How to Calculate the Standard Deviation of a List in Python
-
Calculate the Standard Deviation of a List in Python Using
statistics.pstdev()
-
Calculate the Standard Deviation of a List in Python Using NumPy’s
std()
-
Calculate the Standard Deviation of a List in Python Using the
sum()
Function and List Comprehension -
Calculate the Standard Deviation of a List in Python Using the
math
Module - Conclusion
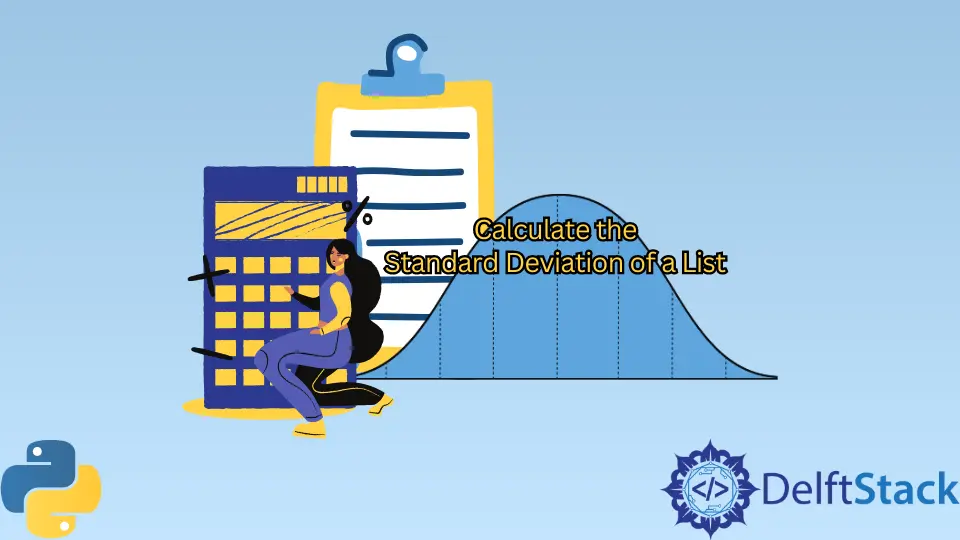
Statistical analysis is a fundamental aspect of data exploration and interpretation in Python. One key metric for understanding the distribution of data is the standard deviation.
Whether you’re working with a basic Python setup or employing specialized libraries, such as NumPy or the statistics module, calculating the standard deviation unveils valuable insights into the variability of your dataset.
This article will guide you through multiple methods, exploring both library-dependent and library-independent approaches to compute the standard deviation of a list in Python.
Calculate the Standard Deviation of a List in Python Using statistics.pstdev()
In Python, the statistics
module provides a convenient function called pstdev()
to calculate the standard deviation of a given list of numeric values.
The pstdev()
function in the statistics
module has the following syntax:
statistics.pstdev(data, mu=None)
Parameters:
data
: The input data for which you want to calculate the standard deviation.mu
: Optional parameter representing the mean of the data. If not specified, the mean is calculated internally.
Now, let’s proceed with a practical example to demonstrate the usage of pstdev()
.
Code Example: Standard Deviation of a List in Python Using statistics.pstdev()
import statistics
# Sample data
data = [2, 4, 4, 4, 5, 5, 7, 9]
# Calculate population standard deviation using pstdev()
std_deviation = statistics.pstdev(data)
# Display the result
print(f"Population Standard Deviation: {std_deviation}")
In this example, we import the statistics
module. We then define a sample dataset named data
. In our case, the dataset is [2, 4, 4, 4, 5, 5, 7, 9]
.
To calculate the population standard deviation, we use the pstdev()
function, passing our data as an argument. The result is stored in the variable std_deviation
.
Finally, we print the calculated population standard deviation using a formatted string.
Code Output:
In this example, the population standard deviation of the given dataset is approximately 2.0. This value represents the spread or dispersion of the data points from the mean.
You can apply the pstdev()
function to your datasets to analyze the variability within your numeric data.
Calculate the Standard Deviation of a List in Python Using NumPy’s std()
In addition to the statistics
module, the NumPy library also provides a powerful tool for statistical calculations, including standard deviation. The std()
function in NumPy can be employed to efficiently compute the standard deviation of a given list.
The std()
function in NumPy has the following syntax:
numpy.std(a, axis=None, dtype=None, ddof=0, keepdims=False)
Parameters:
a
: Input array for which you want to calculate the standard deviation.axis
: Optional parameter specifying the axis or axes along which the standard deviation is computed.dtype
: Optional parameter defining the data type used for computations.ddof
: Optional parameter representing the “degrees of freedom” correction in the calculation.keepdims
: Optional parameter indicating whether to keep the dimensions of the original array.
The std()
function uses the formula for population standard deviation, similar to the pstdev()
function in the statistics
module.
Now, let’s proceed with a practical example to demonstrate the usage of std()
from NumPy.
Code Example: Standard Deviation of a List in Python Using NumPy’s std()
import numpy as np
# Sample data
data = [2, 4, 4, 4, 5, 5, 7, 9]
# Calculate standard deviation using np.std()
std_deviation = np.std(data)
# Display the result
print(f"Standard Deviation: {std_deviation}")
In this example, we import the NumPy library as np
. We define a sample dataset named data
.
