How to Fix the Unhashable Type numpy.ndarray Error in Python
- Understanding the Unhashable Type Error
- Method 1: Converting ndarray to Tuple
-
Method 2: Using the
tobytes()
Method - Method 3: Using a Frozen Array
- Conclusion
- FAQ
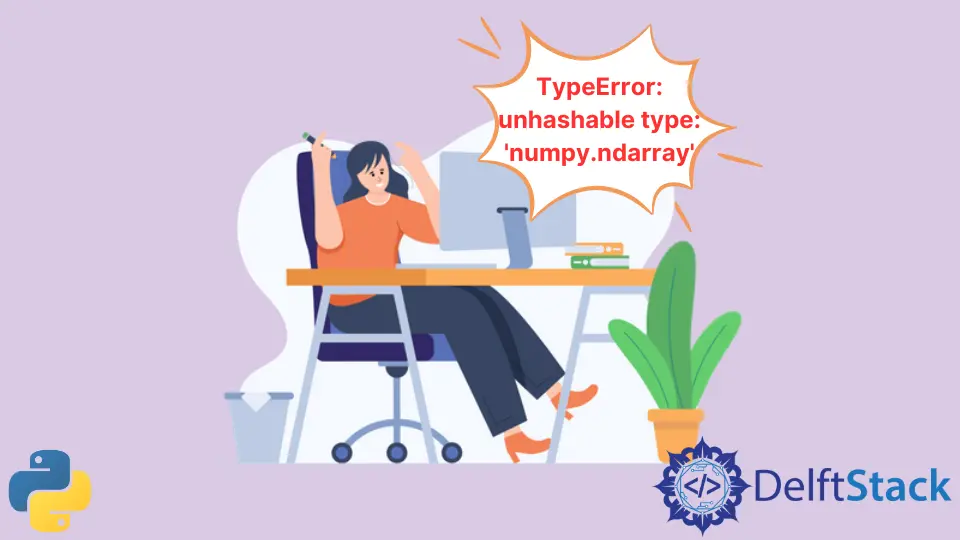
When working with Python, particularly in data science and machine learning, you may encounter the dreaded “unhashable type: numpy.ndarray” error. This error typically arises when you try to use a NumPy array as a key in a dictionary or as an element in a set. Since NumPy arrays are mutable, they cannot be hashed, leading to this common issue.
In this article, we’ll explore effective methods to resolve this error, providing clear explanations and code examples to help you understand the solutions. Whether you’re a seasoned programmer or a beginner, these strategies will enhance your coding experience and boost your problem-solving skills.
Understanding the Unhashable Type Error
Before diving into the solutions, it’s essential to understand what the unhashable type error means. In Python, hashable objects are those that have a fixed hash value throughout their lifetime. This characteristic allows them to be used as keys in dictionaries or as elements in sets. However, mutable objects like lists and NumPy arrays cannot be hashed, resulting in the “unhashable type” error.
For example, if you attempt to add a NumPy array to a set, Python will raise an error because it can’t guarantee the array’s hash value will remain constant. Understanding this concept is crucial for effectively addressing the issue.
Method 1: Converting ndarray to Tuple
One of the simplest ways to fix the unhashable type error is by converting the NumPy array into a tuple. Tuples are immutable and hashable, making them suitable for use as dictionary keys or set elements. Here’s how you can do it:
import numpy as np
array = np.array([1, 2, 3])
my_dict = {}
my_dict[tuple(array)] = "This is a value"
print(my_dict)
Output:
{(1, 2, 3): 'This is a value'}
By converting the NumPy array to a tuple using the tuple()
function, we can now use it as a key in a dictionary. This method is straightforward and effective, allowing you to maintain the array’s data while circumventing the unhashable type error. It’s worth noting that this approach works well for small arrays. However, if you’re dealing with large datasets or multidimensional arrays, consider the implications on performance and memory usage.
Method 2: Using the tobytes()
Method
Another effective method to resolve the unhashable type error is by utilizing the tobytes()
method available in NumPy arrays. This method converts the array into a bytes representation, which is hashable. Here’s how you can implement this solution:
import numpy as np
array = np.array([4, 5, 6])
my_set = set()
my_set.add(array.tobytes())
print(my_set)
Output:
{b'\x04\x00\x00\x00\x05\x00\x00\x00\x06\x00\x00\x00'}
In this example, the tobytes()
method converts the NumPy array into a bytes object. This bytes object can then be added to a set without raising an unhashable type error. This method is particularly useful when working with large datasets, as it efficiently handles the data’s memory representation. However, keep in mind that while this method resolves the error, it may not allow you to easily retrieve the original array from the bytes representation without additional processing.
Method 3: Using a Frozen Array
If you need to maintain the structure of the NumPy array while ensuring it is hashable, consider using a frozen array. A frozen array is essentially a NumPy array that cannot be modified after its creation, making it hashable. You can create a frozen array using the following approach:
import numpy as np
array = np.array([7, 8, 9])
frozen_array = np.array(array, copy=False)
my_dict = {}
my_dict[tuple(frozen_array)] = "This is another value"
print(my_dict)
Output:
{(7, 8, 9): 'This is another value'}
In this case, we create a frozen array by ensuring that the original array is not copied, thus preserving its immutability. By converting it to a tuple, we can use it as a key in the dictionary without encountering the unhashable type error. This method is particularly useful when you want to maintain the integrity of the array while still leveraging its data in hashable contexts.
Conclusion
Encountering the unhashable type numpy.ndarray error in Python can be frustrating, but understanding the underlying principles and applying the right methods can help you overcome this challenge. In this article, we’ve explored several effective solutions, including converting NumPy arrays to tuples, using the tobytes()
method, and creating frozen arrays. By implementing these strategies, you can ensure your code runs smoothly and efficiently, allowing you to focus on what truly matters—analyzing data and building robust applications. Remember, every error is an opportunity to learn and grow as a programmer.
FAQ
- What does the unhashable type error mean in Python?
The unhashable type error indicates that you’re trying to use a mutable object, like a NumPy array, as a key in a dictionary or an element in a set, which is not allowed.
-
How can I convert a NumPy array to a tuple?
You can convert a NumPy array to a tuple by using thetuple()
function, like this:tuple(array)
. -
What is the purpose of the
tobytes()
method in NumPy?
Thetobytes()
method converts a NumPy array into a bytes representation, allowing it to be used in hashable contexts without raising an error. -
Can I retrieve the original array from its bytes representation?
Yes, you can retrieve the original array from its bytes representation, but it requires additional processing to reshape it back into its original form. -
Is using a frozen array the best solution for all scenarios?
Using a frozen array is effective for ensuring immutability, but it may not be necessary for all scenarios. Choose the method that best fits your specific use case.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python