Python Generator Comprehension
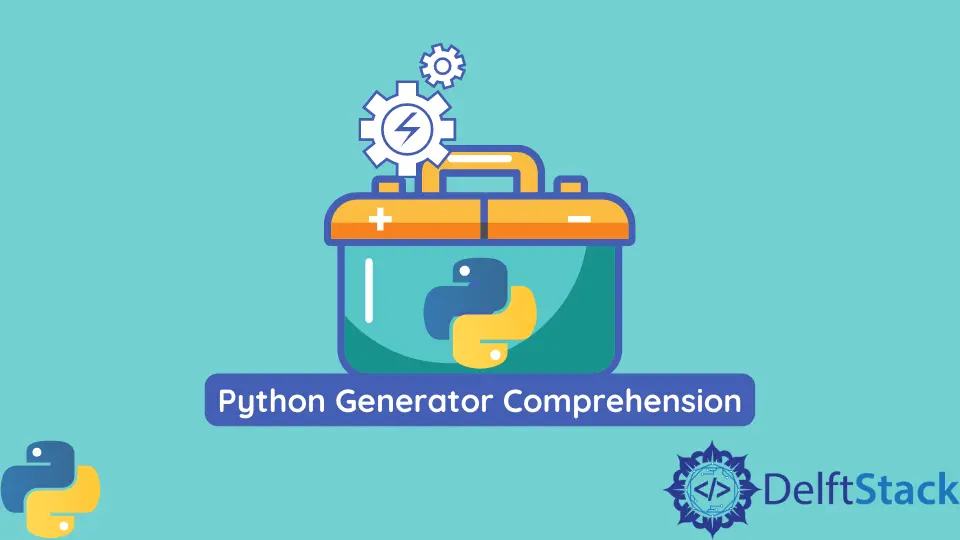
Generator comprehension is a smart and efficient way of creating generators in python. It is a single-line specification of defining a generator which is essential to understand that syntax to code efficiently.
In this article, we will learn python’s generators and generator comprehensions along with examples.
Generators in Python
Generators in Python are functions that return an iterable or traversal object used to create iterators that traverse the items at once. The generator is a simple and easy way of creating iterators with the predefined iter()
and next()
functions to keep track of the internal states, and it also takes care of the StopInteration
exception.
Whereas there is a lot of complexity in creating iteration in Python, you have to define and implement the iter()
and next()
functions. It’s a lengthy process to do so, but thanks to generators.
Let’s see an example of generators in Python.
# A simple generator function
def my_gen():
n = 1
print(f"This is line number {n}")
# generator function always contains the 'yield' Keyword
yield n
n += 1
print(f"This is line number {n}")
yield n
n += 1
print(f"This is line number {n}")
yield n
print(my_gen())
Output:
<generator object my_gen at 0x00000150EA43E448>
We used the yield
in the above function to turn the values into a generator. When we printed the my_gen()
function, we got the object of the function that can be used to iterate over the generator.
Let’s see an example to iterate over the generator.
def even_nums():
# create generator
for i in range(11):
if i % 2 == 0:
yield i
print("This is the generator object of the generator function:", even_nums())
# iterate over the generator function
print("\nEven number from 0 to 11")
for i in even_nums():
print(i, end=", ")
Output:
This is the generator object of the generator function: <generator object even_nums at 0x05ACC2D0>
Even number from 0 to 11
0, 2, 4, 6, 8, 10,
Python Generator Comprehension
Generator comprehension is similar to the list comprehensions in Python; however, parentheses and square brackets differentiate them. We can easily create a simple generator using generator comprehension in Python that works similarly to the lambda functions, which create anonymous functions.
The difference between generator comprehension and list comprehension is that generator comprehension creates one item simultaneously, whereas list comprehension creates the whole list simultaneously. The generator comprehension is much faster and memory efficient.
Let’s see an example of generator comprehension in Python.
# generator comprehension in python
# take the square of each number from 1-6 and store it in 'gen'
gen = (x ** 2 for x in range(1, 6))
print(gen) # return --> generator object
# iterate over the generator 'gen'
for i in gen:
print(i, end=", ")
Output:
<generator object <genexpr> at 0x00000150EA43EC48>
1, 4, 9, 16, 25,
In the above code example, the generator expression has produced the required results on demand but not immediately. It returned the object of the generator that we later used to iterate over the generator using a for
loop in one line.
You can use the next()
function to print each value of the generator sequentially, as the generator takes care of the sequence and state of the values.
# generator comprehension in python
# take the square of each number from 1-6 and store it in 'gen'
gen = (x ** 2 for x in range(1, 6))
# next()
print(next(gen))
print(next(gen))
print(next(gen))
print(next(gen))
print(next(gen))
Output:
1
4
9
16
25
As you can see, we are getting the on-demand value using the next()
, and it is taking care of the sequence of values as I m getting the next value after each next()
function.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn