How to Write an Array to a Text File in Python
-
How to Write and Save an Array to Text File in Python Using
open()
andclose()
Functions - How to Write and Save an Array to Text File in Python Using Context Manager
-
How to Write and Save an Array to Text File in Python Using NumPy’s
numpy.savetxt()
andnumpy.loadtxt()
- Conclusion
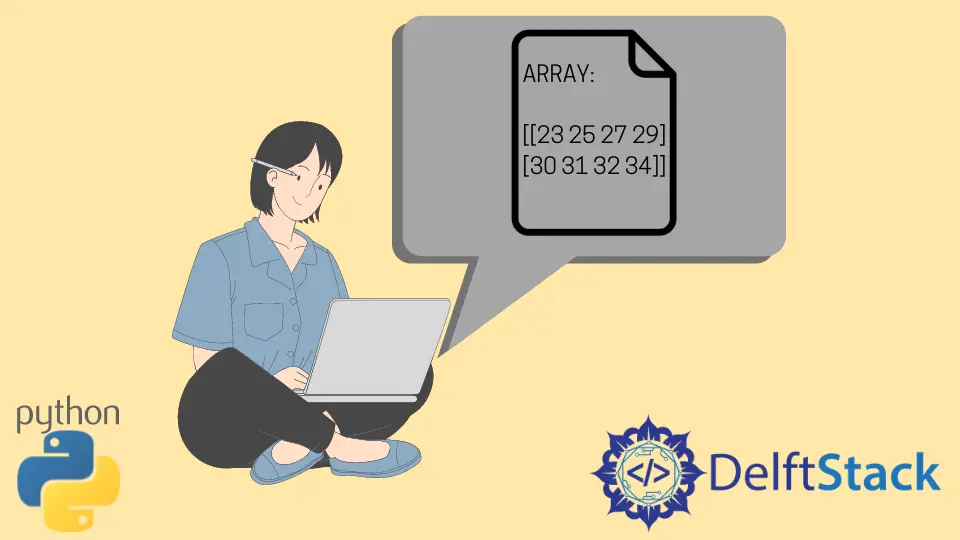
In Python programming, the ability to write arrays into text files is a fundamental skill with widespread applications.
This article aims to provide you with a deep understanding of various methods for performing this essential task.
From the foundational approach utilizing the open()
and close()
functions to more advanced techniques involving context managers and NumPy functions, this guide is your key to learning how to write arrays to text files in Python.
How to Write and Save an Array to Text File in Python Using open()
and close()
Functions
A straightforward approach to writing an array to a text file involves using the open()
and close()
functions in Python.
The open()
function is the gateway to file operations in Python. Its basic syntax is as follows:
open(
file,
mode="r",
buffering=-1,
encoding=None,
errors=None,
newline=None,
closefd=True,
opener=None,
)
Parameters:
file
: The name of the file or a path to the file.mode
(optional): The mode in which the file is opened. The default is'r'
(read mode). Other common modes include'w'
for write,'a'
for append, and'w+'
for both write and read.buffering
(optional): Specifies the buffering policy. Ifbuffering
is set to0
, no buffering occurs. If it’s set to1
, line buffering is enabled. If it’s greater than1
, buffering will have a specific buffer size. If it’s set to-1
(default), the system default buffering will be used.encoding
(optional): The name of the encoding to be used. If omitted, the system’s default encoding is used.errors
(optional): Specifies how encoding and decoding errors are to be handled. If omitted, the default is'strict'
, which raises an error on encoding/decoding errors.newline
(optional): Controls how universal newlines mode works. If omitted (None
), it uses the default behavior. If set to an empty string, it disables universal newlines. If set to'\n'
, it enables universal newlines as a line ending.closefd
(optional): Ifclosefd
isFalse
, the underlying file descriptor will not be closed when the file is closed. The default isTrue
.opener
(optional): An optional custom opener. If provided, it must be a callable that returns an open file descriptor.
In our case, we will use 'w+'
as the mode to open the file for writing and reading. If the file doesn’t exist, it will be created; otherwise, its contents will be overwritten.
Once the file is opened, we can utilize the write()
function to insert data into it. In the context of writing an array, we convert the array to a string using str()
and then write it to the file. Finally, the close()
function is used to close the file, ensuring that the changes are saved.
Code Example:
import numpy as np
# Creating a sample array
sample_list = [23, 22, 24, 25]
new_array = np.array(sample_list)
# Opening a file in write mode
file = open("sample.txt", "w+")
# Converting the array to a string and writing to the file
content = str(new_array)
file.write(content)
# Closing the file
file.close()
# Displaying the contents of the text file
file = open("sample.txt", "r")
content = file.read()
print("Array contents in sample.txt:", content)
# Closing the file after reading
file.close()
In the code above, we start by importing NumPy, a powerful library for numerical operations in Python. We create a sample array named sample_list
and convert it into a NumPy array called new_array
.
We then use the open()
function to open a file named sample.txt
in write mode ('w+'
). This file is created if it doesn’t exist and its existing content is overwritten.
Next, we convert the array to a string using str(new_array)
and write it to the file using the write()
function. The file is then closed with file.close()
.
To verify the contents, we reopen the file in read mode ('r'
), read its content using file.read()
, and print the array contents. Finally, we close the file again after reading it.
Code Output:
In this example, the array [23, 22, 24, 25]
has been successfully written to the sample.txt
file and subsequently read and displayed. This simple and effective method using open()
and close()
functions is useful for basic file writing operations in Python.
Now, let’s explore an example involving a multi-dimensional array. Suppose we have a 2D array:
import numpy as np
# Creating a multi-dimensional array
multi_dim_array = np.array([[23, 22, 24, 25], [13, 14, 15, 19]])
# Opening a file in write mode
file = open("multi_dim_sample.txt", "w+")
# Converting the array to a string and writing to the file
content = str(multi_dim_array)
file.write(content)
# Closing the file
file.close()
# Displaying the contents of the text file
file = open("multi_dim_sample.txt", "r")
content = file.read()
print("Array contents in multi_dim_sample.txt:", content)
# Closing the file after reading
file.close()
In this extended example, we create a 2D array named multi_dim_array
. The subsequent process remains consistent: opening a file in write mode, converting the array to a string, writing to the file, reading the contents, and displaying them.
Code Output:
In this scenario, the multi-dimensional array [[23, 22, 24, 25], [13, 14, 15, 19]]
has been successfully written to the multi_dim_sample.txt
file and displayed. This example illustrates the adaptability of the open()
and close()
functions for handling both 1D and multi-dimensional arrays in Python.
How to Write and Save an Array to Text File in Python Using Context Manager
Alternatively, a context manager can be employed for writing an array to a text file. Unlike open()
and close()
, the context manager automatically manages file opening and closing.
It is employed with the with
statement and ensures that setup and teardown actions are performed around a block of code.
For file handling, the open()
function is often used as a context manager, eliminating the need to explicitly close the file. The basic syntax is as follows:
with open(file, mode, encoding) as f:
# Code to work with the file goes here
In this context, file
is the name of the file, mode
is the opening mode ('r'
for reading, 'w'
for writing, etc.), and encoding
is the character encoding. The file will automatically close when the block of code is exited, ensuring resource cleanup.
In Python, using the context manager is considered a better practice when managing resources instead of using the open()
and close()
functions.
Code Example:
Let’s consider an example where we create a simple array and write it to a text file using a context manager:
import numpy as np
# Creating a sample array
sample_list = [23, 25, 27, 29, 30]
new_array = np.array(sample_list)
print(new_array)
# Writing the array to a text file using a context manager
with open("sample.txt", "w+") as f:
f.write(str(new_array))
In this code snippet, we start by importing NumPy and creating a sample array named sample_list
, which is converted into a NumPy array (new_array
). We then employ the open()
function as a context manager with the with
statement to open the file sample.txt
in write mode ('w+'
).
Inside the indented block, we use the write()
function to insert the string representation of the array into the file. Once the block is exited, the context manager automatically closes the file.
Code Output:
In this example, the array [23, 25, 27, 29, 30]
is written to the sample.txt
file using the context manager. The beauty of this approach lies in its simplicity and efficiency; the file is automatically closed, and resource cleanup is taken care of without the need for explicit close()
statements.
Let’s expand our understanding by considering a scenario involving a multi-dimensional array:
import numpy as np
# Creating a multi-dimensional array
multi_dim_list = [[23, 25, 27, 29], [30, 31, 32, 34]]
multi_dim_array = np.array(multi_dim_list)
print(multi_dim_array)
# Writing the multi-dimensional array to a text file using a context manager
with open("multi_dim_sample.txt", "w+") as f:
f.write(str(multi_dim_array))
In this example, we initiate by generating a multi-dimensional array named multi_dim_list
, which is then converted into a NumPy array (multi_dim_array
). The open()
function is utilized as a context manager within the with
statement to open the file multi_dim_sample.txt
in write mode ('w+'
).
Within the indented block, we use the write()
function to insert the string representation of the multi-dimensional array into the file. Upon exiting the block, the context manager seamlessly closes the file.
Code Output:
In this expanded example, the multi-dimensional array [[23, 25, 27, 29], [30, 31, 32, 34]]
is effortlessly written to the multi_dim_sample.txt
file using the context manager. Context managers in Python not only enhance code aesthetics but also contribute to more robust and maintainable file-handling practices.
How to Write and Save an Array to Text File in Python Using NumPy’s numpy.savetxt()
and numpy.loadtxt()
Python, equipped with the powerful NumPy library, also provides specialized functions—numpy.savetxt()
and numpy.loadtxt()
—that simplify array-to-text file operations.
NumPy’s numpy.savetxt()
function is designed to save arrays to text files. The basic syntax is as follows:
numpy.savetxt(
fname,
X,
fmt="%.18e",
delimiter=" ",
newline="\n",
header="",
footer="",
comments="# ",
encoding=None,
)
Parameters:
fname
: The name of the file or a file-like object.X
: The data to be saved. It can be an array or a sequence of arrays.fmt
: The format for writing data.delimiter
: The string separating values.newline
: String or character separating lines.header
: String to be written at the beginning of the file.footer
: String to be written at the end of the file.comments
: String to be prepended toheader
andfooter
lines.encoding
: Character encoding used to encode the output file.
