How to Use React Inline Styles to Set the Background Image
- Using Inline Styles to Set the Background Image in React
- Using Inline Styles to Set the Local Image as the Background in React
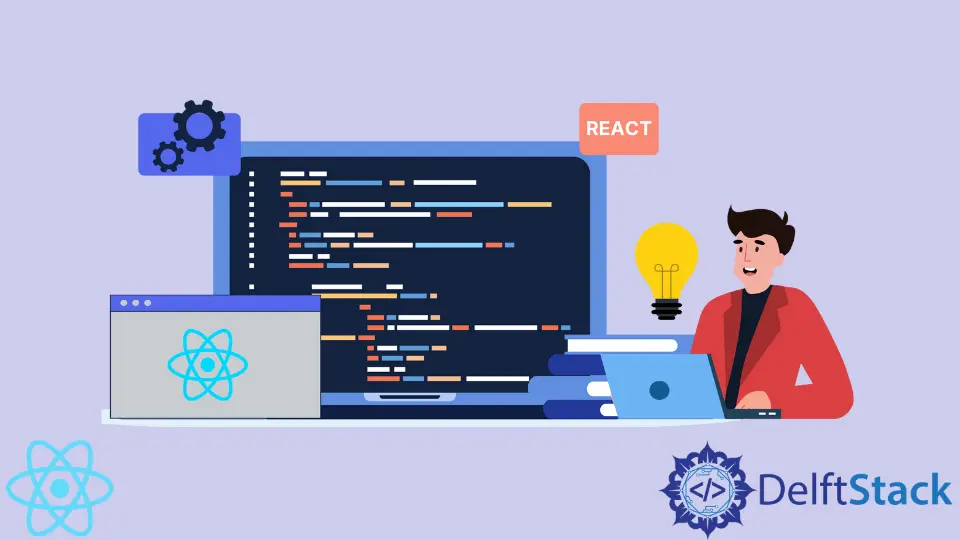
When building complex web applications, developers often need to set a custom background image. The standard approach is to use CSS and HTML.
When developing React applications, you have many options for setting a background image. You can use regular CSS files or use CSS-in-JS
solutions like inline styles in React.
Using Inline Styles to Set the Background Image in React
Inline styles allow you to configure HTML or React components’ styles within JavaScript files. Here’s an example of setting a background image in React class component:
class App extends Component {
render() {
const containerStyle = {
backgroundImage:
"url(https://cdn.pixabay.com/photo/2021/09/02/16/48/cat-6593947_960_720.jpg)",
width: "600px",
height: "600px",
};
return <div style={containerStyle}><h1 style={{color: "black"}}>Hi! Try edit me</h1></div>;
}
}
HTML vs JSX
Like HTML, in JSX, we use the container’s style
attribute to define inline styles. However, unlike HTML, we can’t set its value to a simple text. Instead, we use curly braces to pass a JavaScript variable, containerStyle
. This variable is essentially an object that contains key-value pairs of CSS properties and their corresponding values.
One important difference is that the keys for a style
object must not contain spaces or other non-alphanumeric symbols. In regular CSS, the property would be defined as background-image
, but it becomes backgroundImage
property of a style
object in JavaScript.
One more advantage of using CSS-in-JS
solutions is that your style definitions can include variable references. This is achieved through the use of template literals. Here’s an example:
class App extends Component {
render() {
const backgroundImageURL =
"https://cdn.pixabay.com/photo/2021/09/02/16/48/cat-6593947_960_720.jpg";
const containerStyle = {
backgroundImage:
`url(${backgroundImageURL})`,
width: "600px",
height: "600px",
};
return <div style={containerStyle}><h1 style={{color: "black"}}>Hi! Try edit me</h1></div>;
}
}
In this case, the styles will reflect the changes to the variable.
Using Inline Styles to Set the Local Image as the Background in React
If you’d like to use an image from the local assets
folder, you can use an import
statement or require()
method to load it. However, loading images will only work if your development environment includes webpack.
Whichever way you choose to load your image into the app, you’ll have to specify the relative path:
import image from "./assets/filename.jpg"
This relative path assumes that both the component and asset folder are located in the src
folder.
Once you’ve imported an image, you can reference it by the name of the import (in this case, image
) just like you’d reference a variable. Every image you use in your app must be imported separately, but your background image will only have to be imported once.
You can also create a separate variable and use it to store image value loaded with require()
method:
const image = require("./assets/filename.jpg")
Loading images and giving them a variable name is more readable and gives you the freedom to make changes whenever necessary.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn