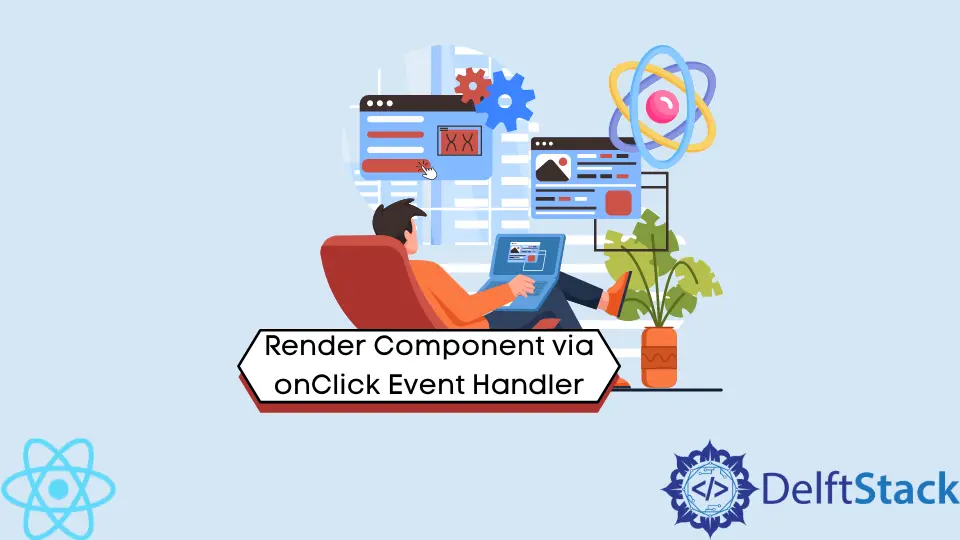
In the world of React, interactivity is key to creating a dynamic user experience. One common requirement is rendering components conditionally based on user actions, such as button clicks.
This article will guide you through the process of setting up React components to render conditionally using the onClick event handler. We’ll explore how to manage state effectively, using hooks like useState, and demonstrate practical examples to solidify your understanding. By the end of this article, you’ll be equipped with the knowledge to enhance your React applications, making them more engaging and responsive to user interactions.
Understanding the onClick Event Handler
React’s onClick event handler allows you to execute a function when a user clicks on an element. This functionality is pivotal when you want to trigger changes in your component’s state or render new components. To get started, you’ll need to create a functional component and set up an event handler that modifies the state.
Here’s a simple example to illustrate this concept. We’ll create a button that, when clicked, toggles the visibility of a text component.
import React, { useState } from 'react';
const ToggleComponent = () => {
const [isVisible, setIsVisible] = useState(false);
const handleClick = () => {
setIsVisible(!isVisible);
};
return (
<div>
<button onClick={handleClick}>
{isVisible ? 'Hide' : 'Show'} Text
</button>
{isVisible && <p>This is a toggleable text component.</p>}
</div>
);
};
export default ToggleComponent;
Output:
This is a toggleable text component.
In this example, we import React and the useState hook. The ToggleComponent
functional component initializes a state variable isVisible
with a default value of false
. The handleClick
function toggles the value of isVisible
when the button is clicked. The button’s label changes based on the visibility of the text, which is conditionally rendered using a simple logical AND operator. This approach not only enhances user interaction but also keeps your UI clean and responsive.
Rendering Multiple Components Conditionally
Sometimes, you may want to render multiple components based on different user actions. In this case, you can utilize an array of components and an index to determine which component to display. This setup allows for a more dynamic interface where users can navigate through various options.
Here’s how you can implement this:
import React, { useState } from 'react';
const components = [
<div key="1">Component 1: Welcome to our application!</div>,
<div key="2">Component 2: Here is some more information.</div>,
<div key="3">Component 3: Thank you for visiting!</div>,
];
const MultiComponentRenderer = () => {
const [currentIndex, setCurrentIndex] = useState(0);
const handleNext = () => {
setCurrentIndex((prevIndex) => (prevIndex + 1) % components.length);
};
return (
<div>
<button onClick={handleNext}>Next Component</button>
{components[currentIndex]}
</div>
);
};
export default MultiComponentRenderer;
Output:
Component 1: Welcome to our application!
In this code, we define an array of components, each with a unique key. The MultiComponentRenderer
component maintains a currentIndex
state to track which component is currently displayed. The handleNext
function increments the index and wraps around when reaching the end of the array. When the button is clicked, the next component in the array is rendered. This method enhances user experience by allowing seamless transitions between different content sections.
Managing State with Complex Components
As your application grows, you might encounter scenarios where you need to manage more complex states. For instance, consider a situation where you want to render different components based on user input. You can achieve this by combining the onClick event with controlled components.
Here’s a practical example:
import React, { useState } from 'react';
const ComponentA = () => <div>This is Component A</div>;
const ComponentB = () => <div>This is Component B</div>;
const ComplexComponentRenderer = () => {
const [selectedComponent, setSelectedComponent] = useState('');
const handleSelect = (component) => {
setSelectedComponent(component);
};
return (
<div>
<button onClick={() => handleSelect('A')}>Show Component A</button>
<button onClick={() => handleSelect('B')}>Show Component B</button>
{selectedComponent === 'A' && <ComponentA />}
{selectedComponent === 'B' && <ComponentB />}
</div>
);
};
export default ComplexComponentRenderer;
Output:
This is Component A
In this example, we define two simple components, ComponentA
and ComponentB
. The ComplexComponentRenderer
component manages the selectedComponent
state. The handleSelect
function updates this state based on which button is clicked. Depending on the state, either ComponentA
or ComponentB
is rendered. This pattern is particularly useful in forms or wizards where users make selections that dictate the next steps.
Conclusion
In this article, we explored how to render components conditionally in React using the onClick event handler. We discussed various methods, from toggling visibility to rendering multiple components based on user interactions. By leveraging React’s state management with hooks like useState, you can create dynamic and engaging user experiences. As you continue to build your React applications, remember that understanding these fundamental concepts will empower you to develop more interactive and user-friendly interfaces.
FAQ
-
What is an onClick event handler in React?
The onClick event handler is a function that executes when a user clicks on an element, allowing you to trigger state changes or render different components. -
How do I manage state in a functional component?
You can manage state in functional components using the useState hook, which allows you to declare state variables and update them as needed. -
Can I render multiple components using a single button?
Yes, you can render multiple components by maintaining an index or state that determines which component to display based on user interactions. -
What are the benefits of conditional rendering in React?
Conditional rendering allows for a more dynamic user experience by displaying different components based on user actions or application state, leading to a more interactive interface. -
How can I improve performance when rendering components conditionally?
You can improve performance by using React’s memoization techniques, such as React.memo, to prevent unnecessary re-renders of components that haven’t changed.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn