How to Python SyntaxError: Non-Ascii Character xe2 in File
- What Are ASCII Codes
-
What Is the
SyntaxError: Non-ASCII character '\xe2'
in File in Python -
How to Fix the
SyntaxError: Non-ASCII character '\xe2'
in File in Python - Conclusion
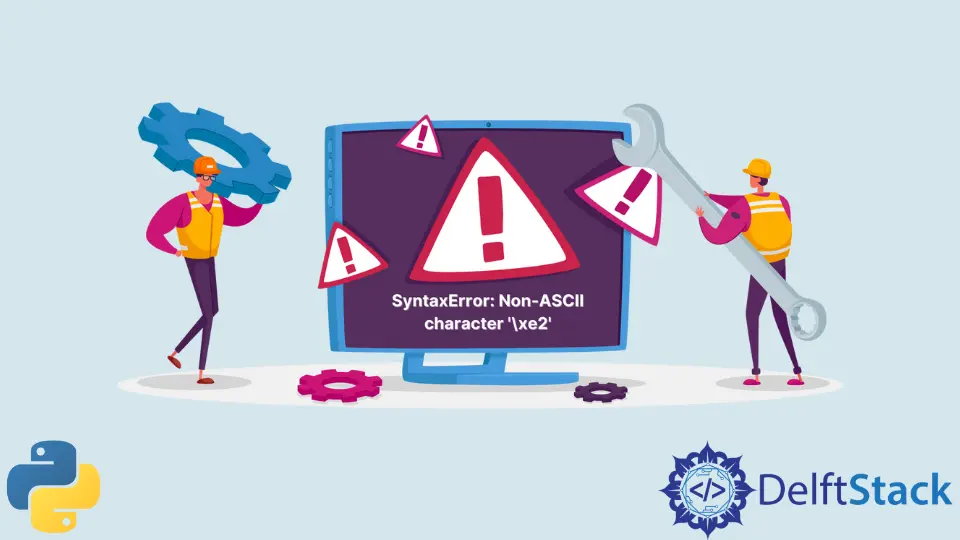
This error indicates that you are writing a Non-ASCII character in your code. At the compile time, the interpreter gets confused and throws the SyntaxError: Non-ASCII character '\xe2'
.
ASCII characters use the same encoding as the first 128 characters of the UTF-8, so ASCII text is compatible with UTF-8. First, you must understand the difference between ASCII and Non-ASCII characters.
What Are ASCII Codes
ASCII is the most popular character encoding format for text data on computers and the internet (American Standard Code for Information Interchange).
There are distinct values for 128 extra alphabetic, numeric, special, and control characters in ASCII-encoded data.
What Is the SyntaxError: Non-ASCII character '\xe2'
in File in Python
The core reason behind this error is that you are reading a character that isn’t recognized by your Python compiler.
For instance, let’s try the symbol £
, which the Python interpreter doesn’t recognize.
string = "£"
fp = open("test.txt", "w+")
fp.write("%s" % string)
print(string)
Output:
SyntaxError: Non-ASCII character '\xe2'
The symbol £
is not recognized by the interpreter, so it’s giving a SyntaxError: Non-ASCII character '\xe2'
.
How to Fix the SyntaxError: Non-ASCII character '\xe2'
in File in Python
Here we have included the statement #coding: utf-8
on the program’s top. This code will create a file test.txt
in which the £
value is stored and will print in the output as shown.
Code Example:
# coding: utf-8
string = "£"
fp = open("test.txt", "w+")
fp.write("%s" % string)
print(string)
Output:
£
Reading the PEP
that the error provides, it said the £
is not an ASCII character, despite your code’s attempts to utilize it. Use UTF-8 encoding if you can, and put # coding: utf-8
at the top of your program to get started.
To grow more complex, you may even declare encodings string by string in your code. However, you’ll need an encoder that supports it across the entire file if you’re trying to add the £
literal to your code.
You can also add the lines of code given below.
#!/usr/bin/env python
# -*- coding: utf-8 -*-
Conclusion
Non-ASCII is not allowed in Python as the interpreter will treat it as a SyntaxError
. Python can’t and shouldn’t attempt to determine what string a sequence of bytes represents outside the ASCII range.
To solve this error, you must add coding: utf-8
on the top of your program.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python