How to Send Function in Python Generators
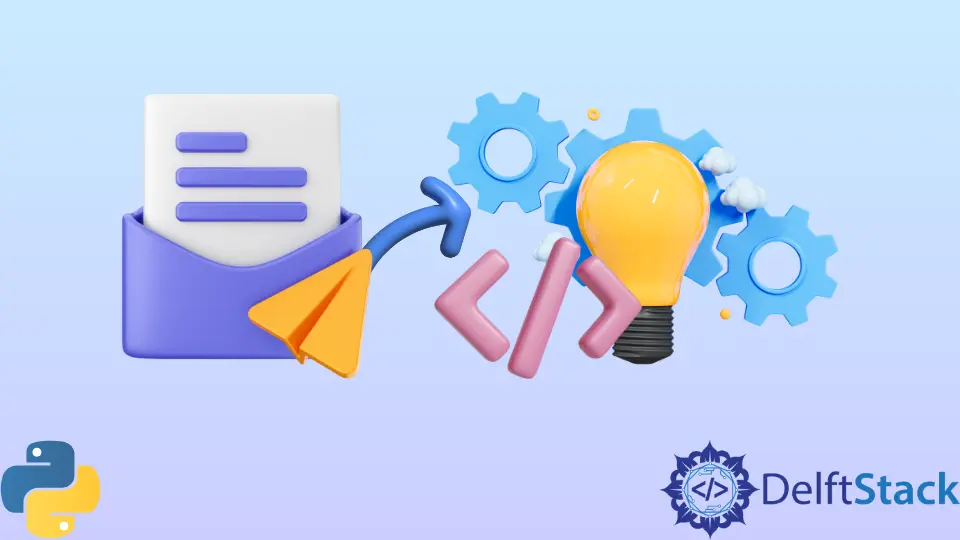
This tutorial will teach how to use the send()
function of the generator in Python.
Overview of Generators
You can make a function that acts like an iterator and can be used in a for
loop via Python generator functions. For example, we’ll compare various implementations of a method called first_n()
that represents the first n
non-negative integers.
Since n
is a huge number, we’ll assume that each integer requires a lot of storage space—say, let’s 10
megabytes each—to make this comparison.
Remember that they do not occupy much space in real life unless they are huge integers. So, for instance, you can use 128 bytes to represent a 309-digit integer.
Let us consider the following example.
def first_n(n):
num, nums = 0, []
while num < n:
nums.append(num)
num += 1
return nums
sum_of_first_n = sum(first_n(1000000))
The above code makes a summation function that sums values up to the first n
numbers. The only problem is that it builds up a lot of memory.
Remember, we cannot afford to keep up to 10
megabyte integers in our memory. So to solve it, we make use of generators in Python.
A generator helps in creating iterators. We can understand it via the following code block.
def first_n(n):
num, nums = 0, []
while num < n:
yield num
num += 1
return nums
print(sum(first_n(1000000)))
Output:
499999500000
The above code fence uses the memory use case feature of the iterator execution.
Use send()
in Python Generators
The send()
method for generator-iterators is useful for generators. Since Python version 2.5, this function, specified in PEP 342
, has been accessible.
The generator is restarted with the send()
method, which also sends a value that will be used to start the subsequent yield. Finally, the process (method) returns the new value yielded by the generator.
The send()
method is similar to using next()
when there is no value. None
is another possible value for this procedure. In both circumstances, the generator will forward its operation to the first return
statement.
Let us explore the send()
function in the following code.
def numberGenerator(n):
number = yield
while number < n:
yield number
number += 1
g = numberGenerator(10)
next(g)
print(g.send(5))
Output:
5
As we can see in the code block above, in the last line, we send the value 5
within our g
variable, ensuring that the generator considers the last input as 5
.
The send()
function sends the last acceptable value to the generator variable that it can use as the last input. Thus, we have understood how generators work and the significance of a generator’s send()
function.
Related Article - Python Function
- How to Exit a Function in Python
- Optional Arguments in Python
- How to Fit a Step Function in Python
- Built-In Identity Function in Python
- Arguments in the main() Function in Python
- Python Functools Partial Function