How to Fix TypeError: List Indices Must Be Integers, Not STR in Python
-
Understand the Root Cause of the
TypeError: list indices must be integers or slices, not str
in Python -
Replicate the
TypeError: list indices must be integers or slices, not str
in Python - Solve the Error in Python
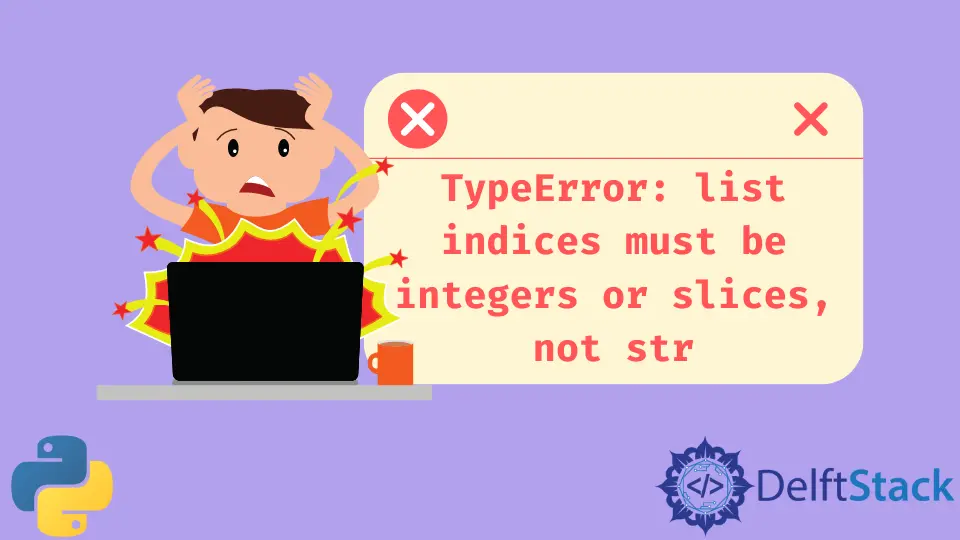
In this tutorial, we aim to explore how to get rid of the TypeError: list indices must be integers or slices, not str
.
This article tackles the following topics.
- Understanding the root cause of the problem.
- Replicating the issue.
- Resolving the issue.
Understand the Root Cause of the TypeError: list indices must be integers or slices, not str
in Python
TypeError
mainly occurs in Python whenever there is a problem with the type of data being operated. For example, adding two strings would result in a TypeError
because you cannot add two strings.
Replicate the TypeError: list indices must be integers or slices, not str
in Python
This problem can be replicated with the help of the following block of code.
Let’s assume we’re trying to assign the score as 1
, age as 2
and rating as 3
for a particular player. We’re then trying to access the score of the same player.
player = [1, 2, 3]
print(player["score"])
As we can see from the code block above, we are trying to find an attribute score from an array named player
.
The output of the code block is below.
TypeError: list indices must be integers or slices, not str
Solve the Error in Python
To resolve this problem, we can directly use a dictionary in Python. The previously illustrated code can be changed to the following to eliminate the error.
player = {"score": 1, "age": 2, "rating": 3}
print(player["score"])
The output of the code block is below.
1
Thus, with the help of this tutorial, we can solve this TypeError
in Python.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python