Python Numpy transpose() Function
-
Syntax of
numpy.transpose()
: -
Example Codes:
numpy.transpose()
Method -
Example Codes: Set
axes
Parameter innumpy.transpose()
Method
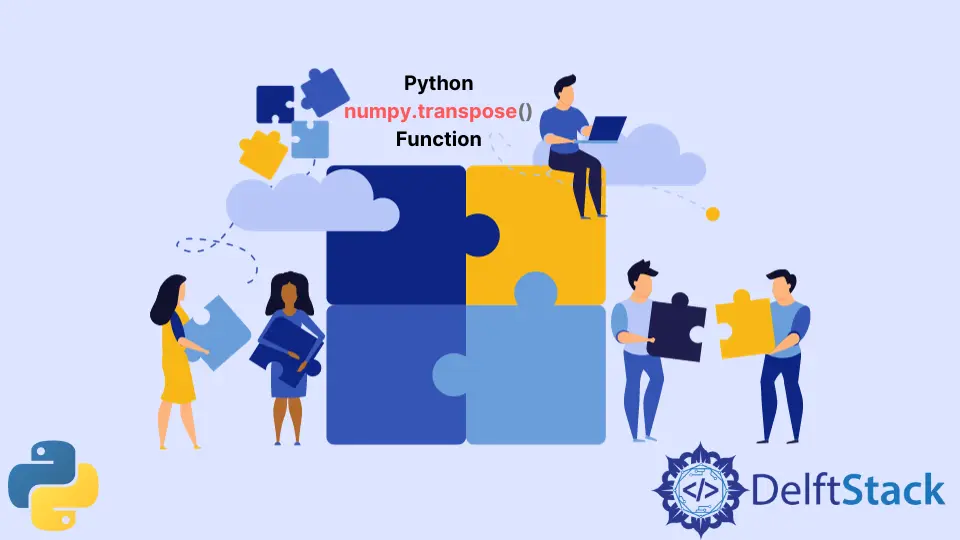
Python Numpy numpy.transpose()
reverses the axes of the input array or simply transposes the input array.
Syntax of numpy.transpose()
:
numpy.transpose(ar, axes=None)
Parameters
ar |
Array or Object which could be converted into an array. |
axis |
Tuple or list of integers. It specifies the order of axes after permutation. |
Return
It returns transpose of the input array if it is a 2-D, however, the input array remains unchanged if it is 1-D.
Example Codes: numpy.transpose()
Method
import numpy as np
x=np.array([[2,3,3],
[3,2,1]])
print("Matrix x:")
print(x)
x_transpose=np.transpose(x)
print("\nTranspose of Matrix x:")
print(x_transpose)
Output:
Matrix x:
[[2 3 3]
[3 2 1]]
Transpose of Matrix x:
[[2 3]
[3 2]
[3 1]]
It returns the transposed version of the input array x
. The rows of matrix x
become columns of matrix x_transpose
and columns of matrix x
become rows of matrix x_transpose
.
However, if we pass a 1-D array in the numpy.transpose()
method, there is no change in the returned array.
import numpy as np
x=np.array([2,3,3])
print("Matrix x:")
print(x)
x_transpose=np.transpose(x)
print("\nTranspose of Matrix x:")
print(x_transpose)
Output:
Matrix x:
[2 3 3]
Transpose of Matrix x:
[2 3 3]
It shows 1-D array remains unchanged after passing through the np.transpose()
method.
Example Codes: Set axes
Parameter in numpy.transpose()
Method
import numpy as np
x = np.random.random((1, 2, 3, 5))
print("Shape of x:")
print(x.shape)
x_permuted=np.transpose(x, (3, 0, 2,1))
print("\nShape of x_permuted:")
print(x_permuted.shape)
Output:
Shape of x:
(1, 2, 3, 5)
Shape of x_permuted:
(5, 1, 3, 2)
Here, axes
is passed as second parameter to the numpy.transpose()
method.
The ith
axis of the returned array will be axes[i]-th
axis of the input array.
Hence 0th
axis in the above example of x
becomes the first axis of x_permuted
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn