How to Get The Next Item in Python for Loop
- Loops in Python
-
the
for
Loop in Python -
Use
for
Loop to Get the Next Item in the Python List, Skipping the First Item - Conclusion
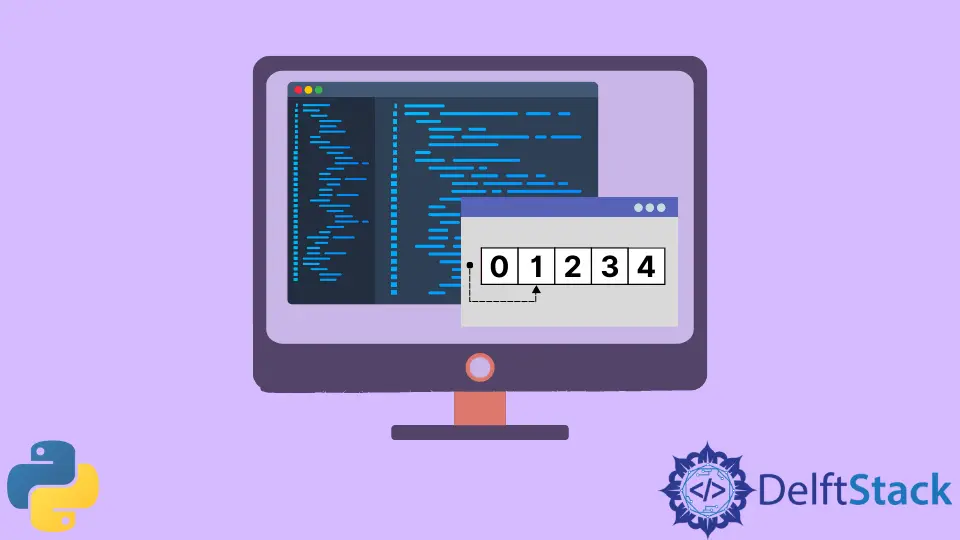
In this article, we will discuss the for
loop and how to use it in Python to skip the first element of a list.
Loops in Python
In programming, a loop is a set of instructions that repeatedly executes itself until a specific condition is fulfilled. Loops help to convert a hundred lines of code into a few. There are three kinds of loops in Python.
for
loopwhile
loopnested
loop
the for
Loop in Python
Commonly for
loop is used to iterate over sequences like lists, types, dictionaries, sets, or perhaps strings.
Syntax:
for item_ in sequence:
statements
Let me explain about above syntax.
- Firstly, the
for
is the keyword in Python which starts the loop. - Then
item
is the iterator variable that, on each repetition, gets the items’ value included in the sequence. - Next, the keyword in syntax is
in
, which represents the iterator variable used to loop over the sequence’s elements. - Finally, there is the
sequence
variable, which might be a list, a tuple, or any other sort of iterator.
Until the last item in the array is attained, the loop never ends. The for
loop statements have a variety of purposes and are indented to separate them from the rest of the code.
Use for
Loop to Get the Next Item in the Python List, Skipping the First Item
In this phase, we will discuss how to print elements by skipping the first element in a list using the for
loop.
Firstly, let’s create an integer list.
list = [1, 2, 3, 4, 5]
print(list)
Before moving on to the skipping first element, let’s see what the typical output of the above code will be.
[1, 2, 3, 4, 5]
Let’s see how to code to skip the first element of the list by using the for
loop.
list = [1, 2, 3, 4, 5]
for index, Element in enumerate(list):
if index < len(list) and index - 1 >= 0:
next_element = list[index]
print(next_element)
Let me explain the code.
-
Firstly, as previously mentioned,
for
is the keyword in Python which starts the loop. Next, there areindex
andElement
, which are built-in.The
index
is used to identify the list’s order, starting from “0”. TheElement
represents values inside of the list. Finally the end of the first code line, there is anenumerate
function.
Keeping track of iterations is necessary when working with iterators, and the built-in function `enumerate()` helps with this in Python.
-
The second line has an
if
condition, used to execute the body when the condition is true. Suppose any conditions may be false; the entireif
statement is not executed.There are two conditions. The first condition is used to check whether the length of the list is exceeded or not while the loop is iterating, and the condition is true when the current index of the list is less than the value of the number of elements.
The second condition is to prove that the index cannot be a negative number because there are no lower number indices. So to execute the
if
condition, both conditions must be correct. -
Then, a variable named
next_element
equals the element in the list corresponding to the index. -
Finally, we can skip the first element and print the list.
Output:
2
3
4
5
As you can see, number “1” has skipped from the list, and the list started from number “2” as we wanted.
Let’s see another example using a string list. Firstly, let’s create a string list named fruits
.
fruits = ["apple", "banana", "cherry", "orange", "grapes"]
print(fruits)
Let’s see the typical output of the above code.
['apple', 'banana', 'cherry', 'orange', 'grapes']
Now let’s see how to code to skip the first element (“apple”) in the list using the for
loop.
fruits = ["apple", "banana", "cherry", "orange", "grapes"]
for index, Element in enumerate(fruits):
if index < len(fruits) and index - 1 >= 0:
next_element = str(fruits[index])
print(next_element)
Here, as you can see, there is the str()
method, a built-in function used to return the string version of the given object.
Output:
banana
cherry
orange
grapes
As you can see, all other elements are printed without the first element in the string list.
Conclusion
This article explains what Python loops are and provides an overview of the for
loop with examples. Then we discussed how to print a list of integers and a list of strings by using a for
loop and skipping the first element of the list.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.