How to Fix the TypeError: Decoding Unicode Is Not Supported in Python
-
the
TypeError: decoding Unicode is not supported
in Python -
Fix the
TypeError: decoding Unicode is not supported
in Python - Conclusion
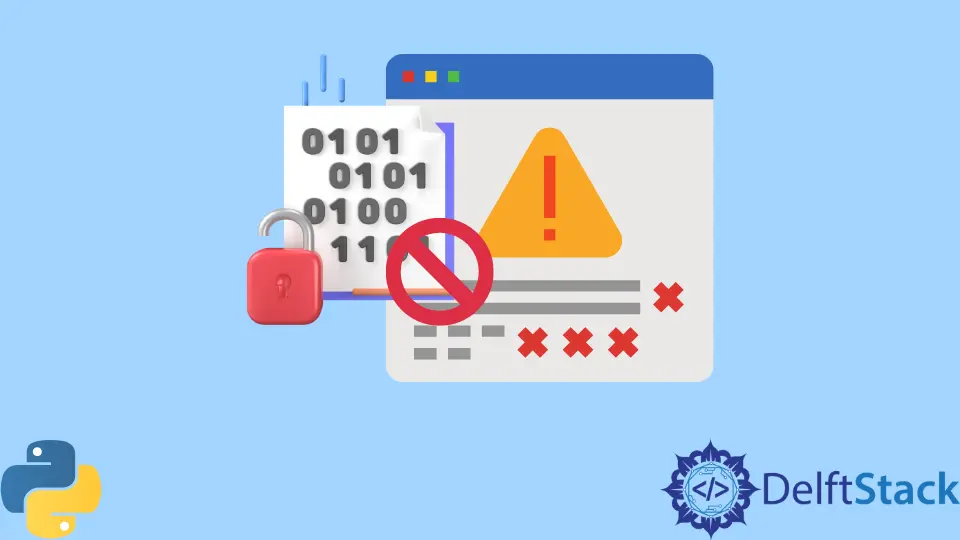
This article will discuss solving the TypeError: decoding Unicode is not supported
error in Python.
the TypeError: decoding Unicode is not supported
in Python
A Unicode string is a collection of code points, which are digits ranging from 0
to 0x10FFFF
(1,114,111 decimal). The set of code units that must be used to represent this series of code points in memory is then mapped to 8-bit bytes.
A character encoding, or simply an encoding, is a set of rules determining how to convert a Unicode text into a series of bytes. Your initial thought could be to use 32-bit integers as the coding unit and then to use the CPU’s representation of 32-bit integers.
One of the most widely used encodings is UTF-8, which Python frequently uses by default. UTF stands for Unicode Transformation Format, and 8
indicates that the encoding uses 8-bit values.
There are other encodings, such as UTF-16 and UTF-32, although they are less popular than UTF-8.
Example Code:
result = unicode(google.searchGoogle(param), "utf-8").encode("utf-8")
Output:
TypeError: decoding Unicode is not supported
Fix the TypeError: decoding Unicode is not supported
in Python
To solve this error, we have to change syntax of this line result = Unicode(google.searchGoogle(param), "utf-8").encode("utf-8")
to result = google.searchGoogle(param).encode("utf-8")
. UTF-8 should be removed after google.searchGoogle(param)
.so this will solve the error decoding Unicode is not supported, as shown in the below example
result = google.searchGoogle(param).encode("utf-8")
Another is the TypeError: decoding str is not supported
in Python. This occurs when we repeatedly try to convert an object to a string or when we call the str()
method without first passing it bytes object.
Example Code:
str("even", str(123))
str("abc", encoding="utf-8")
Output:
TypeError: decoding str is not supported
The str
class is referenced twice in the above example; each call is nested within the other. A proper bytes object is not provided; instead, the encoding keyword argument is set.
Only when a valid bytes object is given can the encoding be configured.
To avoid this error, you can use a formatted string literal. This error can be prevented using the string format interface or the more recent formatted string literals.
Additionally, these options offer more potent, adaptable, and extendable methods.
str1 = "even"
num1 = 2, 4, 6, 8
result = f"{str1} {num1}"
print(result)
Output:
even (2, 4, 6, 8)
Conclusion
Sometimes when we want to encode our output to UTF-8 but we are not using the right syntax, the error Python decoding Unicode is not supported
arises because a correct bytes object is not supplied. Another error decoding str
is not supported in Python occurs.
Only when a valid bytes object is provided can the encoding be defined. The above article fixes these errors.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python