在 Python 中將陣列寫入文字檔案
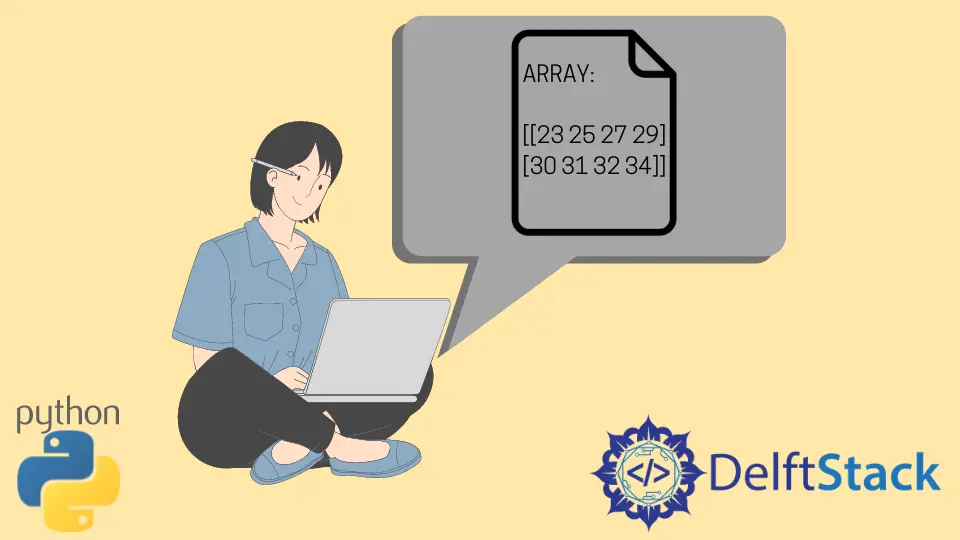
讀取和寫入檔案是構建許多使用者使用的程式的一個重要方面。Python 提供了一系列可用於資源處理的方法。這些方法可能會略有不同,具體取決於檔案的格式和正在執行的操作。
除了通過單擊按鈕建立檔案的傳統方式外,我們還可以使用內建函式(例如 open()
函式)建立檔案。請注意,open()
函式只會在檔案不存在時建立檔案;否則,它本質上用於開啟檔案。
在 Python 中使用 open()
和 close()
函式將陣列寫入文字檔案
由於 open()
函式不單獨使用,將它與其他函式結合使用,我們可以執行更多的檔案操作,例如寫入和修改或覆蓋檔案。這些函式包括 write()
和 close()
職能。使用這些函式,我們將使用 NumPy 建立一個陣列,並使用 write 函式將其寫入文字檔案,如下面的程式所示。
import numpy as np
sample_list = [23, 22, 24, 25]
new_array = np.array(sample_list)
# Displaying the array
file = open("sample.txt", "w+")
# Saving the array in a text file
content = str(new_array)
file.write(content)
file.close()
# Displaying the contents of the text file
file = open("sample.txt", "r")
content = file.read()
print("Array contents in sample.txt: ", content)
file.close()
輸出:
Array contents in text_sample.txt: [23 22 24 25]
在上面的示例中,我們在寫入模式下使用 open 函式建立了一個名為 sample.txt
的文字檔案。然後,我們繼續將陣列轉換為字串格式,然後使用 write
函式將其內容寫入文字檔案。使用 open()
函式,我們以閱讀模式開啟文字檔案的內容。文字檔案內容顯示在終端中,也可以通過物理開啟文字檔案來檢視。
同樣,我們也可以建立一個多維陣列,儲存到一個文字檔案中,如下圖。
import numpy as np
sample_list = [[23, 22, 24, 25], [13, 14, 15, 19]]
new_array = np.array(sample_list)
# Displaying the array
file = open("sample.txt", "w+")
# Saving the array in a text file
content = str(new_array)
file.write(content)
file.close()
# Displaying the contents of the text file
file = open("sample.txt", "r")
content = file.read()
print("Array contents in sample.txt: ", content)
file.close()
輸出:
Array contents in sample.txt: [[23 22 24 25]
[13 14 15 19]]
在 Python 中使用內容管理器將陣列寫入文字檔案
或者,我們可以使用上下文管理器將陣列寫入文字檔案。與 open()
函式不同,我們必須在使用 close()
函式開啟檔案後關閉檔案,內容管理器允許我們在需要時精確地開啟和關閉檔案。在 Python 中,在管理資源而不是使用 open()
和 close()
函式時,使用上下文管理器被認為是一種更好的做法。上下文管理器可以使用如下所示的 with
關鍵字來實現。
import numpy as np
new_list = [23, 25, 27, 29, 30]
new_array = np.array(new_list)
print(new_array)
with open("sample.txt", "w+") as f:
data = f.read()
f.write(str(new_array))
輸出:
[23 25 27 29 30]
在上面的示例中,上下文管理器開啟文字檔案 sample.txt
,由於該檔案不存在,上下文管理器建立它。在上下文管理器的範圍內,我們在將陣列轉換為字串時將其寫入文字檔案。一旦我們選擇退出縮排,上下文管理器會自動關閉檔案。同樣,如下所示,我們也可以使用上下文管理器將多維陣列寫入文字檔案。
import numpy as np
new_list = [[23, 25, 27, 29], [30, 31, 32, 34]]
new_array = np.array(new_list)
print(new_array)
with open("sample.txt", "w+") as f:
data = f.read()
f.write(str(new_array))
輸出:
[[23 25 27 29]
[30 31 32 34]]
NumPy 是一個科學庫,它提供了一系列用於處理陣列的函式。我們可以使用 numpy.savetxt()
函式將陣列儲存到文字檔案中。該函式接受幾個引數,包括檔名、格式、編碼格式、分隔列的分隔符、頁首、頁尾和檔案隨附的註釋。
除此之外,NumPy 函式還提供 numpy.loadtxt()
函式來載入文字檔案。
我們可以將陣列儲存到文字檔案並使用這兩個函式載入它,如下面的程式碼所示。
import numpy as np
new_list = [23, 24, 25, 26, 28]
new_array = np.array(new_list)
print(new_array)
np.savetxt("sample.txt", new_array, delimiter=", ")
content = np.loadtxt("sample.txt")
print(content)
輸出:
[23 24 25 26 28]
如下圖,我們也可以使用這些函式將多維陣列儲存到文字檔案中。
import numpy as np
new_list = [[23, 24, 25, 26, 28], [34, 45, 46, 49, 48]]
new_array = np.array(new_list)
print(new_array)
np.savetxt("sample7.txt", new_array, delimiter=", ")
content = np.loadtxt("sample7.txt")
print(content)
輸出:
[[23 24 25 26 28]
[34 45 46 49 48]]
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn