How to Print Matrix in Python
-
Use the
for
Loop to Print the Matrix in Python - Use the List Comprehension Method to Print the Matrix in Python
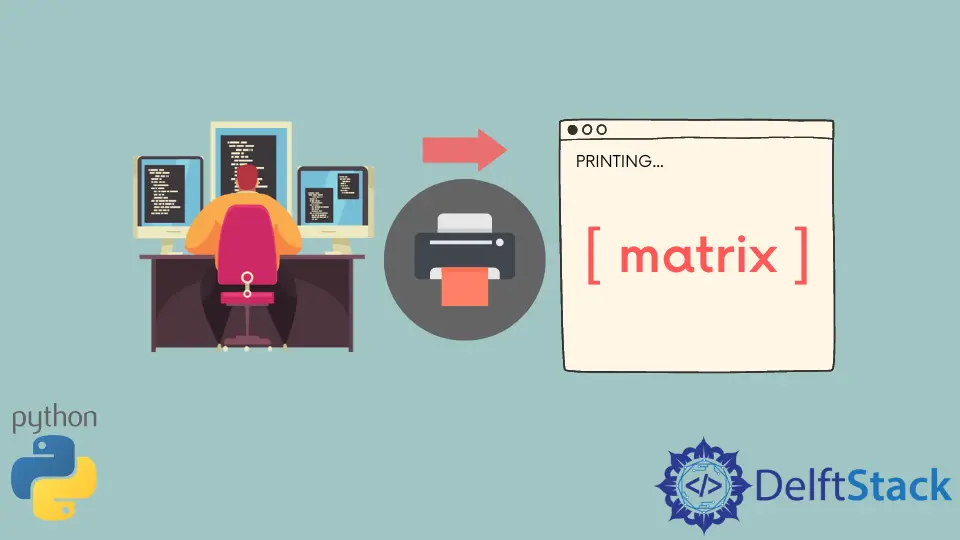
Matrices are used highly in mathematics and statistics for data representation and solving multiple linear equations. In programming, 2D arrays are treated as a matrix.
In Python, we have many functions and classes available for performing different operations on matrices. In this tutorial, we will learn how to print a matrix in Python.
We show how a 2-D array is normally printed in Python with all the square brackets and no proper spacing in the following code.
import numpy as np
a = np.array([[1, 2, 3], [3, 4, 5], [7, 8, 9]])
print(a)
Output:
[[1 2 3]
[3 4 5]
[7 8 9]]
In the methods discussed below, we will print the array in a clean matrix type format.
Use the for
Loop to Print the Matrix in Python
This method will iterate through the matrix using the for
loop and print each row individually after properly formatting it. The following code shows how.
import numpy as np
a = np.array([[1, 2, 3], [3, 4, 5], [7, 8, 9]])
for line in a:
print(" ".join(map(str, line)))
Output:
1 2 3
3 4 5
7 8 9
We use the map
function, which converts the whole row to a string, and then we apply the join
function to this whole row which converts it all to a single string and separate elements by the separator specified.
Use the List Comprehension Method to Print the Matrix in Python
List comprehension offers a concise and elegant way to work with lists in a single line of code.
This method also uses the for
loop but is considered a little faster than using it traditionally, like in the previous method.
Take the following code as an example.
import numpy as np
a = np.array([[1, 2, 3], [3, 4, 5], [7, 8, 9]])
print("\n".join(["".join(["{:4}".format(item) for item in row]) for row in a]))
Output:
1 2 3
3 4 5
7 8 9
Although the above code uses almost the same functions as in the previous method, it does so more efficiently and in one line. The other main difference is the format()
function used here to provide the necessary spacing between the string elements.
Another way of employing this method is shown below. Here we divide the code separately into individual lines and attempt to make the matrix resemble a table-like structure.
import numpy as np
a = np.array([[1, 2, 3], [3, 4, 5], [7, 8, 9]])
s = [[str(e) for e in row] for row in a]
lens = [max(map(len, col)) for col in zip(*s)]
fmt = "\t".join("{{:{}}}".format(x) for x in lens)
table = [fmt.format(*row) for row in s]
print("\n".join(table))
Output:
1 2 3
3 4 5
7 8 9
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn