Diagonal Matrix in Python
- What is a Matrix in Python
- Matrix Representation in Python
- Different Types of Matrices in Python
- How to Create a Diagonal Matrix Using NumPy in Python
- Convert Vectors to Diagonal Matrix in Python
- How to Get Diagonals of a Matrix in Python
- Where Are the Diagonal Matrices Used in Python
- Conclusion
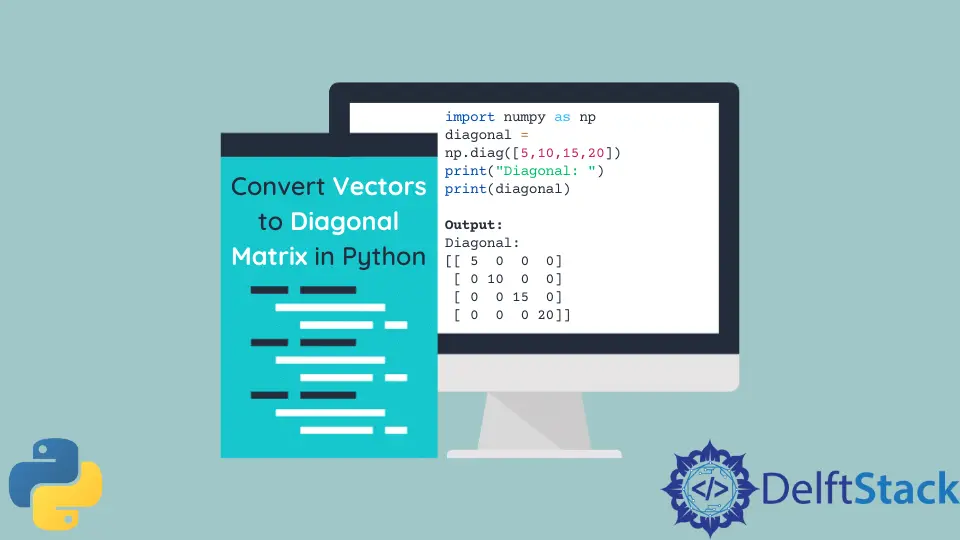
This article on matrices in Python provides insight into different types of matrices, converting vector matrices into diagonal matrices in Python, and shares the uses of diagonal matrices in programming.
Let us discuss first what a matrix is and then move on to diagonal matrices and how you can convert vector matrices to diagonal matrices in Python.
What is a Matrix in Python
A matrix
is a unique structure, which is 2-dimensional, for storing data rows and columns. It can hold all kinds of values such as integers, strings, expressions, special symbols, and more.
A matrix is represented as a collection of rows and columns. The matrix structure is denoted by RxC
, where R
denotes the number of rows and C
denotes the number of matrix columns, respectively.
There are two methods for creating matrices in Python, using lists and the NumPy
library.
For now, we will use lists to explain matrices then move on to the NumPy
library and its functions for the creation of matrices using arrays and converting vector matrices to diagonal matrices for a better understanding of both methods.
The given code represents a 3x3 matrix of alphabets.
X = [["a", "b", "c"], ["d", "e", "f"], ["g", "h", "i"]]
print(X, "is our Matrix")
Output:
[['a', 'b', 'c'], ['d', 'e', 'f'], ['g', 'h', 'i']] is our Matrix
The elements present in the matrix are also identified using their column and row number.
So, in the matrix represented above, the element a
belongs to row 1 of column 1. So a
is present at location (1,1)
inside the matrix.
Similarly, the other elements inside the first row are b(1,2)
and c(1,3)
. Moving on to the second row, d(2,1)
, e(2,2)
, f(2,3)
and then g(3,1)
, h(3,2)
, and i(3,3)
for the third row of the matrix.
Matrix Representation in Python
It would be best to remember that we always put the row number first and then the column number. The correct representation of an element X
inside a matrix becomes X (R, C)
, where R
and C
represent the row and column where the element is present.
A matrix can have any name but is generally denoted by a capital letter.
Let us take a look at how the above matrix is represented. Here, X
represents a 3x3 matrix of alphabets, Y
represents a 2x2 matrix of numbers, and Z
represents a 2x3 matrix of strings.
X = [["a", "b", "c"], ["d", "e", "f"], ["g", "h", "i"]]
Y = [[27, 34], [61, 18]]
Z = [["one", "two", "three"], ["four", "five", "six"]]
print(X, "is 3x3")
print(Y, "is 2x2")
print(Z, "is 2x3")
Output:
[['a', 'b', 'c'], ['d', 'e', 'f'], ['g', 'h', 'i']] is 3x3
[[27, 34], [61, 18]] is 2x2
[['one', 'two', 'three'], ['four', 'five', 'six']] is 2x3
Different Types of Matrices in Python
There are various types of matrices based on the arrangement of elements inside them, including:
- Vector Matrix: A matrix containing only a single row or a single column is a vector matrix. If it has a single row, it is called a row vector, and if it has a single column, it is known as a column vector.
import numpy as np
x = np.array([13, 42, 93])
y = np.array([[21], [72], [36]])
print("Row: ", x)
print("Column: ", y)
Output:
Row: [13 42 93]
Column: [[21]
[72]
[36]]
Naming conventions for vector matrices have to be different to represent that vector, so a bold and lower case letter is used to describe them.
- Square Matrix: A matrix with rows equal to the number of columns.
Square matrices can be 2x2, 3x3, 4x4, 5x5 matrices and so on. Y
and X
are square matrices as their R=C
.
Y = [[27, 34], [61, 18]]
X = [["a", "b", "c"], ["d", "e", "f"], ["g", "h", "i"]]
print("3X3 square: ", X)
print("2X2 sqaure: ", Y)
Output:
3X3 square: [['a', 'b', 'c'], ['d', 'e', 'f'], ['g', 'h', 'i']]
2X2 sqaure: [[27, 34], [61, 18]]
- Diagonal Matrix: A diagonal matrix is a matrix that has elements only in the diagonal positions, i.e., only positions with a similar row and column number filled. Diagonal elements occupy only
(1,1)
,(2,2)
,(3,3)
,(4,4)
positions and so on.
Here, X
and Y
are 2x2 and 3x3 diagonal matrices.
Y = [[27, 0], [0, 18]]
X = [[5, 0, 0], [0, 10, 0], [0, 0, 15]]
print("2x2 Diagonal:", X)
print("3x3 Diagonal:", Y)
Output:
2x2 Diagonal: [[5, 0, 0], [0, 10, 0], [0, 0, 15]]
3x3 Diagonal: [[27, 0], [0, 18]]
How to Create a Diagonal Matrix Using NumPy in Python
For the first portion of the article, we shared the first type of creation of Python matrices which is done using lists.
However, this approach is unsuitable as a particular library can help create matrices efficiently, known as NumPy
. NumPy
is a Python library that provides functions for creating and operations using arrays and matrices.
Moreover, creating matrices using lists displays the matrices as a list only, and you cannot use special library functions to modify or operate on these matrices.
You can use the NumPy
library’s arrays to create normal and diagonal matrices. You can install NumPy
using pip
.
pip install NumPy
You can create a diagonal matrix using the NumPy
array.
import numpy as np
X = np.array([[12, 0, 0], [0, 24, 0], [0, 0, 36]])
print("Diagonal: ")
print(X)
Output:
Diagonal:
[[12 0 0]
[ 0 24 0]
[ 0 0 36]]
Convert Vectors to Diagonal Matrix in Python
Sometimes, converting vector matrices to diagonal ones is also required while writing a program.
You can easily convert row or column vectors to a diagonal matrix using one of two functions for the conversion, which are:
diag
Function: You can use thediag
function in Python to construct a diagonal matrix. It is contained in theNumPy
library and uses two parameters.
The diag
function is numpy.diag(v, k=0)
where v
is an array that returns a diagonal matrix. Specifying v
is important, but you can skip k
.
If v
is an array, it returns a diagonal matrix 4x4 with the array elements as the diagonal matrix elements.
import numpy as np
diagonal = np.diag([5, 10, 15, 20])
print("Diagonal: ")
print(diagonal)
Output:
Diagonal:
[[ 5 0 0 0]
[ 0 10 0 0]
[ 0 0 15 0]
[ 0 0 0 20]]
diagflat
Function: Thediagflat
function is semantically similar to thediag
function and comes with theNumPy
library. Thediagflat
function isnumpy.diagflat(v, k=0)
wherev
andk
are the same as thediag
function.
import numpy as np
diagonal = np.diagflat([5, 10, 15, 20])
print("Diagonal: ")
print(diagonal)
Output:
Diagonal:
[[ 5 0 0 0]
[ 0 10 0 0]
[ 0 0 15 0]
[ 0 0 0 20]]
The value of k
in both functions is not necessary. But the variable can be given a value to offset the position of the starting diagonal element, thereby changing the position of all elements.
Suppose the same example but with a positive and negative value for k
this time.
import numpy as np
# Diagonal with k as 1
diagonal = np.diagflat([5, 10, 15, 20], 1)
print("Diagonal with k=1: ")
print(diagonal)
# Diagonal with k as -1
diagonal2 = np.diag([5, 10, 15, 20], -1)
print("Diagonal with k=-1: ")
print(diagonal2)
Output:
Diagonal with k=1:
[[ 0 5 0 0 0]
[ 0 0 10 0 0]
[ 0 0 0 15 0]
[ 0 0 0 0 20]
[ 0 0 0 0 0]]
Diagonal with k=-1:
[[ 0 0 0 0 0]
[ 5 0 0 0 0]
[ 0 10 0 0 0]
[ 0 0 15 0 0]
[ 0 0 0 20 0]]
If you provide k=1
, the first diagonal element offsets one column, and an extra row is added below. Still, when you provide k=-1
, the first diagonal element offset one row, and an additional column is added to the right.
How to Get Diagonals of a Matrix in Python
Numpy has another function known as diagonal. The diagonal function is used to get the values of all the diagonal elements of a matrix.
import numpy as np
X = np.array([[12, 0, 0], [0, 24, 0], [0, 0, 36]])
de = X.diagonal()
print("Diagonal elements: ", de)
Output:
Diagonal elements: [12 24 36]
Here, the diagonal
function is used to get an array of diagonal elements of the matrix.
Where Are the Diagonal Matrices Used in Python
Diagonal matrices are an essential component of mathematical functions and programs. These are used while working in linear algebra and represent linear maps.
Diagonal matrices are also an integral component of partial differential equations as they provide an easy way of storing different values of a single function at various points.
Conclusion
This is the end of another informative guide. You have learned the basics of matrices, their different types, converting vector matrices or arrays into diagonal matrices, and where they are applied in programming.