How to Calculate the Sum of Digits in Python
-
Use the
sum()
andstr()
Functions to Calculate the Sum of Digits in Python -
Use the
sum()
andmap()
Functions to Calculate the Sum of Digits in Python - Create a User-Defined Function to Calculate the Sum of Digits in Python
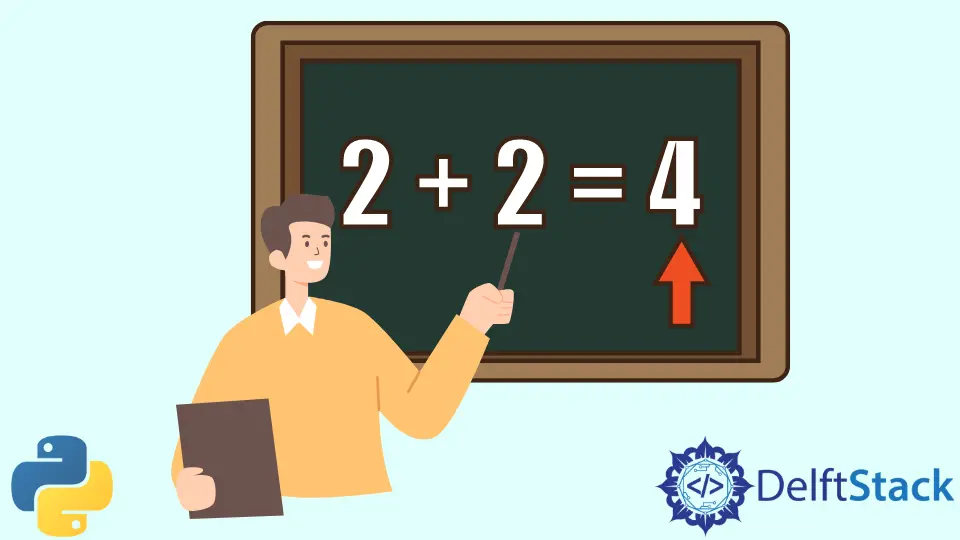
This tutorial will demonstrate calculating the sum of digits in Python. We will take an integer, find its digits and calculate its sum.
Use the sum()
and str()
Functions to Calculate the Sum of Digits in Python
The sum()
function calculates the sum of elements in the iterable. This method will convert an integer into a list of its digits and calculate the sum.
We can utilize the str()
function to convert the integer into a string. After this, we will create a list using list comprehension, where we iterate through the string and add the characters to the list.
Again, each character gets converted to an integer using the int()
function. The sum of this list is calculated and is the sum of digits.
Example:
x = 568
s = sum([int(i) for i in str(x)])
print(s)
Output:
19
Use the sum()
and map()
Functions to Calculate the Sum of Digits in Python
This method is a slightly faster approach than the previous method because we eliminate the use of the for
loop. We use the map()
function that can apply a given function to every element of the iterable.
We convert the integer into a string and then apply the int()
function to every string element using the map()
method. This collection of digits is again calculated using the sum()
function.
x = 568
s = sum(map(int, str(x)))
print(s)
Output:
19
Create a User-Defined Function to Calculate the Sum of Digits in Python
We can also create our functions to calculate the sum of digits in Python. For this, we will use the concept of repeated division.
A while
loop will be created that runs till the given number is greater than 0. In each iteration, we will find the digit from the right by dividing the number by 10 and storing its remainder.
The %
operator does this. Then we update the original number by dividing it by 10, and the loop runs again.
We update the sum of digits in every iteration. We use the //
operator to update the number, which performs floor division; it discards any decimal value.
We implement the above logic in the code below.
def sd(x):
s = 0
while x:
s += x % 10
x //= 10
return s
s = sd(568)
print(s)
Output:
19
To calculate the remainder and divide the number in one step, we can use the divmod()
function. In this function, we can specify the dividend and divisor, and it will return the result and remainder in a tuple.
Example:
def sd(x):
s = 0
while x:
x, r = divmod(x, 10)
s += r
return s
s = sd(568)
print(s)
Output:
19
Another way to improve the code and execution speed is not using augmented assignment statements.
See the code below.
def sd(x):
s = 0
while x:
s, x = s + x % 10, x // 10
return s
s = sd(568)
print(s)
Output:
19
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn