How to Avoid the TypeError: Input Expected at Most 1 Argument, Got 3 in Python
- Take User Input in Python
-
Avoid the
TypeError: input expected at most 1 argument, got 3
Error in Python
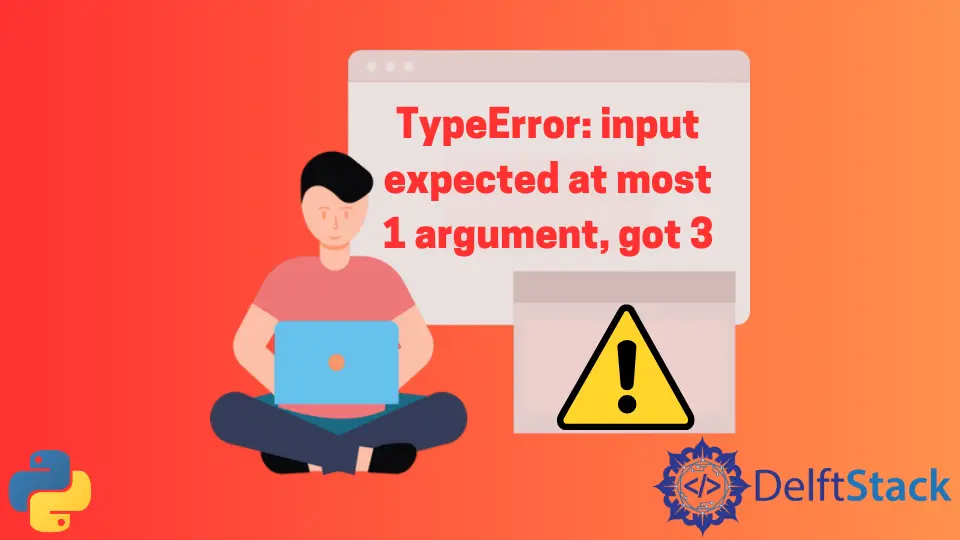
In Python programming, we have two built-in methods to take the user’s input: input(prompt)
and raw_input(prompt)
. The second method, raw_input(prompt)
, is used in the older versions of Python like 2.X, and the input(prompt)
is still in use.
This article will focus on the input(prompt)
method.
Take User Input in Python
In Python, we will use the built-in input()
function to take input from users and use it in the program accordingly.
Use of the input()
function in Python:
name = input("Hey! What is your name? ")
print("Nice to meet you ", name)
Output:
Hey! What is your name? Zeeshan Afridi
Nice to meet you Zeeshan Afridi
In the above program, we have used the input(prompt)
function to ask the user’s name. As the user inputs their name, it will be stored in the name
variable, which you can later utilize in your program anywhere needed.
Avoid the TypeError: input expected at most 1 argument, got 3
Error in Python
In computer programming, the TypeError is a syntactical error that means the developer has made some mistakes writing the program because of an invalid syntax of input or passing extra arguments to the functions. And one of the common errors is TypeError: input expected at most 1 argument, got 3
.
Let’s understand it through an example:
name = input("Please enter your name: ")
age = input("Please enter your age: ")
print("Your good name is ", name, " and your age is ", age)
goal = input("What is your life goal Mr. ", name, " ?")
print(goal)
print("Awesome!")
Output:
Please enter your name: Zeeshan Afridi
Please enter your age: 24
Your good name is Zeeshan Afridi and your age is 24
TypeError: input expected at most 1 argument, got 3
In the above program, the first 3 lines are executed smoothly, but the next line of code goal = input("What is your life goal Mr. ", name, " ?")
has thrown an error TypeError: input expected at most 1 argument, got 3
.
This is because the Python input()
function only expects a single argument, but we have passed three arguments to the input()
function. The first argument is the string What is your life goal Mr.
, the second argument is the variable name
, and the last argument is again a string ?
.
We have separated these arguments with the comma ,
, which indicates that each is a separate argument. But, if you want to print the input prompt as it is, you have to concatenate these arguments to make it a single argument to meet the input()
function’s expectation.
Let us understand it through an example:
name = input("Please enter your name: ")
age = input("Please enter your age: ")
print("\nYour good name is ", name, " and your age is ", age)
goal = input("What is your life goal Mr. " + name + " ? ")
print("Awesome!")
Output:
Please enter your name: Zeeshan Afridi
Please enter your age: 24
Your good name is Zeeshan Afridi and your age is 24
What is your life goal Mr. Zeeshan Afridi ? To be a Python Developer
Awesome!
In the above program, we have concatenated the argument of the input()
function with the help of the plus symbol +
to make them a single argument and to avoid the input TypeError.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python