Nested for Loop in One Line in Python
-
Nested
for
Loop in One Line Using List Comprehension in Python -
Nested
for
Loop in One Line Using theexec()
Function in Python - Conclusion
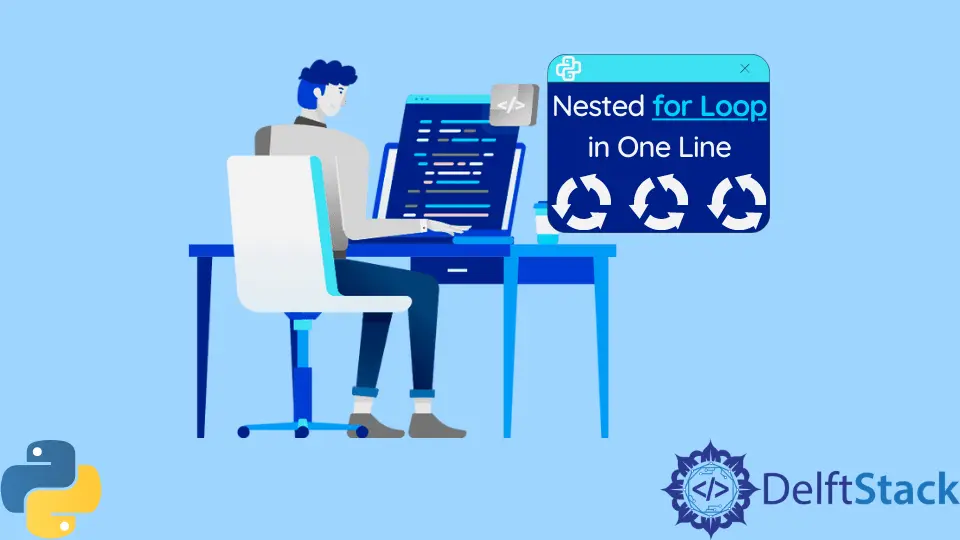
[The for
loop]({{relref “/HowTo/Python/one line for loop python.en.md”}}) is one of the most commonly used loops to iterate items from a list. In Python, we write the for
loop in one line, but how can we write it in one line when we have to use another loop inside it?
This tutorial will discuss the ways that can be used to write a nested for
loop in just one line.
Nested for
Loop in One Line Using List Comprehension in Python
One way of writing nested for
loop in one line is utilizing the list comprehension. List comprehension is a concise and expressive way to create lists in Python, and it allows us to create a new list by specifying its elements using a single line of code.
Basic Syntax:
result = [
expression
for outer_loop_variable in outer_iterable
for inner_loop_variable in inner_iterable
]
In the syntax, the expression
is the value to be included in the new list, and the comprehension iterates over combinations of elements from the outer and inner iterables. You can also include an optional if
statement for filtering.
Let’s go through an example using list comprehension. First of all, we will create two lists, listOne
and listTwo
, and then use them to print the result as shown below.
Example Code 1:
listOne = [1, 3, 5]
listTwo = [2, 4, 6]
[print(a + b) for a in listOne for b in listTwo]
Output:
3
5
7
5
7
9
7
9
11
In the code above, we have two lists, listOne
and listTwo
, with elements [1, 3, 5]
and [2, 4, 6]
, respectively. We utilize a list comprehension to iterate through all possible combinations of elements from these two lists, denoted by the nested for
loops.
For each pair of elements, represented by 'a'
from listOne
and 'b'
from listTwo
, we calculate their sum using the expression (a + b)
and print the sum of each combination using the print()
statement.
Let’s have another example where we nest a for
loop with a condition.
Example Code 2:
listOne = [1, 3, 5]
listTwo = [2, 4, 6]
result = [(a + b) for a in listOne for b in listTwo if a != b]
print(result)
Output:
[3, 5, 7, 5, 7, 9, 7, 9, 11]
In the code above, we initialize two lists, listOne
and listTwo
. We then employ a list comprehension with nested for
loops to iterate through all possible combinations of elements from the two lists.
Next, the condition if a != b
ensures that we exclude pairs where the elements from both lists are equal. For each valid pair, we calculate the sum using the expression a + b
.
The resulting list, named result
, stores these sums. Finally, we print the contents of the result
list.
The output, [3, 5, 7, 9, 7, 9, 11]
, reflects the sums of distinct pairs, excluding cases where elements from both lists are the same.
List comprehensions are preferred for writing nested for
loops in Python due to their concise and expressive syntax, which simplifies code structure and enhances readability, making it easier to write and understand complex nested iterations in a single line.
Nested for
Loop in One Line Using the exec()
Function in Python
Now, we will discuss another function that can help us achieve a nested for
loop in one line, the exec()
function. The exec()
function in Python is used for the dynamic execution of Python programs that are stored in strings or code objects, and It allows us to execute dynamically generated Python code.
Basic Syntax:
exec(object)
In the syntax, the object
is a string containing a Python program or a code object.
Now, let’s go through an example in which we will add two lists and print the sum as shown below.
Example Code 1:
listOne = [1, 3, 5]
listTwo = [2, 4, 6]
exec("for a in listOne:\n for b in listTwo:\n print(a+b)")
Output:
3
5
7
5
7
9
7
9
11
In the code above, we have two lists, listOne
and listTwo
. We use the exec()
function to dynamically execute a nested for
loop, iterating through all combinations of elements from listOne
and listTwo
.
Inside the loop, we print the sum of each pair (a, b)
using the print(a + b)
statement.
Now, let’s have another example using the .join()
string method that concatenates (joins) the elements of an iterable, such as a list(listOne
and listTwo
), using a specified string as the separator ("\n"
).
Example Code 2:
listOne = [1, 3, 5]
listTwo = [2, 4, 6]
expression = "\n".join(
f"a = {a}; b = {b}; print(a + b)" for a in listOne for b in listTwo
)
exec(expression)
Output:
3
5
7
5
7
9
7
9
11
In the above, we start with two lists, listOne
and listTwo
. Using a list comprehension, we create a string expression
by joining together formatted print statements for the sum of each pair (a, b)
from the Cartesian product of the two lists.
Next, the expression
is built using a generator expression with the f-string
syntax to incorporate the values of a
and b
. The "\n".join()
method is then applied to concatenate these formatted print statements, separated by newline characters.
Then, we use the exec()
function to dynamically execute the generated string expression
. During execution, each formatted print statement inside the string is evaluated, leading to the output displaying the sums of all possible combinations.
However, using exec()
in this way is generally not recommended for readability and security reasons. Using a list comprehension is usually a better approach.
Conclusion
In conclusion, the article demonstrates two methods for achieving nested for
loops in one line in Python. It first emphasizes the use of list comprehension, highlighting its concise and expressive syntax as the preferred approach for readability.
The second method involves employing the exec()
function, showcased with dynamic execution of nested loops. However, the article advises caution in using exec()
regularly due to readability and security concerns, reaffirming the preference for list comprehensions in most cases.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn