在 Python 中計算列表的標準差
-
在 Python 中使用
statistics
模組的pstdev()
函式計算列表的標準偏差 -
在 Python 中使用
NumPy
庫的std()
函式計算列表的標準偏差 -
在 Python 中使用
sum()
函式和列表推導式計算列表的標準偏差
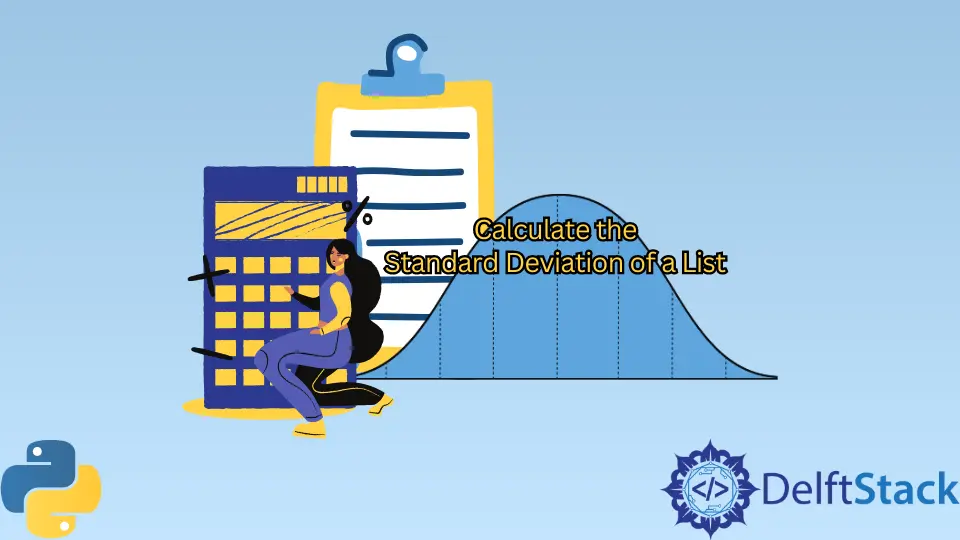
在 Python 中,有很多統計操作正在執行。這些操作之一是計算給定資料的標準偏差。資料的標準偏差告訴我們資料偏離平均值的程度。在數學上,標準偏差等於方差的平方根。
本教程將演示如何在 Python 中計算列表的標準偏差。
在 Python 中使用 statistics
模組的 pstdev()
函式計算列表的標準偏差
pstdev()
函式是 Python 的 statistics
模組下的命令之一。statistics
模組提供了對 Python 中的數值資料執行統計操作的函式,如均值、中位數和標準差。
statistics
模組的 pstdev()
函式幫助使用者計算整個總體的標準偏差。
import statistics
list = [12, 24, 36, 48, 60]
print("List : " + str(list))
st_dev = statistics.pstdev(list)
print("Standard deviation of the given list: " + str(st_dev))
輸出:
List : [12, 24, 36, 48, 60]
Standard deviation of the given list: 16.97056274847714
在上面的示例中,str()
函式將整個列表及其標準偏差轉換為字串,因為它只能與字串連線。
在 Python 中使用 NumPy
庫的 std()
函式計算列表的標準偏差
NumPy
代表 Numerical Python
是 Python 中廣泛使用的庫。這個庫有助於處理陣列、矩陣、線性代數和傅立葉變換。
NumPy
庫的 std()
函式用於計算給定陣列(列表)中元素的標準偏差。參考下面的示例。
import numpy as np
list = [12, 24, 36, 48, 60]
print("List : " + str(list))
st_dev = np.std(list)
print("Standard deviation of the given list: " + str(st_dev))
輸出:
List : [12, 24, 36, 48, 60]
Standard deviation of the given list: 16.97056274847714
在 Python 中使用 sum()
函式和列表推導式計算列表的標準偏差
顧名思義,sum()
函式提供可迭代物件(如列表或元組)的所有元素的總和。列表推導式是一種從現有列表中存在的元素建立列表的方法。
sum()
函式和列表推導式可以幫助計算列表的標準偏差。這是一個示例程式碼。
import math
list = [12, 24, 36, 48, 60]
print("List : " + str(list))
mean = sum(list) / len(list)
var = sum((l - mean) ** 2 for l in list) / len(list)
st_dev = math.sqrt(var)
print("Standard deviation of the given list: " + str(st_dev))
輸出:
List : [12, 24, 36, 48, 60]
Standard deviation of the given list: 16.97056274847714
在上面的示例中,匯入了 math
模組。它提供了 sqrt()
函式來計算給定值的平方根。另外,請注意還使用了函式 len()
。此函式有助於提供給定列表的長度,例如,列表中的元素數。
該方法基於標準差的數學公式。首先,我們計算方差,然後求其平方根以找到標準偏差。
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn