How to Skip Iterations in a Python Loop
- Using the continue Statement
- Handling Exceptions with Try-Except
- Using Conditional Statements to Control Flow
- Conclusion
- FAQ
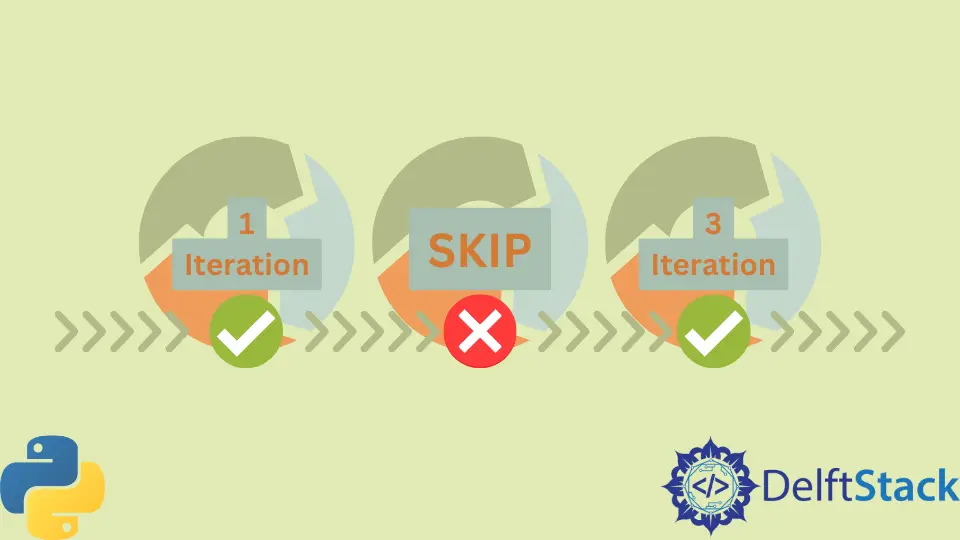
In the world of programming, loops are essential for executing repetitive tasks efficiently. However, there are times when you may want to skip an iteration based on a certain condition, such as an exception. In Python, this is straightforward thanks to the continue
statement. By mastering this technique, you can enhance your code’s performance and readability.
This article will guide you through various methods to skip iterations in a Python loop, helping you to handle exceptions gracefully. Whether you’re a beginner or an experienced developer, understanding how to manage loop iterations effectively is crucial for writing clean and efficient code. Let’s dive into the methods that will empower you to control your loops better.
Using the continue Statement
The continue
statement is the most common way to skip an iteration in Python. When the continue
statement is executed, the loop immediately jumps to the next iteration, bypassing any code that follows it within the loop. This is particularly useful when you want to skip over certain values or handle exceptions without breaking the entire loop.
Here’s a simple example that demonstrates how to use the continue
statement:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for number in numbers:
if number % 2 == 0:
continue
print(number)
Output:
1
3
5
7
9
In this example, we have a list of numbers from 1 to 10. The loop iterates through each number, checking if it is even. If the number is even, the continue
statement is executed, which skips the print
function for that iteration. As a result, only the odd numbers are printed. This method is efficient as it allows you to avoid unnecessary computations for specific conditions.
Handling Exceptions with Try-Except
Another effective way to skip iterations is by using the try-except
block. This method allows you to handle exceptions gracefully, ensuring that if an error occurs, the loop can continue with the next iteration instead of terminating. This is particularly useful when working with data that may contain unexpected values.
Here’s an example demonstrating this approach:
data = [10, 20, 'a', 30, None, 40]
for item in data:
try:
result = 100 / item
print(result)
except (TypeError, ZeroDivisionError):
continue
Output:
10.0
5.0
3.3333333333333335
2.5
In this code, we have a list that contains integers and some values that could cause exceptions. The loop attempts to divide 100 by each item in the list. If the item is a string or None
, a TypeError
will be raised; if it’s zero, a ZeroDivisionError
will occur. The except
block catches these exceptions, and the continue
statement ensures that the loop skips to the next item without crashing. This method is particularly robust for processing data that may not be clean or predictable.
Using Conditional Statements to Control Flow
In some scenarios, you may want to implement more complex conditions to determine when to skip an iteration. By combining conditional statements with the continue
statement, you can create tailored logic that fits your specific needs. This approach allows for greater flexibility in managing loop behavior.
Consider the following example:
numbers = range(1, 21)
for number in numbers:
if number < 10:
continue
if number % 2 == 0:
print(f"{number} is even")
else:
print(f"{number} is odd")
Output:
10 is even
11 is odd
12 is even
13 is odd
14 is even
15 is odd
16 is even
17 is odd
18 is even
19 is odd
20 is even
In this code snippet, the loop iterates through numbers from 1 to 20. The first conditional statement checks if the number is less than 10. If it is, the continue
statement is executed, skipping the rest of the loop for that iteration. For numbers 10 and above, the code checks if the number is even or odd and prints the appropriate message. This method provides a clear structure and allows for more nuanced control over which iterations to skip based on multiple conditions.
Conclusion
Skipping iterations in a Python loop is a powerful technique that enhances your code’s efficiency and clarity. Whether you use the continue
statement, handle exceptions with try-except
, or implement complex conditional logic, mastering these methods will allow you to write cleaner and more effective code. By understanding how to manage loop iterations, you can ensure that your programs run smoothly, even when faced with unexpected data or conditions. Embrace these techniques, and watch your Python programming skills soar.
FAQ
-
What is the purpose of the continue statement in Python?
Thecontinue
statement allows you to skip the current iteration of a loop and proceed to the next iteration. -
How can I handle exceptions while iterating through a list in Python?
You can use atry-except
block to catch exceptions and use thecontinue
statement to skip the iteration that caused the error. -
Can I use multiple conditions to skip iterations in a loop?
Yes, you can combine multiple conditional statements with thecontinue
statement to create complex logic for when to skip iterations. -
What happens if I don’t handle exceptions in a loop?
If an exception occurs and is not handled, it will terminate the loop and raise an error, potentially crashing your program. -
Is using the continue statement considered good practice?
Yes, using thecontinue
statement can improve code readability and efficiency by allowing you to skip unnecessary iterations without cluttering your code with additional nested conditions.