How to Convert Tuple to String in Python
-
Use the
str.join()
Function to Convert Tuple to String in Python -
Use the
reduce()
Function to Convert a Tuple to a String in Python -
Use the
for
Loop to Convert a Tuple to a String in Python
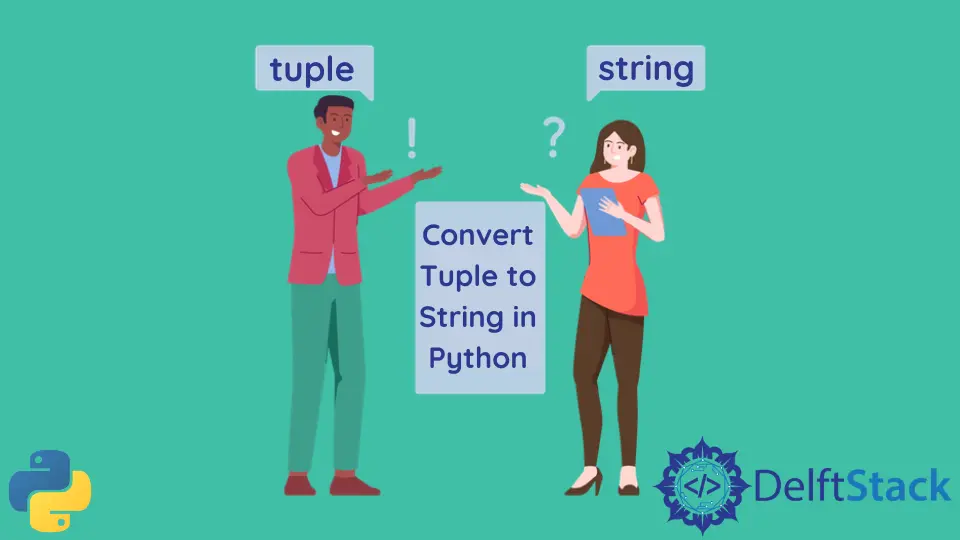
In Python, multiple items can be stored in a single variable using tuples. Strings can be defined as the cluster of characters that are enclosed in single or double-quotes.
This tutorial will discuss different methods to convert a tuple to a string in Python.
Use the str.join()
Function to Convert Tuple to String in Python
The join()
function, as its name suggests, is used to return a string that contains all the elements of sequence joined by an str separator.
We use the join()
function to add all the characters in the input tuple and then convert it to string.
The following code uses the str.join()
function to convert a tuple into a string.
tup1 = ("h", "e", "l", "l", "o")
# Use str.join() to convert tuple to string.
str = "".join(tup1)
print(str)
Output:
hello
A delimiter, like a comma, can also be added to the converted string. The following code uses the str.join()
method with a delimiter ,
to convert a tuple into a string.
tup1 = ("h", "e", "l", "l", "o")
# Use str.join() to convert tuple to string.
str = ",".join(tup1)
print(str)
Output:
h,e,l,l,o
Use the reduce()
Function to Convert a Tuple to a String in Python
The reduce(fun, seq)
function is utilized to apply a particular function passed in the entirety of the list components referenced in the sequence passed along.
In this method, we need to import functools
and operator
modules to run the codes successfully.
The functools
module provides the ability for high-order functions to work on other functions.
The following code uses the reduce()
function to convert a tuple to a string.
import functools
import operator
tup1 = ("h", "e", "l", "l", "o")
# Use reduce() to convert tuple to string.
str = functools.reduce(operator.add, (tup1))
print(str)
Output:
hello
Use the for
Loop to Convert a Tuple to a String in Python
A basic for
loop can also be used to iterate on the entirety of the elements in the tuple and then append the elements to a string.
We use a tuple and an empty string. And all the elements of the tuple are iterated over to be appended to the empty string.
The following code uses the for
loop to convert a tuple to a string in Python.
tup1 = ("h", "e", "l", "l", "o")
str = ""
# Use for loop to convert tuple to string.
for item in tup1:
str = str + item
print(str)
Output:
hello
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Tuple
- Named Tuple in Python
- How to Iterate Through a Tuple in Python
- How to Create a List of Tuples From Multiple Lists and Tuples in Python
- Tuple Comprehension in Python
- How to Convert Tuple to List in Python