Image Tag in React
- Method 1: Using the Image Tag
- Method 2: Importing Local Images
- Method 3: Using CSS Background Images
- Conclusion
- FAQ
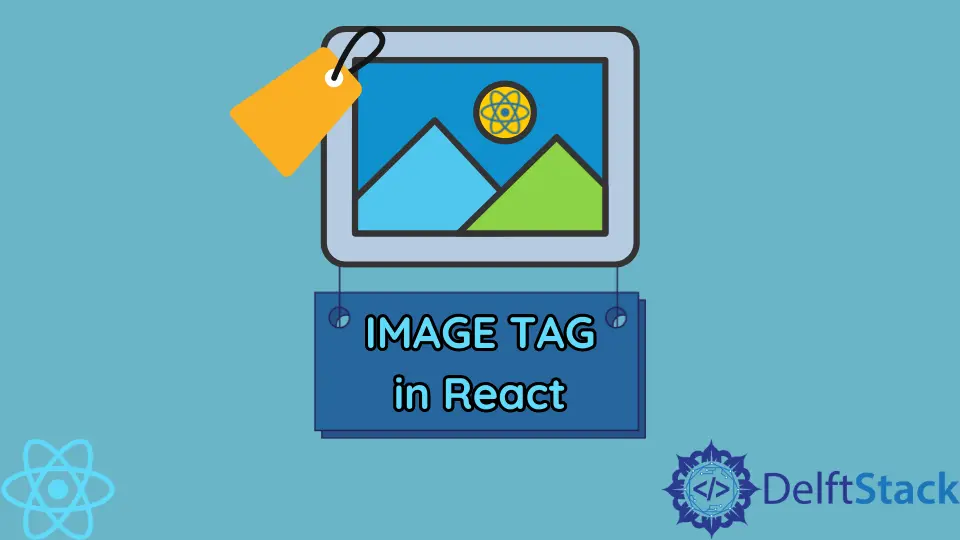
Adding images to your React applications can enhance user experience and make your content visually appealing. Whether you’re building a simple portfolio or a complex web application, knowing how to effectively incorporate images is essential.
In this tutorial, we’ll explore various methods to add images in React, covering everything from basic image tags to advanced techniques. By the end of this guide, you’ll be equipped with the knowledge to seamlessly integrate images into your projects, making them more engaging and user-friendly. Let’s dive into the world of images in React!
Method 1: Using the Image Tag
The most straightforward way to add an image in React is by using the HTML <img>
tag. This method is simple and effective for displaying images that are hosted online or stored locally within your project.
Here’s how you can do it:
import React from 'react';
function App() {
return (
<div>
<h1>Welcome to My React App</h1>
<img src="https://example.com/image.jpg" alt="Description of Image" />
</div>
);
}
export default App;
In this example, we import React and define a functional component named App
. Inside the component, we use the <img>
tag to display an image. The src
attribute points to the URL of the image, while the alt
attribute provides a textual description, which is important for accessibility.
Output:
Welcome to My React App
[Image displayed here]
Using the <img>
tag is a straightforward method that works well for most scenarios. However, if you want to include images stored in your local project directory, you can do so by importing them.
Method 2: Importing Local Images
When working with local images in a React project, you can import them directly into your component. This method allows you to keep your assets organized and ensures that your images are bundled with your application.
Here’s how to import a local image:
import React from 'react';
import myImage from './assets/myImage.jpg';
function App() {
return (
<div>
<h1>My Local Image</h1>
<img src={myImage} alt="My Local Image" />
</div>
);
}
export default App;
In this code snippet, we import the image from the assets
folder and assign it to the variable myImage
. We then use this variable in the src
attribute of the <img>
tag. This approach is beneficial because it helps to manage your assets effectively and ensures that your images are part of your build process.
Output:
My Local Image
[Local image displayed here]
Importing local images is particularly useful when you want to maintain control over your assets and ensure that they are optimized during the build process. Additionally, this method prevents issues related to broken links that can occur when using external URLs.
Method 3: Using CSS Background Images
Another effective method to add images in React is by using CSS background images. This approach is beneficial when you want to create visually appealing sections or components without relying solely on the <img>
tag.
Here’s an example of how to use CSS for background images:
import React from 'react';
import './App.css';
function App() {
return (
<div className="background-image">
<h1>Hello, World!</h1>
</div>
);
}
export default App;
And in your App.css
file:
.background-image {
background-image: url('./assets/background.jpg');
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
color: white;
text-align: center;
}
In this example, we create a div
with a class of background-image
. The CSS sets a background image using the background-image
property. The height
is set to 100vh
to cover the full viewport height, and we center the text inside the div
.
Output:
Hello, World!
[Background image displayed behind the text]
Using CSS background images can be particularly powerful for creating visually rich layouts. It allows for more flexibility in design, such as adding overlays, adjusting positioning, and creating responsive designs. This method is perfect for hero sections, banners, or any area where you want to enhance the visual appeal without relying solely on image tags.
Conclusion
Incorporating images into your React applications is a fundamental skill that enhances the user experience. Whether you choose to use the <img>
tag, import local images, or utilize CSS background images, each method has its unique advantages. By mastering these techniques, you can create visually stunning applications that engage users and convey your message effectively. Remember, the key is to choose the method that best suits your project’s needs and design goals. Happy coding!
FAQ
-
How do I add an image from a URL in React?
You can add an image from a URL using the<img>
tag and setting thesrc
attribute to the image URL. -
Can I use images stored in my local project?
Yes, you can import local images into your React components and use them in the<img>
tag. -
What is the advantage of using CSS background images?
CSS background images allow for more design flexibility, enabling overlays and responsive adjustments without relying on the<img>
tag. -
How do I ensure images are accessible?
Always use thealt
attribute in your<img>
tags to provide a description of the image for screen readers. -
Can I use images in React Native?
Yes, React Native supports images, but the syntax is slightly different, using theImage
component instead of the<img>
tag.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn