How to Create a List of Tuples From Multiple Lists and Tuples in Python
- Manually Create a List of Tuples From Multiple Lists and Tuples in Python
-
Create a List of Tuples From Multiple Lists and Tuples With the
zip()
Method in Python
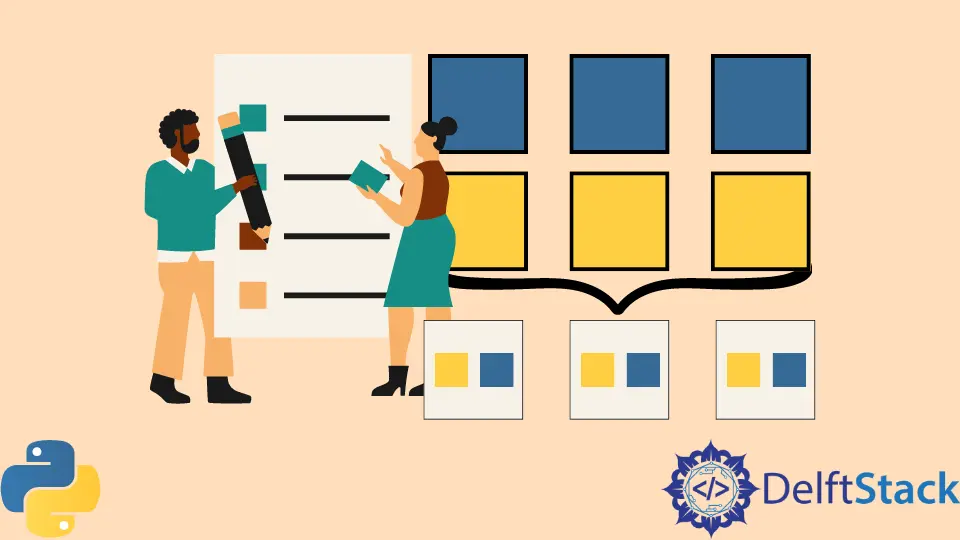
When working with multiple lists and tuples, we often have to combine the values present inside the objects. This approach makes iterating over them a lot simpler. When developing web applications or APIs using Python, this approach is mostly followed to ensure that we do not have any missing values. Moreover, it becomes very to iterate over these values inside HTML templates.
In this article, we will learn how to create a list of tuples and tuples from multiple lists in Python.
Manually Create a List of Tuples From Multiple Lists and Tuples in Python
The most basic approach of creating a list of tuples from multiple lists and tuples is to write a stub or utility function yourself. The function would accept multiple lists and tuples and return a list of tuples. While iterating over the lists, the logic would have to ensure that it only considers the smallest length across all the lists and adds elements only up to that index. The following Python code implements the discussed approach.
def convert(*args):
"""
Returns a list of tuples generated from multiple lists and tuples
"""
for x in args:
if not isinstance(x, list) and not isinstance(x, tuple):
return []
size = float("inf")
for x in args:
size = min(size, len(x))
result = []
for i in range(size):
result.append(tuple([x[i] for x in args]))
return result
a = [1.1, 2.2, 3.3, 4.4, 5.5, 6.6, 7.7, 8.8]
b = ["H", "E", "L", "L", "O"]
c = [True, False, False, True, True, True]
d = [100, 200, 300, 400]
result = convert(a, b, c, d)
print(result)
Output:
[(1.1, 'H', True, 100), (2.2, 'E', False, 200), (3.3, 'L', False, 300), (4.4, 'L', True, 400)]
The convert()
function accepts multiple lists and tuples as arguments. First, it checks if each passed argument is an instance of list
or tuple
. If not, it returns an empty list. Next, it finds the smallest length, say n
, across all the lists and tuples. This operation makes sure that we do not run into the IndexError
exception.
Once the smallest size is found, the convert()
function creates an empty list and iterates over all the arguments n
times. Each iteration creates a tuple and appends it to the empty list created before. Once the iteration is complete, the list of tuples is returned as an answer.
Considering the number of arguments as n
and the smallest length as m
, the time complexity of the above function is O(m * n)
, and the space complexity is also O(m * n)
. The time complexity is O(m * n)
because of the double nested for
loop that creates the result.
Create a List of Tuples From Multiple Lists and Tuples With the zip()
Method in Python
The zip()
function is Python’s built-in utility function. It accepts multiple iterable objects such as lists and tuples and returns an iterable object. This zip
object stores elements as a list of tuples. Implementing the zip()
function would be similar, not exact, to the convert()
function that we implemented in the last section.
The value returned by the zip()
function is an iterable zip
object. If we printed it, the output would be similar to the following.
<zip object at 0x7fee6d0628c0>
Now that we are done with the theory, let us understand how to use the zip()
method for our use case. Refer to the following code for the same.
a = [1.1, 2.2, 3.3, 4.4, 5.5, 6.6, 7.7, 8.8]
b = ("H", "E", "L", "L", "O")
c = [True, False, False, True, True, True]
d = [100, 200, 300, 400]
result = zip(a, b, c, d)
for x in result:
print(x)
Output:
(1.1, 'H', True, 100)
(2.2, 'E', False, 200)
(3.3, 'L', False, 300)
(4.4, 'L', True, 400)
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python