On the other hand, the numpy.loadtxt()
function is used for loading data from a text file. The basic syntax is as follows:
numpy.loadtxt(
fname,
dtype=float,
comments="#",
delimiter=None,
converters=None,
skiprows=0,
usecols=None,
unpack=False,
ndmin=0,
encoding="bytes",
max_rows=None,
)
Parameters:
fname
: The name of the file or a file-like object.dtype
: Data type of the resulting array.comments
: The character or characters used to indicate the start of a comment.delimiter
: The string used to separate values.converters
: A dictionary mapping column number to a function that will convert that column to a float.skiprows
: Number of rows to skip at the beginning of the file.usecols
: Which columns to read, with 0 being the first.unpack
: IfTrue
, the returned array is transposed.ndmin
: The array returned will have at least this number of dimensions.encoding
: Character encoding used to decode the input file.max_rows
: Maximum number of rows to read.
Let’s illustrate the usage of these functions with a practical example. We’ll create a simple array, save it to a text file using numpy.savetxt()
, and then load it back using numpy.loadtxt()
:
import numpy as np
# Creating a sample array
sample_list = [23, 24, 25, 26, 28]
new_array = np.array(sample_list)
print("Original Array:\n", new_array)
# Saving the array to a text file
np.savetxt("numpy_sample.txt", new_array, delimiter=", ", fmt="%d")
# Loading the array from the text file
loaded_array = np.loadtxt("numpy_sample.txt", dtype=int)
print("\nLoaded Array:\n", loaded_array)
In this code snippet, we start by importing NumPy and creating a sample array named sample_list
, which is converted into a NumPy array (new_array
).
The original array is then displayed. We proceed to use numpy.savetxt()
to save the array to a text file named numpy_sample.txt
. The delimiter
parameter is set to ,
to separate values by a comma and space, and fmt
is set to %d
to indicate integer formatting.
Subsequently, we use numpy.loadtxt()
to load the array back from the text file. The resulting loaded array is then displayed.
Code Output:
In this example, the original array [23, 24, 25, 26, 28]
is successfully saved to the numpy_sample.txt
file and later loaded back into the loaded_array
.
Let’s consider another example involving a 2D array. We’ll create a multi-dimensional array, save it to a text file using numpy.savetxt()
, and then load it back using numpy.loadtxt()
:
import numpy as np
# Creating a multi-dimensional array
multi_dim_list = [[23, 24, 25, 26, 28], [34, 45, 46, 49, 48]]
multi_dim_array = np.array(multi_dim_list)
print("Original Multi-Dimensional Array:\n", multi_dim_array)
# Saving the array to a text file
np.savetxt("numpy_multi_dim_sample.txt", multi_dim_array, fmt="%d")
# Loading the array from the text file
loaded_multi_dim_array = np.loadtxt("numpy_multi_dim_sample.txt", dtype=int)
print("\nLoaded Multi-Dimensional Array:\n", loaded_multi_dim_array)
In this example, we begin by creating a 2D array named multi_dim_list
, which is then converted into a NumPy array (multi_dim_array
). The original multi-dimensional array is displayed.
We proceed to use numpy.savetxt()
to save the array to a text file named numpy_multi_dim_sample.txt
. The fmt
parameter is set to %d
for integer formatting.
Subsequently, we use numpy.loadtxt()
to load the array back from the text file. The resulting loaded multi-dimensional array is then displayed.
Code Output:
In this extended example, the original 2D array [[23, 24, 25, 26, 28], [34, 45, 46, 49, 48]]
is successfully saved to the numpy_multi_dim_sample.txt
file and later loaded back into the loaded_multi_dim_array
. The capabilities of NumPy’s numpy.savetxt()
and numpy.loadtxt()
functions shine through, offering a robust solution for handling multi-dimensional arrays in text files with ease.
Conclusion
Python provides several methods for efficiently saving arrays to text files, catering to different preferences and use cases. We explored three distinct approaches: the open()
and close()
functions, a context manager for automatic file handling, and NumPy with numpy.savetxt()
and numpy.loadtxt()
functions.
The first method, employing the open()
and close()
functions, provides a straightforward and fundamental way to write arrays to text files. This approach is suitable for basic scenarios and offers control over the file’s opening mode and encoding.
The context manager method, using the with
statement with the open()
function, enhances code readability and eliminates the need for explicit closing statements. It is a recommended practice for resource management, ensuring files are closed automatically after use.
Finally, NumPy’s numpy.savetxt()
and numpy.loadtxt()
functions offer specialized functionality for handling numerical arrays. These functions provide flexibility in formatting and are particularly useful when dealing with multi-dimensional arrays.
The choice of method will depend on the specific requirements of the task at hand. For simple and quick operations, the open()
and close()
functions may suffice.
The context manager method is recommended for improved code structure and resource management. When dealing with numerical arrays, especially in scientific computing, NumPy’s functions provide a powerful and convenient solution.
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn