How to Import Image in a React Component
- Display Externally Hosted Images Using the Image Link to Import Images in React
-
Use the
import
Statement to Import Images in React -
Use the
require()
Function to Import Images in React
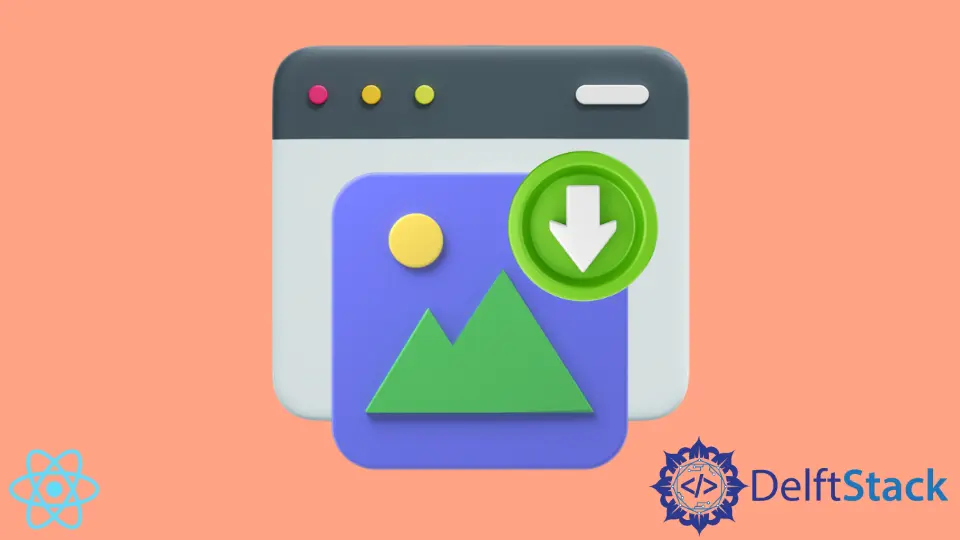
Consumption of visual content motivates billions of internet users to visit their favorite websites every day.
When deciding on a tech stack to build an application, developers should consider technology and visual content compatibility, such as images.
Most modern JavaScript frameworks give you a lot of freedom to import and feature images in your apps. Being one of the most popular frameworks for building dynamic JavaScript applications, React is no exception to that rule.
It allows you to feature images from local as well as external sources. In this article, we want to discuss incorporating images in React applications.
To choose the most optimal approach for importing images in React, you must consider certain factors.
The most important factors are your webpack configuration (and your environment setup in general), project structure, and whether you are hosting the application locally or not.
Display Externally Hosted Images Using the Image Link to Import Images in React
Let’s start with the easiest case, displaying externally hosted images using their link. Let’s look at an example code.
class App extends Component {
render() {
return <img src="https://reactjs.org/logo-og.png" />
}
}
We have a simple app component that returns just one <img>
element. We use the src
attribute to specify the link to the image.
You can check out a live example on playcode.
Featuring locally stored images is a little more difficult.
Use the import
Statement to Import Images in React
The import
statement is the easiest and most readable approach to importing a locally stored image in React.
Let’s see how we could use the image from the previous example when stored locally.
import Logo from "../src/Reactlogo.jpg";
class App extends Component {
render() {
return <img src={Logo} />
}
}
It looks simple enough. Yet, there’s a lot to unpack in this code.
First, we treat the image as a default export and specify the relative path from the file to the image. It’s a convention to store the most important images within the src
folder.
To better understand how to write relative paths, read this article.
In this example, we still use the src
attribute (not to be confused with the folder) of the <img>
element. However, unlike last time, the value for the src
attribute isn’t a string; it’s a Logo
variable.
For this reason, we must use curly braces to read its value. In JSX, we use curly braces to read the value of JavaScript variables and, in general, to treat an expression as a valid JavaScript code.
You can import as many images as you’d like. However, you must provide them with unique names in the import
statement.
Use the require()
Function to Import Images in React
The require()
function does the same thing as the import
statement; it allows you to include external modules from outside files.
Let’s look at how to use require()
to feature the same image from previous examples.
let Logo = require("../src/Reactlogo.jpg")
class App extends Component {
render() {
return <img src={Logo} />
}
}
The syntax is a little different, but as we can see, the require()
function works the same way as the import
statement. The function takes one argument, a relative path of the image.
You can also use the require()
function as an inline value, unlike the import statement. Let’s look at an example.
class App extends Component {
render() {
return <img src={require("../src/Reactlogo.jpg")} />
}
}
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn