The onDoubleClick Event in React
-
Understanding the
onDoubleClick
Event in React -
Use the
onDoubleClick
Event in React - Best Practices and Considerations
- Conclusion
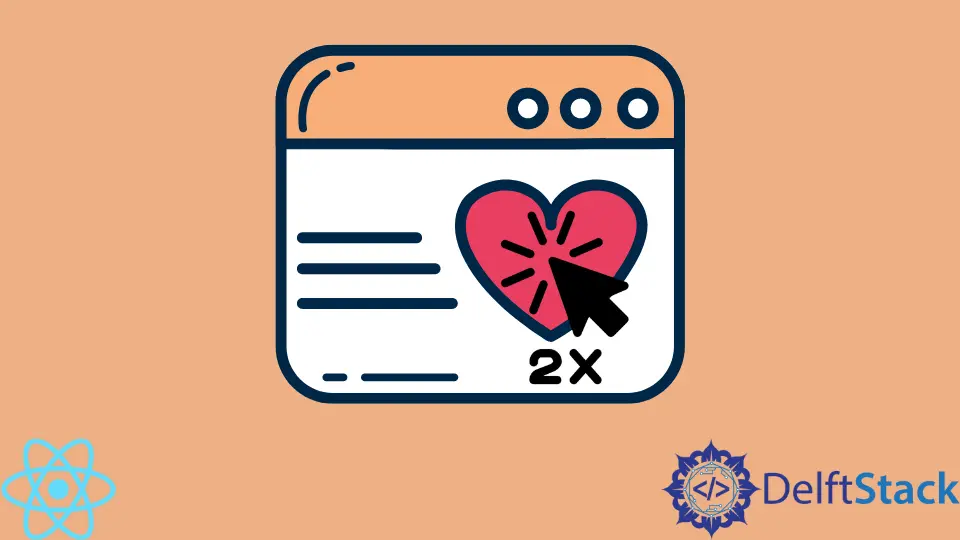
React, a popular JavaScript library for building user interfaces provides a rich set of event handling capabilities. One such event, onDoubleClick
, allows developers to respond to a double-click event.
We will introduce how to use the onDoubleClick
event in our React application and use onClick
and onDoubleClick
on the same button.
Understanding the onDoubleClick
Event in React
The onDoubleClick
event is triggered when a user clicks an element twice in rapid succession. It provides a powerful way to capture user interactions that require a double click, such as opening a modal or initiating an action.
Syntax:
In React, onDoubleClick
is used as an attribute in JSX, and its value is set to a function that will be executed when the double click event occurs.
<button onDoubleClick={handleDoubleClick}>Double Click Me</button>
Here, handleDoubleClick
is the function that will be called when the button is double-clicked.
Use the onDoubleClick
Event in React
When developing a web application or commercial software, we need to use onClick
events to perform many functions. But sometimes, we may need to call a function on double click or disable a function on double click.
React gives us the onDoubleClick
that can be activated whenever a user clicks two times on the element, to which we have attached an onDoubleClick
method.
To demonstrate the usage of onDoubleClick
, let’s walk through a practical example of a React component that responds to a double-click event.
So, let’s create a new React application by using the following command:
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command:
cd my-app
Let’s run our app to check if all dependencies are installed correctly:
npm start
We will return a button in App.js
with an onClick
event of function handleDoubleClick()
.
<button onDoubleClick={handleDoubleClick}>Click This Button Twice</button>
Let’s create the function handleDoubleClick()
that will console.log
a value.
function handleDoubleClick() {
console.log("You Double Clicked!");
}
Let’s add some styles to our buttons using CSS.
button {
background: black;
color: white;
border: none;
padding: 15px 15px;
}
button:hover {
background: white;
color: black;
border: 1px solid black;
}
Output:
We can easily create any onDoubleClick
events on buttons and pass any functions using these simple steps.
Now let’s check how our button will respond when we add both onClick
and onDoubleClick
. We will include an onClick
event on the same button, and our code will look like below.
<button onClick={singleClick} onDoubleClick={handleDoubleClick}>
Click This Button Twice
</button>
Once we have added the onClick
method, we will create a function singleClick
that will be called when this button is clicked once.
function singleClick() {
console.log("You clicked One Time");
}
Output:
As shown above, both methods can work on the same button but not accurately because when we click once on the button, the onClick
method is executed.
But when we click twice on the button, the onClick
method is executed twice, and the onDoubleClick
method is executed. It is suggested not to use both methods on the same button because it will not function as intended.
Implementing Alert on the onDoubleClick
Event in React
Here is another demonstration of how to use the onDoubleClick
event in a React component. This component provides a simple UI with a button that triggers an alert when double-clicked.
import React from 'react';
class DoubleClickComponent extends React.Component {
handleDoubleClick = () => {
alert('You double-clicked!');
}
render() {
return (
<div>
<button onDoubleClick={this.handleDoubleClick}>
Double Click Me
</button>
</div>
);
}
}
export default DoubleClickComponent;
In this example, we have a DoubleClickComponent
class that extends React.Component
. It contains a method handleDoubleClick
that displays an alert when called.
Within the render
method, a button element is rendered. It has an onDoubleClick
attribute set to the handleDoubleClick
function.
This means that when the button is double-clicked, handleDoubleClick
will be executed.
Output:
Handling Event Parameters
The onDoubleClick
event handler can also receive event parameters, which can be useful for obtaining information about the event, such as the target element or coordinates.
handleDoubleClick = (event) => {
const targetElement = event.target;
const clientX = event.clientX;
const clientY = event.clientY;
console.log(`Double click at coordinates (${clientX}, ${clientY}) on element:`, targetElement);
}
In this modified handleDoubleClick
function, we receive an event
parameter. We then extract information about the target element and the client coordinates where the double click occurred.
Output:
Best Practices and Considerations
Debouncing
In situations where you want to handle double clicks but avoid rapid, unintended double clicks, consider using a debounce function to throttle the event handler.
Accessibility
Ensure that your double-click interactions are accessible to all users. Some users may not be able to perform double clicks, so provide alternative methods for performing the same action.
Testing
When working with onDoubleClick
events, thorough testing is crucial. Use testing libraries like Jest and React Testing Library to verify that your event handlers behave as expected.
Avoid Overuse
Double-click interactions can be powerful, but they can also be overused. Consider the user experience and whether a single click or other interaction might be more appropriate.
Conclusion
In conclusion, the onDoubleClick
event in React provides a powerful way to capture user interactions that require a double click. It enables developers to create dynamic and interactive user interfaces.
This article has covered various aspects of using onDoubleClick
, including its syntax, practical implementation, handling event parameters, and best practices.
We demonstrated how to use onDoubleClick
in a React application, along with cautionary advice about combining it with onClick
events on the same button. Additionally, we showcased an example component that triggers an alert on double click.
Remember to consider accessibility, debounce if necessary, and thoroughly test your event handlers. By following these best practices, you can ensure a seamless user experience in your React applications.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn