Web Element Methods in Selenium Python
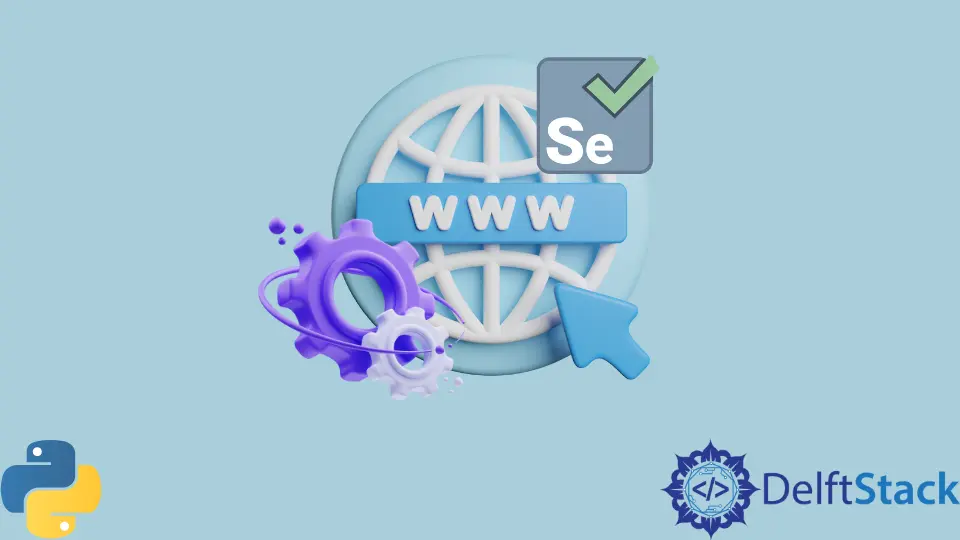
Selenium is a powerful automation and testing tool for web browsers. We write scripts using Selenium, which controls the web browser and performs specific actions.
Install Selenium and Chrome WebDriver
To install Selenium, we use the following command.
#Python 3.x
pip install selenium
ChromeDriver is another executable that Selenium WebDriver uses to interact with Chrome. If we want to automate tasks on the Chrome web browser, we also need to install ChromeDriver.
According to the version of the Chrome browser, we need to select a compatible driver for it. Following are the steps to install and configure the Chrome driver:
- Click on this link. Download Chrome driver according to the version of your Chrome browser and the type of operating system.
- If you want to find the version of your Chrome browser, click on the three dots on the top right corner of Chrome, click on Help, and select About Google Chrome. You can see the Chrome version in the about section.
- Extract the zip file and run the Chrome driver.
Web Element Methods in Selenium Python
A web element is an HTML element on the website. It can be a text field, button, scrollbar, etc.
It’s something we want to interact with, like performing a click, filling a text field, etc. Once we get a web element in Python, we can apply many methods to that element provided by Selenium.
For example, we have the following web element in HTML.
<input type="text" name="email" id="email_id" />
We use the following code snippet to locate a web element using its name
.
element_tf = driver.find_element_by_name("email")
Or, if we locate it by its id
, we will use this method.
element_tf = driver.find_element_by_id("email_id")
After we get the element, we can apply many methods to it. Some of the widely used methods and their description are as follows.
Element Method | Description |
---|---|
click() |
Clicks any element. |
clear() |
Clears any text from a text field. |
send_keys() |
Enters the text in the text fields. |
text |
Gets the text of the current element. |
screenshot() |
Takes a screenshot of the current element and saves it as a PNG file. |
submit() |
Submit the form’s data after we finish entering the details. |
location |
Gets the location of the current element. |
size |
Returns the size of the element. |
tag_name |
Returns the name of the tag of the current element. |
is_selected() |
Returns a Boolean value indicating whether the element is selected or not. |
is_displayed() |
Returns a Boolean value indicating whether the element is visible to the user on-screen or not |
get_property() |
Returns the element’s property like anchor text’s text_length property. |
get_attribute() |
Returns the element’s attribute, such as the href attribute of the anchor tag. |
Code Demonstrating Web Element Methods
We have used some of the above methods in the following code. We get the email
and password
text fields and the login button by their names using the find_view_by_name()
method.
We entered the dummy email and password using the send_keys()
method and have cleared the password
field using the clear()
method. We have extracted the login button’s text, location, and tag name using the methods text
, location
, and tag_name
.
We have checked whether the login button is selected and displayed using the is_selected()
and is_displayed()
methods. Finally, we have clicked the login button using the click()
method.
# Python 3.x
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
driver = webdriver.Chrome(r"E:\download\chromedriver.exe")
driver.maximize_window()
driver.get("https://discpersonalitytesting.com/login-here/")
email = "myemail@email.com"
password = "mypassword1@23"
email_textfield = driver.find_element_by_name("email")
password_textfield = driver.find_element_by_name("password")
login_button = driver.find_element_by_name("LoginDAPLoginForm")
email_textfield.send_keys(email)
time.sleep(2)
password_textfield.send_keys(password)
time.sleep(3)
password_textfield.clear()
password_textfield.send_keys(password)
time.sleep(2)
print(login_button.text)
print(login_button.location)
print(login_button.is_selected())
print(login_button.is_displayed())
print(login_button.tag_name)
login_button.click()
Output:
Login
{'x': 251, 'y': 499}
False
True
button
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python