How to Check if the Generator Is Empty in Python
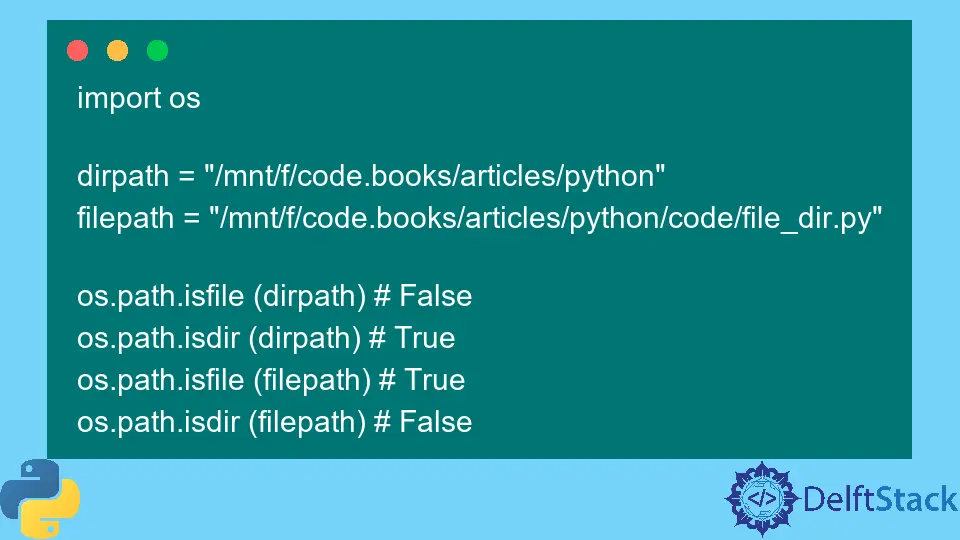
This article discusses how to check if a generator is empty in Python.
Check if the Generator Is Empty in Python
Checking if a generator is empty in Python refers to a path getting checked to ensure that it is empty. For this, the relevant path must be identified beforehand as either a directory or a file, which allows us to select the required approach to check to be used effortlessly.
The following snippet shows the involvement of two placeholder variables named filepath
and dirpath
. These variables are used for the identification of a local file and directory:
dirpath = "/mnt/f/code.books/articles/python"
filepath = "/mnt/f/code.books/articles/python/code/file_dir.py"
The above placeholder variables can work with the os
module in Python that acts as a standard package. It can be used with objects, functions, and constants that work with the relevant operating system.
The os
module can also be denoted as os.path
and involves isdir()
and isfile()
that work as functions. These functions enable the users to differentiate between the given directory and any file.
The return value in the working of both functions is a Boolean value.
Consider the following example:
import os
dirpath = "/mnt/f/code.books/articles/python"
filepath = "/mnt/f/code.books/articles/python/code/file_dir.py"
os.path.isfile(dirpath) # False
os.path.isdir(dirpath) # True
os.path.isfile(filepath) # True
os.path.isdir(filepath) # False
The isdir()
and isfile()
functions are denoted as os.path.isdir
and os.path.isfile
, respectively, in this example. The return value in the working of both functions is a Boolean value.
It means that the output of the implementation of these functions should return as either True
or False
. The return values of True
and False
show if the relevant path is either a file or directory based on its respective function.
After this confirmation, another module named pathlib
can be used in and after the Python 3.4 version. With this module, an object-oriented interface has enabled users to work with their file systems easily.
This module ensures simplifications within the implementation compared to the os.path
module. It has a Path
class involved in its work, with a path accepted as an argument.
After this, a Path
object is returned by that path so the users can easily chain or query it with attributes and methods.
Take a look at the following code snippet:
from pathlib import Path
dirpath = "/mnt/f/code.books/articles/python"
filepath = "/mnt/f/code.books/articles/python/code/file_dir.py"
Path(dirpath).is_file() # False
Path(dirpath).is_dir() # True
Path(filepath).is_file() # True
Path(dirpath).is_file() # False
In this snippet, the Path
object is checked to ensure that it is either a directory or a file with a Boolean value as the return value. The dirpath
and filepath
variables are also involved with the Path
object associated with the is_file()
and is_dir()
methods.
Their implementation will return output as either True
or False
, showing either a directory or a file as the object.
The next step is to check if a generator is empty and can involve an empty path or file. The path or file can also be named a zero-byte file and can be of different types with no content or data.
Remember that files containing metadata with no data do not fall under the umbrella of empty files. This can even involve music files that contain an author.
Empty files can be easily created on platforms like macOS and LINUX. Here is a snippet of how that can be done:
$ touch emptyfile
The following snippet shows how to do that on Windows:
$ type nul > emptyfile
After doing this, we have to define variables with which a non-empty file or an empty file can point to an empty file. That empty file is likely zero bytes, whereas the non-empty file is a byte in size.
The following is an example of what that can look like:
emptyfile = "/mnt/f/code.books/articles/python/emptyfile"
nonemptyfile = "/mnt/f/code.books/articles/python/onebytefile"
With this, an optional parameter can be used for the next()
function, with which users can ensure that the generator is empty.
Here is a code snippet for that:
_exhausted = object()
if next(some_generator, _exhausted) is _exhausted:
print("empty generator")
In this snippet, the empty generator is denoted by an exhausted iterator and an object’s usage. After this, an if
condition is placed within the implementation, which checks for an exhausted generator with some generator value.
In the case of an empty generator, the condition is fulfilled, and the implementation returns an empty generator as its output.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn