Named Tuple in Python
- What Is a Named Tuple in Python
- Difference Between Simple and Named Tuple in Python
- When to Use Named Tuple Instead of Simple Tuple in Python
- How to Use Named Tuple in Python
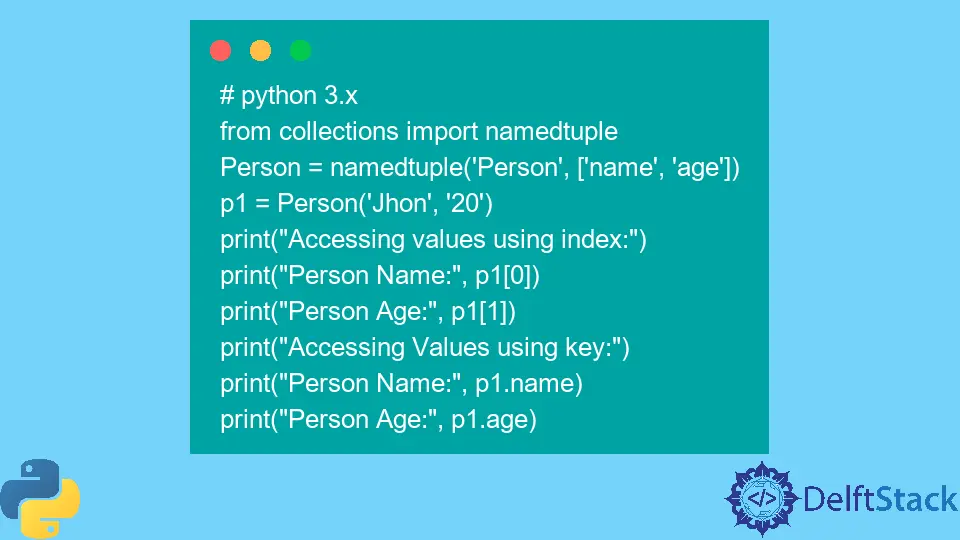
This article explains a named tuple, when and how to use it, and the difference between normal and named tuples in Python.
What Is a Named Tuple in Python
A named tuple is a special kind of tuple that has all the functionalities of a tuple. Named tuple was introduced in Python 2.6. Just like a dictionary, a named tuple contains key-value pair.
A value can be accessed using its key and also using its index. It is similar to a struct in C language.
Difference Between Simple and Named Tuple in Python
Named Tuple
is the object representation of a simple tuple. It is a subclass of simple tuples with named variables created programmatically using a factory function.
We can access the items of named tuple using the dot operator with the reference variable of the named tuple and by using the item’s index with the reference variable. While in a simple tuple, we can only use the item’s index with the reference variable to access it.
When to Use Named Tuple Instead of Simple Tuple in Python
We use named tuples instead of simple tuples when we want our code to look clean, understandable, and more pythonic.
For example, a person.age
looks cleaner than a person['age']
in the case of a dictionary. And, a person.age
also looks cleaner than a person[1]
in the case of the simple tuple.
How to Use Named Tuple in Python
To generate a named tuple, we first import the namedtuple()
, a factory function inside the collections module. The factory function is a function that is used for manufacturing a class of different prototypes.
Using this factory function, we specify the name and attributes of the class. So the general syntax is below.
Class_Name = namedtuple('Class_Name', ['field_1', 'field_2', ....., 'field_n'])
Then we can instantiate the class. Instantiating the class will make an object and assign values to the corresponding fields.
So the general syntax is below.
Ref_Variable_Name = Class_Name('Value_1', 'Value_2', ....., 'Value_n')
We can access the value of a specific field using the dot notation. So the general syntax is below.
Ref_Variable_Name.field_name
In the following complete example code, the name of the class is Person
, and its attributes are name
and age
. Then we make an instance, p1
, of the class Person
.
Now, this instance p1
is a named tuple. We have accessed the class variables using object notation and by the index with the reference variable in the code.
But object notation is clearer and understandable, which is the motive behind the named tuple.
Example Code:
# python 3.x
from collections import namedtuple
Person = namedtuple("Person", ["name", "age"])
p1 = Person("Jhon", "20")
print("Accessing values using index:")
print("Person Name:", p1[0])
print("Person Age:", p1[1])
print("Accessing Values using key:")
print("Person Name:", p1.name)
print("Person Age:", p1.age)
Output:
# python 3.x
Accessing values using index:
Person Name: Jhon
Person Age: 20
Accessing Values using key:
Person Name: Jhon
Person Age: 20
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn