How to Resize Images in React
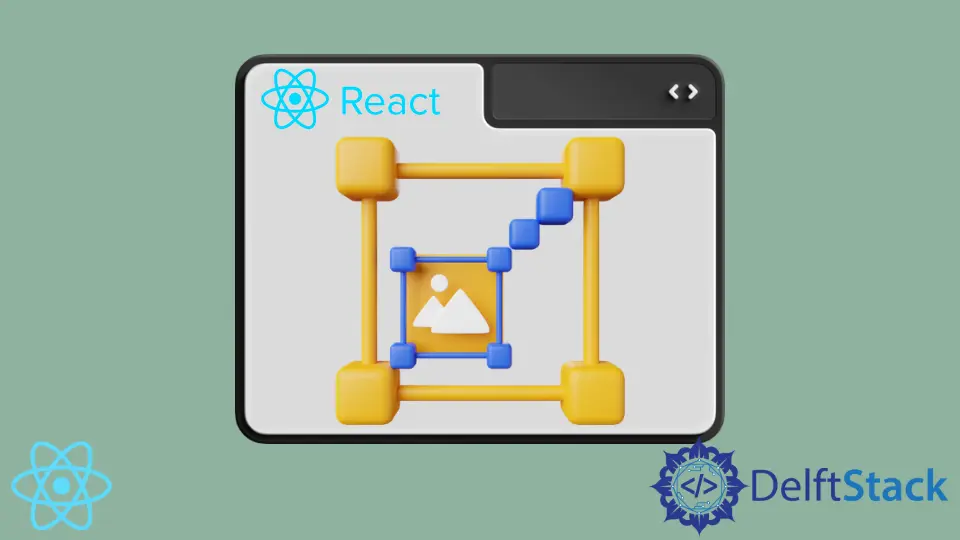
Almost all UX designers agree that content should contain visual elements to catch the readers’ attention. React is one of the most popular frameworks for building modern web applications.
Naturally, React developers have to find a way to include images in their applications. This article focuses on styling the images to adjust their size.
Resize Images in React
There are a lot of ways to resize images in React. You can use styles to specify their size or even let the users specify the absolute or relative size of images.
To style any element in React, you need to use CSS styles, which can be set using the className
and styles
attributes. The className
value needs to be a string that refers to a class definition in CSS files.
The styles
attribute value needs to be a JavaScript object, with key-value pairs equal to CSS rules.
When developing React applications, you should use the className
attribute instead of the class
, the traditional HTML attribute. If you try to use the class
attribute, it might work (depending on your version of React), but it will display a warning in the console.
A Basic Example of How to Resize Images in React
Here is a simple example of how to use CSS styles to change the appearance of an image.
img {
width: 200px;
height: 200px;
}
In this case, we select all img
elements in our application. Read this article if you want to learn more about CSS selectors, their specificity, etc.
This code example has a simple application that consists of just one img
element.
export default function App() {
return (
<div>
<img src="https://i.pinimg.com/564x/40/7e/e1/407ee19bfc18ed58afd556695524630c.jpg" />
</div>
);
}
If you look at the live demo on CodeSandbox, you’ll see that the image is 200 pixels wide and 200 pixels tall.
Pixels are the most basic units for setting the width and height of elements in React. Other units are relative and depend on other factors.
For instance, vh
(short for viewport height) depends on the height of the user’s screen. You can also set the percentage value, which tells CSS to ensure the element takes up the specified free space.
If you set the width to 50%, the element will take up 50% free space width in a container. To learn more about units of measurement in CSS, read this guide.
Image Size Properties in React and CSS
Modern web applications should be responsive. For instance, images should automatically change their sizes depending on whether users are browsing with a smartphone or personal computer.
To resize images in React, developers use CSS properties like min-height
, max-height
, min-width
, and max-width
. As you can probably guess, min-height
and min-width
set the image’s basic minimum size.
The elements will not become smaller than the specified values regardless of the user’s screen size. On the other hand, max-height
and max-width
define the maximum sizes the image can expand to.
You can use the border-radius
CSS property to give your images rounded corners. The value of 50% will give it a circle shape.
Inline Styles
Sometimes it’s better to set the styles for image elements quickly. In this case, you can use the styles
attribute in JSX.
Let’s take a look at a simple example.
<img
styles={{ minWidth: 300, minHeight: 300 }}
src="https://i.pinimg.com/474x/53/c3/3b/53c33b5e9758144727b99d2bcc141b1f.jpg"
/>
The value of the styles
attribute needs to be a JavaScript object. For this reason, there are multiple things to keep in mind.
First, in JSX, you must always wrap JavaScript expressions with a pair of curly braces. We have two pairs of curly braces in the example: one pair is there to define an object, and the other pair of curly braces tells JSX to treat it as a JavaScript expression.
Also, note that the CSS property min-width
becomes minWidth
. This is required because the CSS property name becomes a property of the styles
object.
The two words are pushed together and camelCased
.
The object is directly supplied as the styles
attribute value in the above examples. When there are too many properties, a more readable approach is defining the styles
object outside JSX and referencing it.
Let’s take a look at an example.
export default function App() {
const imageStyles = { minWidth: 300, minHeight: 300 };
return (
<div>
<img
className="sampleImg"
src="https://i.pinimg.com/564x/40/7e/e1/407ee19bfc18ed58afd556695524630c.jpg"
/>
<img
styles={imageStyles}
src="https://i.pinimg.com/474x/53/c3/3b/53c33b5e9758144727b99d2bcc141b1f.jpg"
/>
</div>
);
}
Here, we define the imageStyles
variable and set it equal to the object that contains CSS rules for styling the image. Within the return
statement, we reference it.
A live demo is available on CodeSandbox.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn