How to Transpose a Matrix in Python
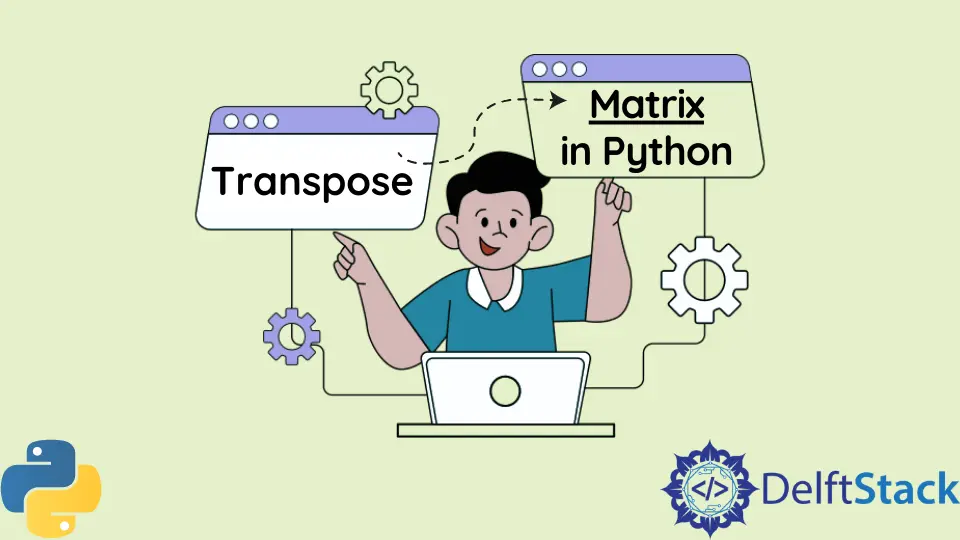
Matrices can be represented using multi-dimensional lists in Python. Once expressed, we can perform all the operations that we can perform over matrices in mathematical theory, such as matrix multiplication, matrix addition, and subtraction.
This article will talk about one such operation. We will learn how to transpose a matrix using Python with the help of some relevant examples.
Transpose a Matrix in Python
To transpose a matrix in Python, we can write a simple stub function and use for
loops for transposing an input matrix.
def transpose(matrix):
if matrix == None or len(matrix) == 0:
return []
result = [[None for i in range(len(matrix))] for j in range(len(matrix[0]))]
for i in range(len(matrix[0])):
for j in range(len(matrix)):
result[i][j] = matrix[j][i]
return result
def print_matrix(matrix):
for row in matrix:
print(*row)
array = [[1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15]]
result = transpose(array)
print_matrix(result)
Output:
1 6 11
2 7 12
3 8 13
4 9 14
5 10 15
The above method’s time and space complexity are O(mn)
, where m
is the number of rows in the input matrix and n
is the number of columns in the input matrix.
The time complexity is O(mn)
because we are iterating over the whole input matrix. The space complexity is O(mn)
because we create a copy of the input matrix and store it in a separate variable.
The above stub function first creates a temporary matrix of size n x m
with None
values if the shape of the input matrix is m x n
. Here, m
is the number of rows in the input matrix, and n
is the number of columns in the input matrix.
Next, it iterates over the input matrix using two nested for
loops. Inside the two for
loops, the None
values are replaced with the actual values from the input matrix (result[i][j] = matrix[j][i]
). Lastly, the temporary matrix is returned as the output.
Instead of using two nested for
loops for filling the temporary matrix, we can also write the transposing logic in a single line to save some space.
def transpose(matrix):
if matrix == None or len(matrix) == 0:
return []
return [[matrix[i][j] for i in range(len(matrix))] for j in range(len(matrix[0]))]
def print_matrix(matrix):
for row in matrix:
print(*row)
array = [[1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15]]
result = transpose(array)
print_matrix(result)
Output:
1 6 11
2 7 12
3 8 13
4 9 14
5 10 15
This solution’s time and space complexity are also the same as the previous solution’s, O(mn)
.
Transpose a Matrix in Python Using the NumPy
Module
The NumPy
is a Python package that is rich with utilities for playing around with large multi-dimensional matrices and arrays and performing both complex and straightforward mathematical operations over them. These utilities are not only dynamic to the inputs but also highly optimized and fast.
Using the NumPy
module in Python, we can transpose a matrix in two ways. The first is by using the T
attribute of a NumPy
array, and the second is by calling the transpose()
method of a NumPy
array.
Refer to the following Python code to understand how to use the two mentioned methods.
NumPy
array using the numpy.array()
method. To learn more about this method, click here.import numpy as np
array = [[1, 2, 3, 4, 5], [6, 7, 8, 9, 10], [11, 12, 13, 14, 15]]
array = np.array(array)
print(array.T) # First Method
print(array.transpose()) # Second Method
Output:
[[ 1 6 11]
[ 2 7 12]
[ 3 8 13]
[ 4 9 14]
[ 5 10 15]]
[[ 1 6 11]
[ 2 7 12]
[ 3 8 13]
[ 4 9 14]
[ 5 10 15]]
The transpose()
accepts an axes
argument that can be used to perform some cool transposing modifications over a NumPy
array. To learn about this method more, click here.