How to Combine Two Data Frames in R
-
How to Combine Two Data Frames in R Using the
rbind()
Function -
How to Combine Two Data Frames in R Using the
dplyr
Package -
How to Combine Two Data Frames in R Using the
plyr
Package -
How to Combine Two Data Frames in R Using the
merge()
Function - Conclusion
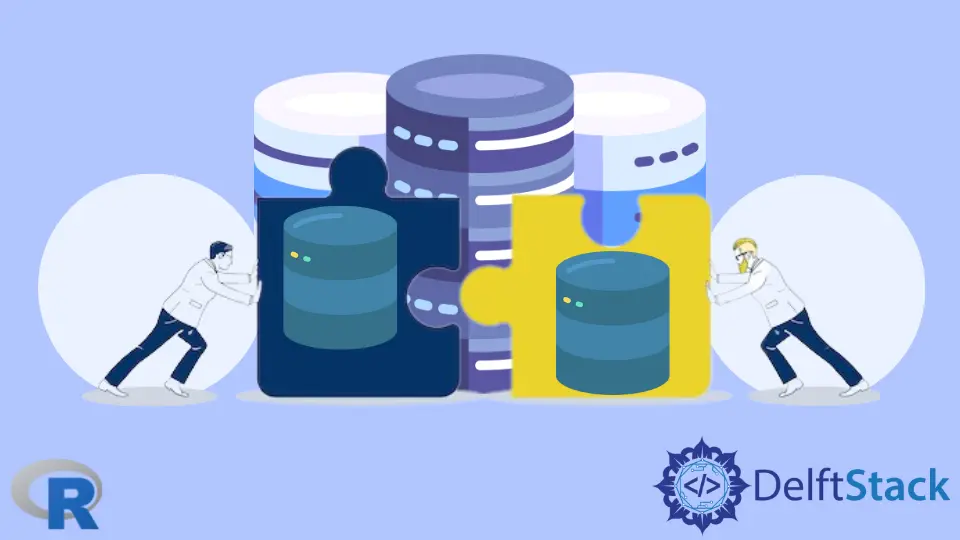
In data analysis and manipulation in R, the need to merge or combine two separate data frames arises frequently. Whether consolidating information from multiple sources or conducting relational operations, learning how to merge data frames is crucial for efficient data handling.
In this article, we delve into various techniques and methods available in R to seamlessly merge two data frames. From basic functions like rbind()
to more advanced tools like those offered by packages such as dplyr
, plyr
, and the versatile merge()
function, we’ll explore each approach, providing insights into their syntax, functionality, and practical applications.
How to Combine Two Data Frames in R Using the rbind()
Function
The rbind()
function is a straightforward tool for combining or merging two data frames by stacking the rows of two or more data frames on top of each other.
The syntax of the rbind()
function is straightforward:
result <- rbind(dataframe1, dataframe2, ...)
Here, dataframe1
, dataframe2
, etc., are the data frames you want to combine. The function takes one or more data frames as arguments and returns a new data frame with the rows stacked on top of each other.
The rbind()
function essentially stacks the rows of the specified data frames one after another to create a new data frame. It aligns the columns based on their names, so the data frames must have the same column names and data types.
If the column names are not identical across the data frames, the function will throw an error.
Now, let’s delve into a practical example to illustrate how to use the rbind()
function in R.
Suppose we have two data frames, x
and y
, with some overlapping columns. We aim to combine these data frames into a single one using the rbind()
function.
x <- data.frame(
a = c(218, 415, 339),
b = c(25, 19, 43),
c = c(950, 872, 645)
)
y <- data.frame(
a = c(309, 115),
b = NA, c = c(799, 814)
)
z <- rbind(x, y)
print("Combined dataframe:")
print(z)
In this example, we first define two data frames, x
and y
, with differing numbers of rows and some overlapping columns (a
and c
). We then use the rbind()
function to combine these data frames into a new data frame, z
.
The rbind()
function seamlessly stacks the rows of x
and y
, aligning them based on their column names. Since both x
and y
have columns a
and c
, the function successfully combines them.
The resulting data frame z
contains all the rows from both x
and y
.
Output:
As shown in the output, the combined data frame z
consists of all the rows from x
followed by all the rows from y
, maintaining the structure and column names of the original data frames.
How to Combine Two Data Frames in R Using the dplyr
Package
In addition to base R functions like rbind()
, the dplyr
package provides a powerful set of functions for data manipulation, including merging or combining data frames.
The dplyr
package offers several functions for merging data frames, including bind_rows()
for row-wise merging. The syntax is as follows:
result <- dplyr::bind_rows(dataframe1, dataframe2, ...)
Here, dataframe1
, dataframe2
, etc., are the data frames you want to combine. The dplyr::bind_rows()
function takes one or more data frames as arguments and returns a new data frame with the rows stacked on top of each other.
The dplyr::bind_rows()
function behaves similarly to rbind()
, stacking the rows of the specified data frames one after another to create a new data frame. It aligns the columns based on their names, ensuring that the columns with the same names are merged correctly.
Like rbind()
, the data frames must have identical column names and data types for successful merging.
Now, let’s proceed with a practical example to demonstrate how to use the dplyr
package for merging data frames in R.
