How to Link to Another Page in React
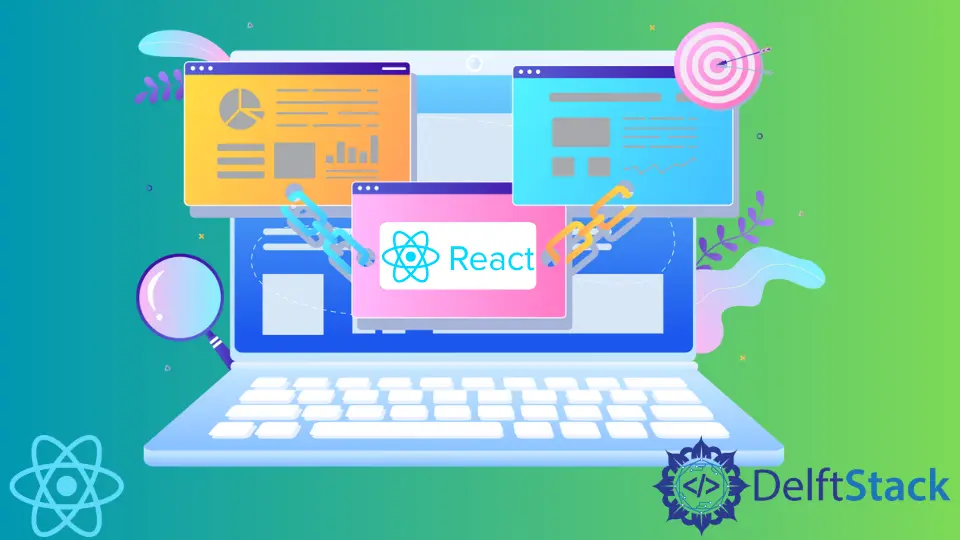
Links may be found all over the internet. They are critical in discovering resources like web pages, photographs, and videos, not to mention their significance in SEO.
Routing determines which code should be executed when a URL is visited. This article will teach you about how React’s routing works.
Links in React
As illustrated below, links in ordinary apps that do not use a library like React are built with an anchor tag.
<a href="https://Youtube.com">Go To Youtube</a>
Remember that this also works in React. This routing strategy triggers a full page refresh when the only thing that changes on the new route may be an image and some text.
A full-page refresh is beneficial in some situations, but it is rarely required.
Libraries like React Router
use this to let your app render components that need to be re-rendering depending on a given route.
Use react-router-dom
Package to Redirect to Another Page in React
Let’s look at how to use the react-router-dom
package to redirect to another page in ReactJS. The react-router-dom
is a reactJS package that allows you to construct dynamic routing on a web page.
First, create a react app and then add different components.
We have created two pages, Home.jsx
and About.jsx
.
Code - Home.jsx
:
import React from 'react';
import {Link} from 'react-router-dom';
const Home = () => {
return (<div><h1>Home Page</h1>
<p>You are in home Page</p><br /><ul>
<li><Link to = '/'>Home</Link>
</li><li>
<Link to = '/about'>About</Link>
</li></ul>
</div>);
};
export default Home;
Code - About.jsx
:
import React from 'react';
const About = () => {
return (
<div>
<h1>About Page</h1>
<p>This is about page</p>
</div>
);
};
export default About;
Use the react-router-dom
package to implement routing. This section will implement the react-router-dom
package in our React app.
To do so, we must import specific components from the react-router-dom
package, including BrowserRouter,
Routes,
and Route
.
We will alias BrowserRouter
as Router to keep things simple. Then, we go over each imported component one by one.
The BrowserRouter
keeps the UI in sync with the URL by utilizing the HTML5 history API. The Route
is responsible for rendering UI when its path matches the current URL, and Routes
displays the first child Route or Redirects corresponding to the location.
Here is the code for the App.js
file, in which we will implement the react-router-dom
package.
Code - App.js
:
import React from 'react';
import {BrowserRouter as Router, Route, Routes} from 'react-router-dom';
import About from './components/About';
import Home from './components/Home';
function App() {
return (
<>
<Router>
<Routes>
<Route exact path='/' element={
<Home />} />
<Route path="/about' element={<About />} /'
</Routes>
</Router>
</>
);
}
export default App;
Even though we’re connecting to React, the React Router
secretly rewrites it to respond, ensuring that our current URLs continue functioning correctly. It also implies that if you modify your server settings such that the entire is no longer required, those links will automatically update.
When you click on About,
it will redirect you to the About page:
That’s all. It would help if you now understood the notion of React links and how to manage routes efficiently.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn