How to Sort With Lambda in Python
-
Understanding
sorted()
vs.sort()
-
Sorting with the
key
Parameter -
Using
lambda
Functions for Custom Sorting - Comparison Table: When to Use Each Sorting Method
- Conclusion
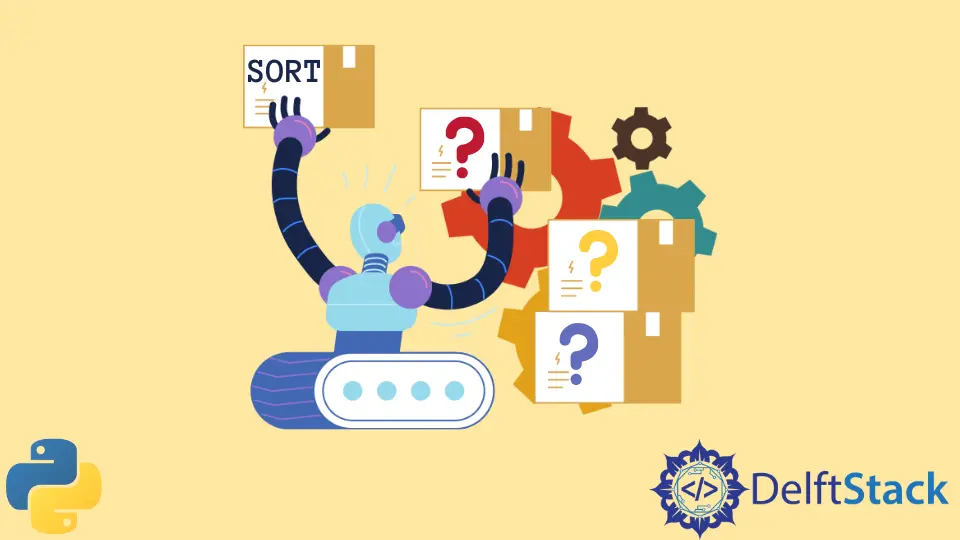
Sorting is a fundamental operation in Python, essential for organizing data efficiently. Python provides two built-in functions for sorting lists: sorted()
and sort()
. These functions allow sorting in ascending or descending order, and they support custom sorting logic using the key
parameter.
In this guide, we will explore the differences between sorted()
and sort()
, demonstrate how to use the key
parameter for custom sorting, and show practical examples using lambda
functions.
Understanding sorted()
vs. sort()
Before diving into sorting with lambda
functions, it’s important to understand how sorted()
and sort()
differ.
Function | Works On | Returns | In-Place Sorting? | Usage |
---|---|---|---|---|
sorted() |
Any iterable (lists, tuples, strings, dictionaries, etc.) | A new sorted list | ❌ No | sorted(lst) |
.sort() |
Only lists | Modifies the original list | ✅ Yes | lst.sort() |
Example: Difference Between sorted()
and .sort()
# Using sorted() (returns a new list)
numbers = [5, 3, 8, 1]
new_sorted_list = sorted(numbers)
print(new_sorted_list) # [1, 3, 5, 8]
print(numbers) # [5, 3, 8, 1] (unchanged)
# Using sort() (modifies the original list)
numbers.sort()
print(numbers) # [1, 3, 5, 8] (original list is modified)
When to Use Each Method?
- Use
sorted()
when you need a sorted copy without modifying the original list. - Use
.sort()
when sorting in place is preferred to save memory.
Sorting with the key
Parameter
The key
parameter allows us to define custom sorting logic. Instead of sorting based on default order (e.g., alphabetical or numerical), we can extract specific values before sorting.
Example: Sorting Words by Length
words = ["banana", "apple", "cherry", "blueberry"]
sorted_words = sorted(words, key=len)
print(sorted_words)
Output:
['apple', 'banana', 'cherry', 'blueberry']
Why? This sorts words by their length, not alphabetically.
Using lambda
Functions for Custom Sorting
lambda
functions provide a concise way to define custom sorting logic inline. Let’s explore different use cases.
1. Sorting Strings with Numerical Parts
lst = ["id01", "id10", "id02", "id12", "id03", "id13"]
lst_sorted = sorted(lst, key=lambda x: int(x[2:]))
print(lst_sorted)
Output:
['id01', 'id02', 'id03', 'id10', 'id12', 'id13']
Explanation: This ignores the first two characters (id
) and sorts based on the numeric value.
2. Sorting a List of Tuples by a Specific Element
lst = [("Mark", 1), ("Jack", 5), ("Jake", 7), ("Sam", 3)]
lst_sorted = sorted(lst, key=lambda x: x[1])
print(lst_sorted)
Output:
[('Mark', 1), ('Sam', 3), ('Jack', 5), ('Jake', 7)]
Explanation: This sorts by the second element in each tuple (the number).
3. Sorting in Descending Order
Use the reverse=True
argument to sort in descending order.
students = [("Alice", 85), ("Bob", 78), ("Charlie", 92)]
sorted_students = sorted(students, key=lambda x: x[1], reverse=True)
print(sorted_students)
Output:
[('Charlie', 92), ('Alice', 85), ('Bob', 78)]
Why? This sorts students by score in descending order.
4. Sorting a Dictionary by Values
scores = {"Alice": 85, "Bob": 78, "Charlie": 92}
sorted_scores = sorted(scores.items(), key=lambda x: x[1])
print(sorted_scores)
Output:
[('Bob', 78), ('Alice', 85), ('Charlie', 92)]
Why? Sorting a dictionary by values is a common use case.
5. Sorting by Last Name (Using split()
)
names = ["John Doe", "Jane Smith", "Alice Brown"]
sorted_names = sorted(names, key=lambda name: name.split()[-1])
print(sorted_names)
Output:
['Alice Brown', 'John Doe', 'Jane Smith']
Why? Sorting a list of full names by last name is useful in employee lists, contact apps, and databases.
6. Sorting a List of Objects
class Product:
def __init__(self, name, price):
self.name = name
self.price = price
products = [Product("Laptop", 1200), Product("Phone", 800), Product("Tablet", 600)]
sorted_products = sorted(products, key=lambda p: p.price)
for p in sorted_products:
print(p.name, p.price)
Output:
Tablet 600
Phone 800
Laptop 1200
Why? Sorting objects is essential in real-world applications like e-commerce or inventory management.
Comparison Table: When to Use Each Sorting Method
Sorting Method | Best Use Case |
---|---|
sorted() |
When you need a new sorted list without modifying the original |
.sort() |
When sorting lists in-place for memory efficiency |
key=lambda |
Custom sorting (e.g., sorting by second element in tuples) |
reverse=True |
Sorting in descending order |
Sorting Objects | When working with custom classes and data models |
Conclusion
Sorting lists efficiently is an essential skill in Python. We explored the differences between sorted()
and .sort()
, learned how to use the key
parameter for custom sorting, and demonstrated real-world examples using lambda
functions.
By mastering these techniques, you can handle complex sorting tasks effectively and write more optimized, readable, and Pythonic code.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn