How to Fix TypeError: __str__ Returned Non-String but Printing Output
- Python Returned Non-String Error but Printing Output
-
Resolve TypeError:
__str__
Returned Non-String -
__str__
Returned Non-String With__repr__()
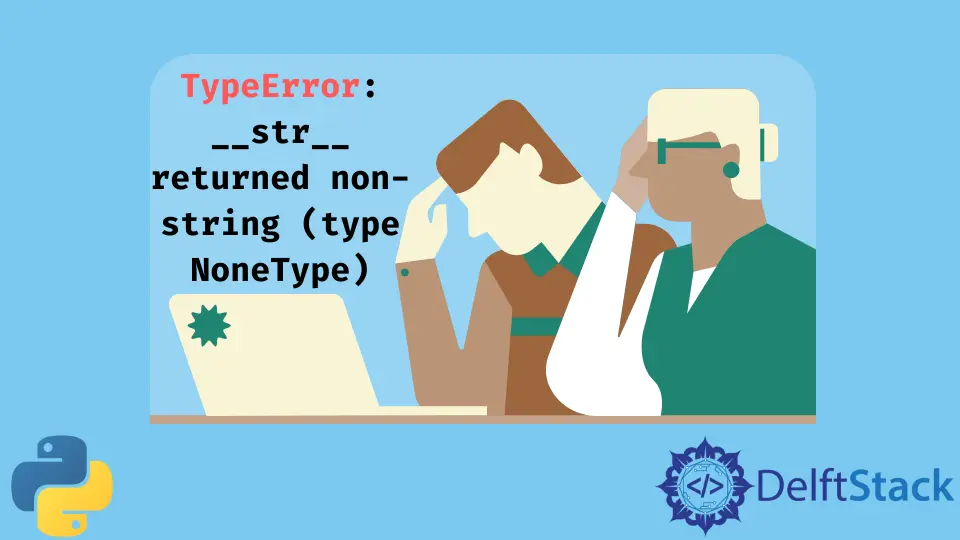
This article aims to solve the problem that occurs when we try to print the string instead of using a return statement in the function.
Python Returned Non-String Error but Printing Output
The following code shows the TypeError: __str__
returned non-string, but it still prints the output.
Example Code:
class xy:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
print("X={0}, Y={1}")
if __name__ == "__main__":
x_y = xy("value of x is 1", "value of y is 2")
print(x_y)
Output:
TypeError Traceback (most recent call last)
<ipython-input-1-7b40a083df6c> in <module>
10 if __name__ == "__main__":
11 x_y = xy('value of x is 1','value of y is 2')
---> 12 print(x_y)
TypeError: __str__ returned non-string (type NoneType)
Why are we getting an error i.e. __str__
returned non-string (type NoneType)? First, we need to understand the working of the __str__
operator.
The __str__
operator is primarily designed to return the string, but we mostly use it to print the string. There are two terms for __str__
in Python, used interchangeably, operator or Dunder method.
In the above code, the __str__
method directly calls the print()
function and prints the string but does not return a string; that’s why it is showing the above error.
Additionally, our __str__
operator prints the string and returns nothing; that’s why it uses None in error.
Resolve TypeError: __str__
Returned Non-String
We can solve this problem using the return statement in the __str__
method. So, we must return the string in the __str__
method instead of printing it. See the following code.
Example Code:
class xy:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return ("X={0}, Y={1}").format(self.x, self.y)
if __name__ == "__main__":
x_y = xy("value of x is 1", "value of y is 2")
print(x_y)
Output:
X=value of x is 1, Y=value of y is 2
The above code has one more problem. And the problem is, if the value we are returning is not a string, it will show the exact error, but this time, the variable type is not None
.
Example Code:
class xy:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return 123
if __name__ == "__main__":
x_y = xy("value of x is 1", "value of y is 2")
print(x_y)
Output:
TypeError Traceback (most recent call last)
<ipython-input-9-173c41d63dab> in <module>
12 if __name__ == "__main__":
13 x_y = xy('value of x is 1','value of y is 2')
---> 14 print(x_y)
TypeError: __str__ returned non-string (type int)
To solve it, we need to typecast the value and wrap it with the str()
method, which means converting the type of the variable to string as it is the requirement of the str
method.
Example Code:
class xy:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return str(123)
if __name__ == "__main__":
x_y = xy("value of x is 1", "value of y is 2")
print(x_y)
Output:
123
Now, the code runs for all the possible cases because we solved all the expected problems and met the requirements of the str
method, i.e. it only returns the string value.
__str__
Returned Non-String With __repr__()
The __repr__()
method does the same thing as the str
method. See the following code.
Example Code:
class calculate(object):
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __repr__(self):
print("(%r, %r, %r)" % (self.x, self.y, self.z))
equation = calculate(1, 2, 3)
print(equation)
Output:
(1, 2, 3)
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-6-0ee74d4c74c8> in <module>
8
9 equation = calculate(1, 2, 3)
---> 10 print(equation)
TypeError: __str__ returned non-string (type NoneType)
The above code also prints the string instead of returning it from the __repr__()
method. Therefore, we can solve it by using the return
statement instead of printing the string using the __repr__()
method.
Example Code:
class calculate(object):
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __repr__(self):
return str("(%r, %r, %r)" % (self.x, self.y, self.z))
equation = calculate(1, 2, 3)
print(equation)
Output:
(1, 2, 3)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python