How to Fix ValueError: Too Many Values to Unpack
-
What Is
ValueError
in Python -
Fix the
ValueError: too many values to unpack
in Python -
Handle Exception
ValueError: too many values to unpack
WithTry-Catch
in Python
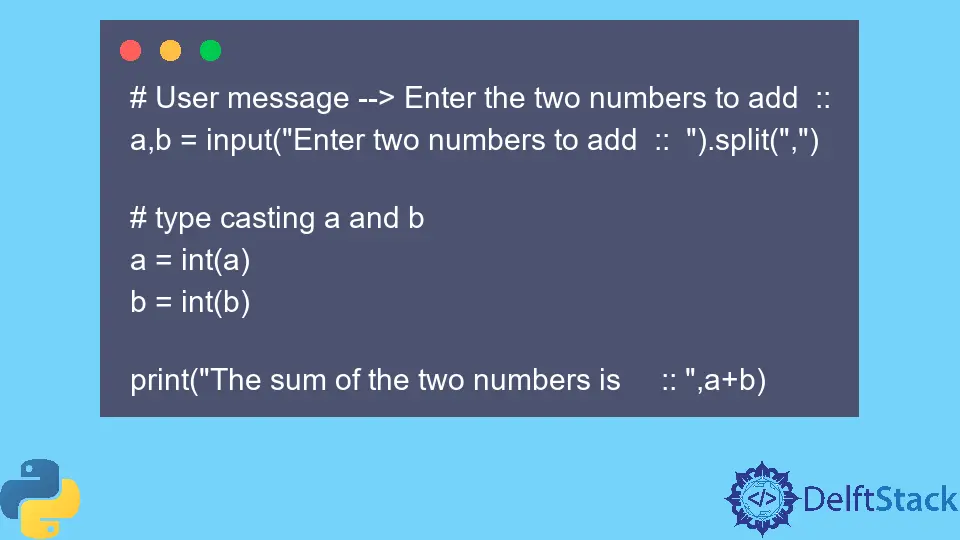
The ValueError: too many values to unpack
sometimes occurs when the variables on the left of the assignment operator =
are not equal to the values on the right side of the assignment operator =
.
It commonly happens when you try to take more than one input from the user in a single input
statement or assign unequal variables to some values.
What Is ValueError
in Python
A ValueError
is a common exception in Python that occurs when your number of values does not meet the number of variables either taking input, direct assignment or through an array. To understand the ValueError
, let’s take an example.
Code example:
# take two string values as input separated by a comma
x, y = input("Enter x and y: ").split(",")
Output:
Eneter x and y: 3,2,1
ValueError: too many values to unpack (expected 2)
As you can see in the above code, we are taking two inputs, x
and y
; the input
statement expects two values separated by the comma ,
.
But in this case, we have provided three values as input to demonstrate the topic, which has thrown the ValueError: too many values to unpack
.
Another case of the ValueError: too many values to unpack
could be directly assigning values to variables. Let’s understand it through an example.
a, b = 2, 3, 5 # ValueError: too many values to unpack (expected 2)
a, b, c = 2, 3 # ValueError: not enough values to unpack (expected 3, got 2)
a, b = [3, 2, 1] # ValueError: too many values to unpack (expected 2)
The above are a few other cases that throw the ValueError
.
Fix the ValueError: too many values to unpack
in Python
To avoid the ValueError
, you should provide the exact number of values as expected by either the input
statement, a list
or an array
. The best practices are to use try-catch
blocks and display messages to the users to guide them.
Let’s understand how we can fix the ValueError: too many values to unpack
.
# User message --> Enter the two numbers to add ::
a, b = input("Enter two numbers to add :: ").split(",")
# type casting a and b
a = int(a)
b = int(b)
print("The sum of the two numbers is :: ", a + b)
Output:
Enter two numbers to add :: 22,23
The sum of the two numbers is :: 45
Handle Exception ValueError: too many values to unpack
With Try-Catch
in Python
Almost every other programming language has the try-catch
mechanism for exception handling. Exception handling is a proactive mechanism that controls the errors or exceptions before crashing the program and helps display the nature and cause of the error.
Also, you can display your messages to interpret the exceptions.
Let’s understand it through an example.
try:
# User message --> Enter the two numbers to add ::
x, y = input("Enter two numbers to add :: ").split(",")
# type casting x and y
x = int(x)
y = int(y)
print("The sum of the two numbers is :: ", x + y)
except ValueError as v:
print(
"Oops! Looks like you have enter an invalid number of input"
+ "\nPlease enter two numbers to add"
)
print("ValueError:", v)
print("\nWow! The program is not crashed.")
Output:
Enter two numbers to add :: 1,2,3
Oops! Looks like you have enter an invalid number of input
Please enter two numbers to add
ValueError: too many values to unpack (expected 2)
Wow! The program is not crashed.
As you can see, the above program is failed, and it causes the ValueError
, but note that the program has not yet crashed.
You can see the code in the try
block has caused the ValueError
exception, and instead of crashing it at the line, the try
block has passed the error to the except
block, which interprets the exception and displays a customized message to the user. The customized message helps us interpret the error easily.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python