How to Resolve the TypeError: Can't Multiply Sequence by Non-Int of Type STR in Python
-
Cause of the
TypeError: Can't Multiply Sequence by Non-Int of Type Str
in Python -
Convert Both the Values Into Int Values to Resolve the
TypeError: Can't Multiply Sequence by Non-Int of Type Str
-
Convert a Single String Value Into an Int Value to Resolve the
TypeError: Can't Multiply Sequence by Non-Int of Type Str
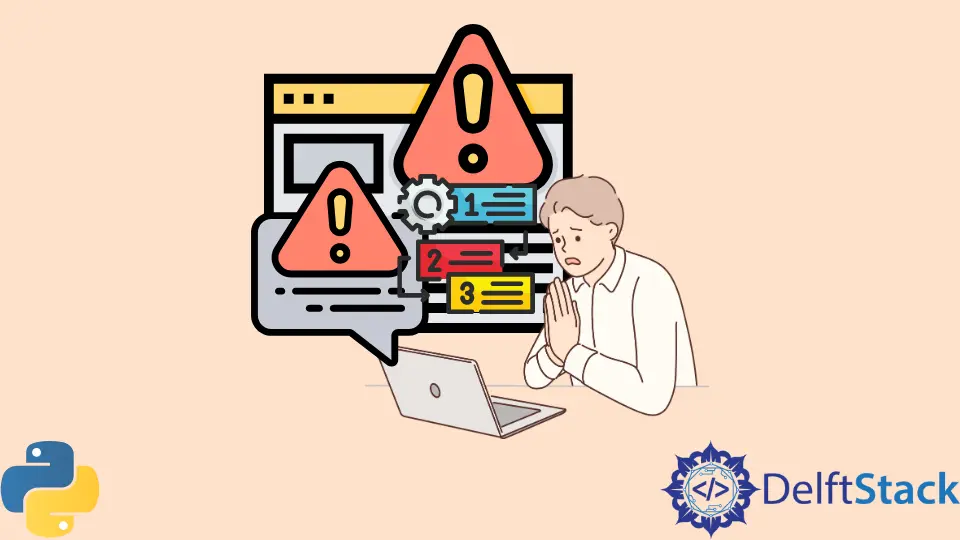
Python provides a lot of variety in data types, and the str
data type is one of those offered. Strings in Python represent a sequence of Unicode characters, surrounded by quotation marks, single or double quotes.
This tutorial focuses on and aims to provide a solution to the TypeError: can't multiply sequence by non-int of type 'str'
error.
Cause of the TypeError: Can't Multiply Sequence by Non-Int of Type Str
in Python
The TypeError: can't multiply sequence by non-int of type 'str'
error generally occurs when a string is multiplied with another string without converting the specified string into a floating-point or an integer first.
Python does not allow the process of multiplication of a string with another string. It only allows the multiplication of a string with an integer value; however, there is a quick way to resolve this error.
This error can be resolved by converting the content of the string to an integer value. Let us take an example of a string that stores a number and another string that holds another number.
x = "10"
y = "15"
Now, multiplying these strings together would result in an error.
x = "10"
y = "15"
z = x * y
print(z)
The above code provides the following output:
Traceback (most recent call last):
File "/tmp/sessions/d1292bc9a03e82de/main.py", line 3, in <module>
z = x*y
TypeError: can't multiply sequence by non-int of type 'str'
Convert Both the Values Into Int Values to Resolve the TypeError: Can't Multiply Sequence by Non-Int of Type Str
To resolve this error, we will convert both string values into integer values and then complete the multiplication process.
The following code converts both the values into int values to resolve the TypeError: can't multiply sequence by non-int of type 'str'
error.
x = "10"
y = "15"
z = int(x) * int(y)
print(z)
The above code provides the following output:
150
Convert a Single String Value Into an Int Value to Resolve the TypeError: Can't Multiply Sequence by Non-Int of Type Str
Alternatively, we can convert either one of the string values to an int value while taking the other one as it is. However, the result would vary as the value of the variable taken as a string would be displayed n
times, where n
would be the value of the string converted to an int.
The following code converts a single string value into an int value to resolve the TypeError: can't multiply sequence by non-int of type 'str'
error.
x = "10"
n = "15"
z = x * int(n)
print(z)
The above code provides the following output:
101010101010101010101010101010
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python