Suppose we have two data frames, x
and y
, with some overlapping columns. We aim to combine these data frames into a single one using the dplyr
package’s bind_rows()
function.
library(dplyr)
x <- data.frame(
a = c(218, 415, 339),
b = c(25, 19, 43),
c = c(950, 872, 645)
)
y <- data.frame(
a = c(309, 115),
c = c(799, 814)
)
z <- dplyr::bind_rows(x, y)
print("Combined dataframe:")
print(z)
In this example, we begin by loading the dplyr
package using the library()
function. Next, we define two data frames, x
and y
, with differing numbers of rows and some overlapping columns (a
and c
).
We then utilize the dplyr::bind_rows()
function to combine these data frames into a new data frame, z
.
The dplyr::bind_rows()
function seamlessly stacks the rows of x
and y
, aligning them based on their column names. Since both x
and y
have columns a
and c
, the function successfully combines them.
The resulting data frame z
contains all the rows from both x
and y
.
Output:
As illustrated in the output, the combined data frame z
consists of all the rows from x
followed by all the rows from y
, maintaining the structure and column names of the original data frames.
How to Combine Two Data Frames in R Using the plyr
Package
In addition to the base R functions and the dplyr
package, the plyr
package also offers functionality for merging or combining data frames in R. This package is particularly known for its consistent and intuitive syntax, making it popular among R users for data manipulation tasks.
The plyr
package provides the join()
function, which can be used to merge two data frames based on common variables. The syntax is as follows:
result <- join(dataframe1, dataframe2, by = "common_column", type = "join_type")
Where:
x
andy
: The data frames to be merged.by
: The common column(s) based on which the merge should be performed.type
: The type of join to be performed (e.g.,inner
,left
,right
,full
,semi
,anti
).
The plyr::join()
function performs an SQL-style join operation between the two data frames based on the specified common column(s).
It combines rows from both data frames where the common column(s) have matching values. The type of join determines how unmatched rows are handled.
Before we begin, ensure that the plyr
package is installed. If not, you can install it from CRAN using the following command:
install.packages("plyr")
Now, let’s proceed with a practical example to demonstrate how to use the plyr
package for merging data frames in R.
library(plyr)
df1 <- data.frame(
ID = c(1, 2, 3),
Name = c("John", "Ray", "Dan")
)
df2 <- data.frame(
ID = c(2, 3, 4),
Age = c(25, 30, 35)
)
inner_merged <- join(df1, df2, by = "ID", type = "inner")
print("Combined dataframe:")
print(inner_merged)
In this example, we first load the plyr
package using the library()
function. Then, we create two sample data frames, df1
and df2
, representing individuals’ information with some overlapping columns.
We aim to merge these data frames based on the common column ID
.
We then use the plyr::join()
function to perform an inner join between df1
and df2
, specifying "ID"
as the common column to merge on and "inner"
as the type of join. This ensures that only rows with matching ID
values in both data frames are retained in the merged result.
Finally, we print the merged data frame inner_merged
to view the result of the inner join operation.
Output:
As shown in the output, the inner join operation using the plyr
package combines rows from df1
and df2
where the ID
values match, resulting in a merged data frame containing columns from both data frames.
How to Combine Two Data Frames in R Using the merge()
Function
In addition to the functions and packages discussed above, the merge()
function is also a powerful tool for combining or merging two data frames based on common columns.
The basic syntax for merging data frames using the merge()
function is as follows:
merge(x, y, by = "common_column", all.x = FALSE, all.y = FALSE, ...)
Where:
x
andy
are the data frames or data tables to be merged.by
specifies the common column(s) based on which the merge should be performed.all.x
andall.y
are logical values indicating whether to include all observations fromx
ory
, respectively.- Additional arguments (
...
) can be used to specify additional options such as the type of join (all
,all.x
,all.y
,intersect
), sorting, or other parameters.
The merge()
function performs an SQL-style join operation between the two data frames based on the specified common column(s). It combines rows from both data frames where the common column(s) have matching values.
Now, let’s proceed with a practical example to demonstrate how to use the merge()
function for merging data frames in R.
df1 <- data.frame(
ID = c(1, 2, 3),
Name = c("John", "Ray", "Dan")
)
df2 <- data.frame(
ID = c(2, 3, 4),
Age = c(25, 30, 35)
)
inner_merged <- merge(df1, df2, by = "ID")
print("Combined dataframe:")
print(inner_merged)
In this example, we create two sample data frames, df1
and df2
, representing individuals’ information with some overlapping columns. We aim to merge these data frames based on the common column ID
.
We then use the merge()
function to perform an inner join between df1
and df2
, specifying "ID"
as the common column to merge on. By default, merge()
performs an inner join, so only rows with matching ID
values in both data frames are retained in the merged result.
Finally, we print the merged data frame inner_merged
to view the result of the inner join operation.
Output:
As shown in the output, the inner join operation using the merge()
function combines rows from df1
and df2
where the ID
values match, resulting in a merged data frame containing columns from both data frames.
Conclusion
Combining or merging two data frames in R is a fundamental operation in data analysis and manipulation. We explored several methods to accomplish this task, including using base R functions like rbind()
, as well as leveraging packages such as dplyr
, plyr
, and merge()
.
The rbind()
function provides a straightforward approach for combining data frames by stacking their rows, while packages like dplyr
and plyr
offer more advanced functionalities for data manipulation, including various types of joins and efficient row-wise operations. Additionally, the merge()
function provides fast and efficient methods for merging data frames, making it suitable for handling large datasets.
Ultimately, the choice of method depends on factors such as the complexity of the data, the desired output, and performance considerations. By understanding the syntax and functionality of these methods, you can effectively merge data frames to streamline your data analysis workflows and derive meaningful insights from your data.