XOR in Python
- What is XOR?
- Using XOR with Integers
- Using XOR with Booleans
- Practical Applications of XOR
- Conclusion
- FAQ
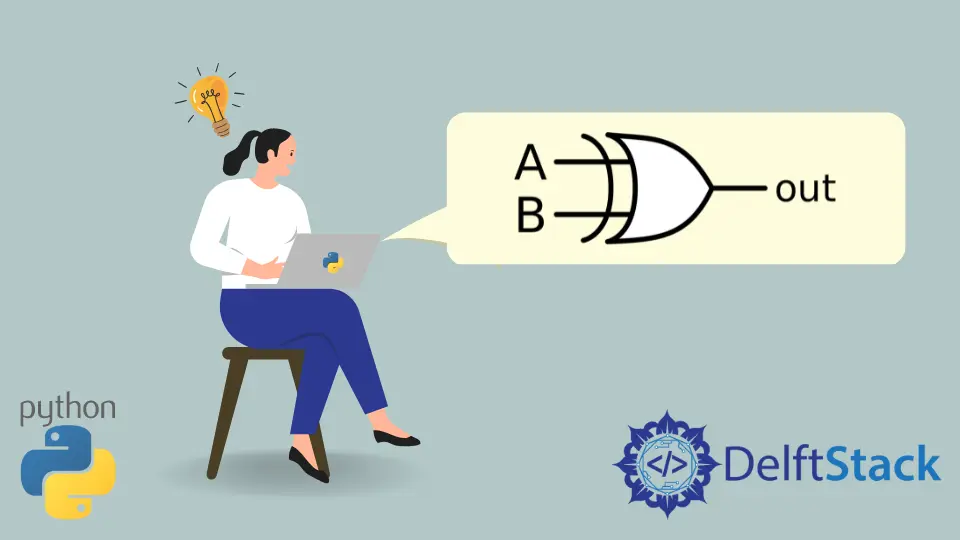
XOR, or exclusive OR, is a fundamental bitwise operation in programming that plays a crucial role in various algorithms and data manipulation tasks. In Python, the XOR operation is performed using the caret symbol (^), and it can be applied to integers and booleans alike.
This tutorial will guide you through the process of obtaining the XOR of two variables in Python, illustrating how this powerful operator can be utilized in practical scenarios. Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to refine your skills, this article will provide you with clear examples and explanations to enhance your understanding of XOR in Python.
What is XOR?
Before diving into the implementation, let’s clarify what XOR is. The XOR operation compares two bits and returns 1 if the bits are different, and 0 if they are the same. This means:
- 0 XOR 0 = 0
- 0 XOR 1 = 1
- 1 XOR 0 = 1
- 1 XOR 1 = 0
This property makes XOR particularly useful in various applications, such as cryptography, error detection, and toggling bits. In Python, you can easily perform XOR operations on integers and boolean values using the ^
operator.
Using XOR with Integers
One of the most common uses of XOR is with integers. Let’s look at how to use the XOR operator in Python to calculate the XOR of two integer variables.
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a ^ b
print(result)
Output:
6
In this example, we first define two integers, a
and b
. The binary representation of 5 is 0101
, and for 3, it is 0011
. When we apply the XOR operation, we compare each corresponding bit:
- 0 XOR 0 = 0
- 1 XOR 0 = 1
- 0 XOR 1 = 1
- 1 XOR 1 = 0
Thus, the resulting binary is 0110
, which equals 6 in decimal form. This simple example demonstrates how easily you can manipulate integer values using the XOR operator in Python.
Using XOR with Booleans
XOR can also be applied to boolean values in Python. This is particularly useful in scenarios where you want to toggle states or evaluate conditions. Let’s see how this works.
x = True
y = False
result = x ^ y
print(result)
Output:
True
In this case, we define two boolean variables, x
and y
. When we apply the XOR operation, we can analyze the possible outcomes:
- True XOR True = False
- True XOR False = True
- False XOR True = True
- False XOR False = False
Since x
is True
and y
is False
, the result of x ^ y
is True
. This example illustrates how XOR can be utilized to evaluate boolean expressions, making it a valuable tool in logical operations.
Practical Applications of XOR
Understanding how to use XOR can open the door to various applications in programming. One practical use case is in swapping two variables without a temporary variable. This is a neat trick that showcases the power of bitwise operations.
x = 10
y = 20
x = x ^ y
y = x ^ y
x = x ^ y
print(x, y)
Output:
20 10
In this example, we start with two integers, x
and y
. By performing the XOR operation three times, we effectively swap their values without needing a temporary variable. Here’s how it works:
x
becomes the XOR ofx
andy
.y
is then updated to the original value ofx
by XORing the newx
withy
.- Finally,
x
is updated to the original value ofy
using the same logic.
This technique is not only efficient, but it also demonstrates the versatility of the XOR operator in Python.
Conclusion
In summary, the XOR operator in Python is a powerful tool for performing bitwise operations on integers and booleans. Whether you’re working with simple integer calculations or more complex logical expressions, understanding how to use XOR can enhance your programming skills and open up new possibilities in your coding projects. From swapping variables to evaluating conditions, the XOR operation is an essential concept worth mastering. As you continue to explore Python, keep in mind the various applications of XOR and how it can simplify your code.
FAQ
-
What does XOR mean in Python?
XOR, or exclusive OR, is a bitwise operator in Python that compares two bits and returns 1 if they are different and 0 if they are the same. -
How do I perform an XOR operation on two integers in Python?
You can use the caret symbol (^) to perform an XOR operation on two integers, like this:result = a ^ b
. -
Can I use XOR with boolean values in Python?
Yes, you can use XOR with boolean values in Python. The operation will returnTrue
if one of the values isTrue
and the other isFalse
. -
What is a practical application of XOR in programming?
One practical application of XOR is swapping two variables without using a temporary variable, which can be done using a series of XOR operations. -
Is XOR commutative in Python?
Yes, the XOR operation is commutative, meaning thata ^ b
is equal tob ^ a
.