To calculate the standard deviation, we use the np.std()
function, passing our data as an argument. The result is stored in the variable std_deviation
.
Finally, we print the calculated standard deviation using a formatted string.
Code Output:
In this example, the standard deviation of the given dataset is also 2.0. This value represents the spread or dispersion of the data points from the mean.
Using NumPy’s std()
function allows for efficient and convenient calculation of standard deviation, especially when working with large datasets or multidimensional arrays.
Calculate the Standard Deviation of a List in Python Using the sum()
Function and List Comprehension
While libraries like statistics
and NumPy provide specialized functions for standard deviation, you can also calculate it using basic Python constructs. In this approach, we’ll utilize the sum()
function and list comprehension to compute the standard deviation of a given list.
The standard deviation formula involves several steps, including calculating the mean and finding the squared differences between each data point and the mean. Here, we’ll use list comprehension to efficiently perform these operations.
The steps involved are as follows:
-
Find the mean of the dataset by summing all elements and dividing by the total number of elements.
-
Use list comprehension to generate a new list containing the squared differences between each data point and the mean.
-
Find the mean of the squared differences calculated in step 2.
-
Take the square root of the mean of squared differences to obtain the standard deviation.
Now, let’s proceed with a practical example to demonstrate the usage of this method.
Code Example: Standard Deviation of a List in Python Using the sum()
Function and List Comprehension
# Sample data
data = [2, 4, 4, 4, 5, 5, 7, 9]
# Calculate mean
mean = sum(data) / len(data)
# Calculate squared differences and mean of squared differences
squared_diff = [(x - mean) ** 2 for x in data]
mean_squared_diff = sum(squared_diff) / len(squared_diff)
# Calculate the standard deviation
std_deviation = mean_squared_diff**0.5
# Display the result
print(f"Standard Deviation: {std_deviation}")
In this example, we start by defining a sample dataset named data
. We then calculate the mean by summing all elements and dividing by the length of the dataset (mean = sum(data) / len(data)
).
Using list comprehension, we create a new list, squared_diff
, containing the squared differences between each data point and the mean. We find the mean of these squared differences and finally calculate the standard deviation by taking the square root.
The result is printed using a formatted string.
Code Output:
Here, the standard deviation of the given dataset is 2.0, matching the result obtained using the NumPy method. This approach showcases how basic Python constructs can be utilized to perform statistical calculations, providing insight into the underlying operations involved in standard deviation calculations.
Calculate the Standard Deviation of a List in Python Using the math
Module
In addition to specialized libraries like statistics
and NumPy, Python’s built-in math
module offers functionality for calculating the standard deviation. While not as feature-rich as some external libraries, the math
module provides the sqrt()
function, which can be employed to calculate the square root, an essential step in standard deviation calculation.
To calculate the standard deviation using the math
module, we follow similar steps as in the previous approach. The key difference lies in using the sqrt()
function from the math
module to obtain the square root of the mean of squared differences.
The steps are as follows:
-
Find the mean of the dataset by summing all elements and dividing by the total number of elements.
-
Use list comprehension to create a new list containing the squared differences between each data point and the mean.
-
Find the mean of the squared differences calculated in step 2.
-
Utilize the
sqrt()
function from themath
module to obtain the square root of the mean of squared differences.
Now, let’s proceed with a practical example to demonstrate the usage of this method.
Code Example: Standard Deviation of a List in Python Using the math
Module
import math
# Sample data
data = [2, 4, 4, 4, 5, 5, 7, 9]
# Calculate mean
mean = sum(data) / len(data)
# Calculate squared differences and mean of squared differences
squared_diff = [(x - mean) ** 2 for x in data]
mean_squared_diff = sum(squared_diff) / len(squared_diff)
# Calculate standard deviation using math.sqrt()
std_deviation = math.sqrt(mean_squared_diff)
# Display the result
print(f"Standard Deviation: {std_deviation}")
In this example, we begin by importing the math
module. We define a sample dataset named data
and calculate the mean using mean = sum(data) / len(data)
.
List comprehension is then employed to create squared_diff
, a list of squared differences. We find the mean of these squared differences and, instead of using the exponentiation operator (**
), use the math.sqrt()
function to calculate the square root.
The result is printed using a formatted string.
Code Output:
In this example, the standard deviation of the given dataset is 2.0, consistent with the results obtained using the NumPy and basic Python constructs methods. The math
module provides a simple yet effective means to perform standard deviation calculations in scenarios where more specialized libraries are not necessary.
Conclusion
Calculating the standard deviation of a list in Python is a fundamental operation in statistical analysis, offering insights into the variability of data points. Whether utilizing the built-in statistics
module, the powerful NumPy library, or employing basic mathematical operations without external libraries, Python provides versatile options for standard deviation computation.
Each method discussed—the statistics
module, NumPy, manual calculations, and math
module—offers unique advantages, allowing you to choose an approach that aligns with your specific needs and preferences. By mastering these techniques, you can enhance your data analysis capabilities and gain a deeper understanding of the distribution and spread of their datasets.
Whether you prefer the simplicity of built-in functions or the flexibility of manual calculations, Python provides the tools for accurate and insightful standard deviation calculations.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedInRelated Article - Python Statistics
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python