React Image src
-
Reference a Local Image in React Using the
create-react-app
Package -
Load an Image Using the
import
Statement in React -
Load an Image Using the
require()
Method in React -
How
import
andrequire()
Work in React
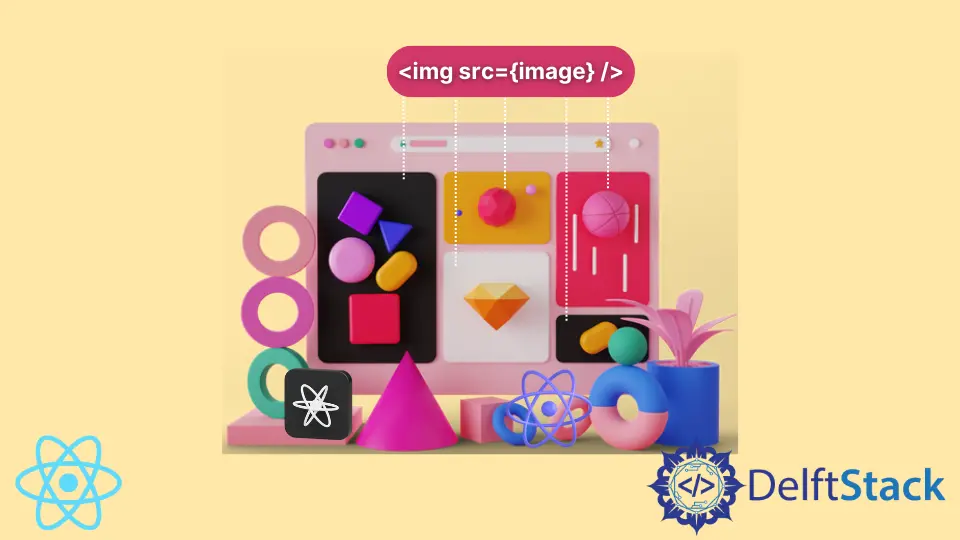
If you’re new to React, you have probably noticed that React uses HTML-like syntax called JSX. This syntax provides a simple way to write React applications. When it comes to including images in the app, things get a little muddy. Unlike HTML, React code goes through many changes before being shown to the users.
When switching from development to production mode, the relative location of images might change. You need a reliable way to import and include images in your React components.
Reference a Local Image in React Using the create-react-app
Package
When developing a React application, you can easily include an image as long as your development environment includes webpack
. When using the create-react-app
package, webpack
is automatically included.
The element tag in JSX works the same way as in HTML - it is a self-closing img
tag. Developers can use the src
attribute to specify the path. In this example, the <img/>
element uses hard-coded image URL as a source:
class App extends Component {
render() {
return <img src="https://cdn.pixabay.com/photo/2021/09/02/16/48/cat-6593947_960_720.jpg"/>
}
}
If you want to specify a relative path to image location within the folder, things get a little more complicated. Providing a source for an image is not as simple as setting the src
attribute equal to a relative file path on the computer. First of all, sandboxed browsers can not access your computer’s src
relative file path. You especially can’t rely on local pathname once the application is deployed. Instead, developers must use either import
or require()
statements to load the image.
When developing a create-react-app
project, it is a good practice to have an assets folder to store all your images. Usually, this folder is created in the src
folder of your project. Assuming that you follow this convention for storing the images, let’s look at how you’d import and use images as a value for the src
attribute.
Load an Image Using the import
Statement in React
You can also use import
statement to load an image. It looks straightforward and accomplishes the same goal as hard-coded url:
import React, { Component } from "react";
import image from "./assets/cat.jpg";
export default class testComponent extends Component {
render() {
return (
<div>
<img src={image} />
</div>
);
}
}
As long as you provide a valid path, your create-react-app
should display an image.
In this case, the path works because both testComponent
and assets
folders are located in the src
folder. The ending of the path - cat.jpg
is just a name of the individual file.
import
statement provides an additional benefit of storing the imported asset in a variable. When setting a value to the src
attribute, you can reference the variable name from the import statement.
You can read more about import here.
Load an Image Using the require()
Method in React
React developers use the require()
method to load various types of modules. It can be used for loading images as well.
If you want to use it within JSX, the require()
method must be placed between curly braces. As you may know, the code between curly braces is interpreted as valid JavaScript in JSX.
<img src={require("./assets/cat.jpg").default} />
The require()
method takes one argument, a valid relative path to an image. Also, note that you must access the .default
property of the returned object in some browsers.
Alternatively, you can create an image
variable to store your image, and then reference it in your component. Here’s an example:
const image = require("./assets/cat.jpg").default;
export default class testComponent extends Component {
render() {
return (
<div>
<img src={image} />
</div>
);
}
}
You can name the variable anything you want. It doesn’t have to be called image
.
Remember that if you want to reference a JavaScript variable in React, you must wrap it in curly braces.
There is no difference between the require()
method and the import
statement regarding performance.
How import
and require()
Work in React
Some React developers might find it surprising that webpack
(and other bundlers) handle image loading, not JavaScript or React.
When you load an image in a component, the bundler internally records the association between the component and the image. Afterward, it will source the asset, copy it, give it a unique name and place it in the accessible directory on the server.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn