One-Line for Loop in Python
-
Traditional
for
Loops in Python -
Introduction to One-Line
for
Loops -
List Comprehensions: The Foundation of One-Line
for
Loops - Using Conditional Statements in Comprehensions
- Nested Comprehensions
- Dictionary and Set Comprehensions
- Conclusion
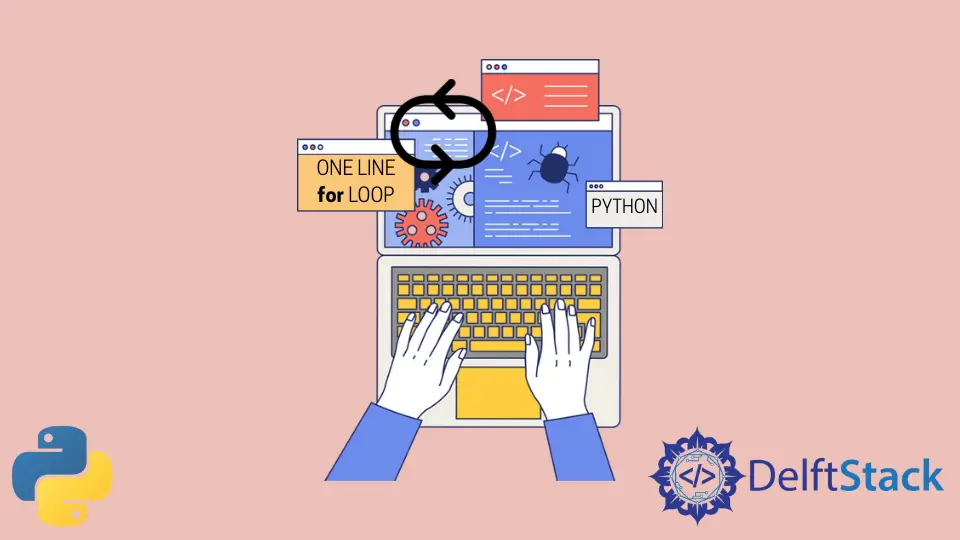
Python’s simplicity and versatility make it one of the most popular programming languages. One of its powerful features is the ability to write concise and readable code
, reducing the number of lines needed for common operations.
Among these techniques, one-line for
loops (also known as comprehensions
) stand out as an elegant way to iterate over data structures while maintaining clarity and efficiency. Whether you’re processing lists, dictionaries, sets, or even generating complex data transformations, one-line for
loops help improve readability, execution speed, and performance.
This article will take a deep dive into one-line for
loops in Python, exploring their syntax, benefits, and real-world applications. We’ll start with traditional for
loops and progressively move towards comprehensions, conditional statements, and nested loops.
By the end of this guide, you’ll be able to write clean, efficient, and optimized Python code
using one-line for
loops.
Traditional for
Loops in Python
Before diving into one-line for
loops, let’s first understand how traditional for
loops work in Python. A standard ``for loop
iterates over a sequence (such as a list, tuple, or range) and executes a block of code for each item in the sequence.
Example: Printing a List of Numbers
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
Output:
1
2
3
4
5
Here, Python iterates through each item in numbers
, executing print(num)
on every iteration.
Example: Squaring Numbers in a List
numbers = [1, 2, 3, 4, 5]
squares = []
for num in numbers:
squares.append(num ` 2)
print(squares)
Output:
[1, 4, 9, 16, 25]
While traditional for
loops are easy to read and understand, they can become lengthy and take up multiple lines
when performing simple operations. This is where one-line for
loops come in handy, making the code more concise while maintaining readability.
Introduction to One-Line for
Loops
A one-line for loop
, also known as list comprehension
, allows us to execute the same logic in a single line, eliminating unnecessary indentation and improving readability. The general syntax follows this structure:
[expression for item in iterable]
Example: Squaring Numbers in One Line
Instead of using a traditional for
loop, we can use a list comprehension
to achieve the same result more efficiently:
numbers = [1, 2, 3, 4, 5]
squares = [num ` 2 for num in numbers]
print(squares)
Output:
[1, 4, 9, 16, 25]
This approach offers several benefits:
Concise & Readable
: The entire operation is performed in a single line.Faster Execution
: List comprehensions are optimized internally for better performance compared to traditionalfor
loops.Easy to Modify
: Adjusting the operation is simpler within a single expression.
Example: Converting a List of Strings to Uppercase
words = ["hello", "world", "python"]
uppercase_words = [word.upper() for word in words]
print(uppercase_words)
Output:
['HELLO', 'WORLD', 'PYTHON']
List comprehensions can be used for a wide variety of data transformations
, making them a fundamental part of modern Python programming.
List Comprehensions: The Foundation of One-Line for
Loops
List comprehensions allow you to create new lists from existing iterables in a single, compact statement, while another common way to transform lists in Python is by using the map()
function. The basic syntax follows this pattern:
[expression for item in iterable]
expression
: The operation performed on each item.item
: The current element in the iterable.iterable
: The data structure being looped over (e.g., list, tuple, set, dictionary).
Example: Doubling Numbers in a List
numbers = [1, 2, 3, 4, 5]
doubled = [num * 2 for num in numbers]
print(doubled)
Output:
[2, 4, 6, 8, 10]
Example: Extracting First Letter of Words
words = ["apple", "banana", "cherry"]
first_letters = [word[0] for word in words]
print(first_letters)
Output:
['a', 'b', 'c']
Using Conditional Statements in Comprehensions
List comprehensions can include conditional statements to filter elements based on specific criteria. This allows for cleaner, more efficient filtering within the same line of code. Another approach to achieve filtering in a functional style is by using the filter()
function with Lambda.
Example: Filtering Even Numbers
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers)
Output:
[2, 4, 6]
In this example, the if
condition ensures that only numbers divisible by 2 are added to the new list. This approach is more efficient than using a traditional loop with an if
statement.
Example: Extracting Words That Contain a Specific Letter
words = ["apple", "banana", "cherry", "date"]
words_with_a = [word for word in words if "a" in word]
print(words_with_a)
Output:
['apple', 'banana', 'date']
This method is useful for filtering words based on specific conditions, such as containing certain characters or meeting length requirements. However, when working with sorting operations, you might find Lambda Functions in Sort useful for applying quick inline functions.
Nested Comprehensions
You can nest comprehensions to handle multi-dimensional structures like lists of lists. This is particularly useful when working with matrices or multi-level data structures. If you want to explore more on writing nested loops in a single line, check out our guide on Nested for
Loops in One Line in Python.
Example: Flattening a 2D List
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = [num for row in matrix for num in row]
print(flattened)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Here, the nested comprehension iterates first over each row
and then over each num
in that row, effectively flattening the matrix into a one-dimensional list.
Example: Creating a Multiplication Table
table = [[i * j for j in range(1, 6)] for i in range(1, 6)]
for row in table:
print(row)
Output:
[1, 2, 3, 4, 5]
[2, 4, 6, 8, 10]
[3, 6, 9, 12, 15]
[4, 8, 12, 16, 20]
[5, 10, 15, 20, 25]
Dictionary and Set Comprehensions
Just like lists, Python allows one-line dictionary and set comprehensions to create collections efficiently. If you’re looking to remove elements dynamically from a dictionary, check out How to Remove an Element from a Python Dictionary.
Example: Creating a Dictionary of Squares
numbers = [1, 2, 3, 4]
square_dict = {num: num ** 2 for num in numbers}
print(square_dict)
Output:
{1: 1, 2: 4, 3: 9, 4: 16}
Example: Creating a Set of Unique Word Lengths
words = ["apple", "banana", "cherry"]
unique_lengths = {len(word) for word in words}
print(unique_lengths)
Output:
{5, 6}
Conclusion
In this comprehensive guide, we’ve explored the power of one-line for
loops in Python, starting from the basics of traditional loops and progressing through more advanced techniques like list comprehensions, conditional filtering, nested comprehensions, and dictionary and set comprehensions.
We saw how list comprehensions provide a more concise and readable way to transform data, how conditions within comprehensions enable efficient filtering, and how nested comprehensions allow for processing multi-dimensional data structures seamlessly. Additionally, we explored dictionary and set comprehensions, which offer powerful ways to generate key-value mappings and unique collections efficiently.