在 Python 中將元組轉換為字串
Vaibhhav Khetarpal
2021年4月29日
Python
Python Tuple
Python String
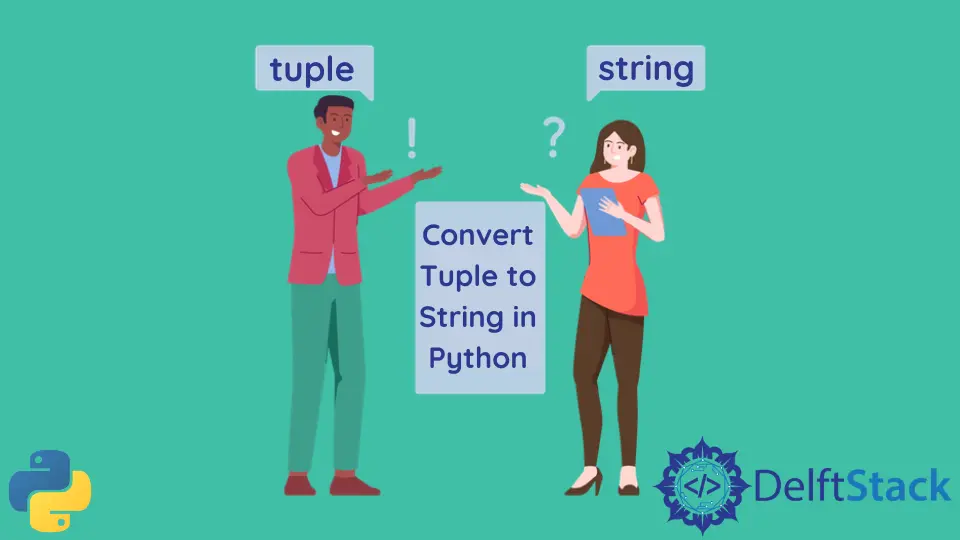
在 Python 中,可以使用元組將多個專案儲存在單個變數中。字串可以定義為用單引號或雙引號引起來的字元簇。
本教程將討論在 Python 中將元組轉換為字串的不同方法。
在 Python 中使用 str.join()
函式將元組轉換為字串
顧名思義,join()
函式用於返回一個字串,該字串包含由 str 分隔符連線的所有序列元素。
我們使用 join()
函式在輸入元組中新增所有字元,然後將其轉換為字串。
以下程式碼使用 str.join()
函式將元組轉換為字串。
tup1 = ("h", "e", "l", "l", "o")
# Use str.join() to convert tuple to string.
str = "".join(tup1)
print(str)
輸出:
hello
分隔符(如逗號)也可以新增到轉換後的字串中。以下程式碼使用帶有分隔符 ,
的 str.join()
方法將元組轉換為字串。
tup1 = ("h", "e", "l", "l", "o")
# Use str.join() to convert tuple to string.
str = ",".join(tup1)
print(str)
輸出:
h,e,l,l,o
在 Python 中使用 reduce()
函式將元組轉換為字串
reduce(fun, seq)
函式用於應用在傳遞的序列中引用的整個列表元件中傳遞的特定函式。
在這種方法中,我們需要匯入 functools
和 operator
模組以成功執行程式碼。
functools
模組提供了使高階函式可以在其他函式上工作的功能。
以下程式碼使用 reduce()
函式將元組轉換為字串。
import functools
import operator
tup1 = ("h", "e", "l", "l", "o")
# Use reduce() to convert tuple to string.
str = functools.reduce(operator.add, (tup1))
print(str)
輸出:
hello
在 Python 中使用 for
迴圈將元組轉換為字串
一個基本的 for
迴圈也可以用於遍歷元組中的所有元素,然後將這些元素附加到字串中。
我們使用一個元組和一個空字串。元組的所有元素都被迭代以附加到空字串中。
以下程式碼使用 for
迴圈在 Python 中將元組轉換為字串。
tup1 = ("h", "e", "l", "l", "o")
str = ""
# Use for loop to convert tuple to string.
for item in tup1:
str = str + item
print(str)
輸出:
hello
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn相關文章 - Python Tuple
- 如何在 Python 中將列表轉換為元組
- Python 中的命名元組
- 在 Python 中從多個列表和元組建立元組列表
- 在 Python 中遍歷一個元組
- Python 中的元組理解
- Python 中將元組轉換為列表