How to Implement Selection Sort Algorithm in Python
- What is Selection Sort?
- Implementing Selection Sort in Python
- Analyzing the Time Complexity
- Conclusion
- FAQ
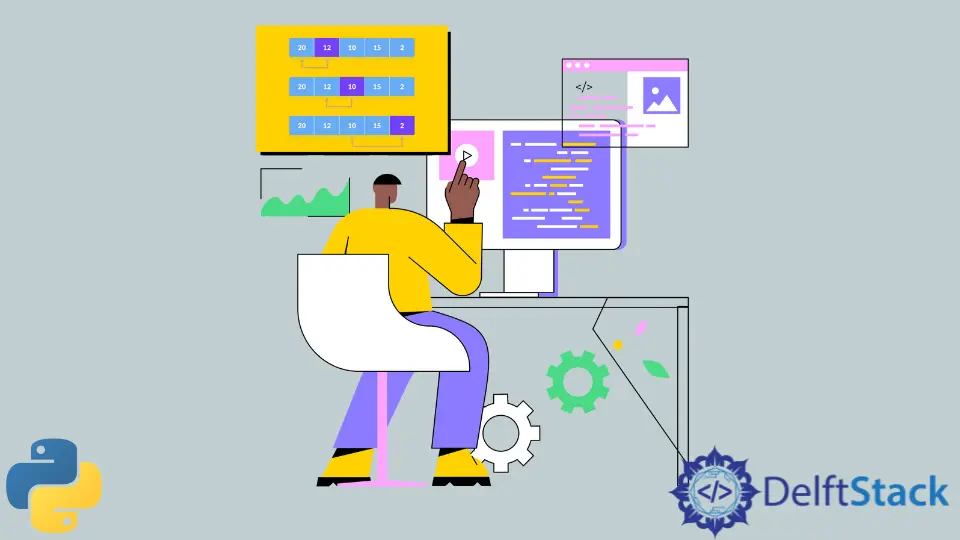
Sorting algorithms are fundamental to computer science and programming, and one of the simplest yet effective sorting algorithms is the selection sort.
This tutorial will guide you through the selection sort algorithm, its mechanics, and how to implement it in Python. Whether you’re a beginner looking to grasp the basics or an experienced programmer brushing up on your skills, this article will provide you with a clear understanding of selection sort. By the end, you’ll be able to implement this algorithm confidently in your own Python projects.
What is Selection Sort?
Selection sort is a straightforward sorting algorithm that works by repeatedly selecting the smallest (or largest, depending on the sorting order) element from the unsorted portion of the list and moving it to the sorted portion. The algorithm divides the list into two parts: the sorted section, which is built up from left to right, and the unsorted section, which is reduced as the algorithm progresses.
The process involves iterating through the list, finding the minimum element, and swapping it with the first element of the unsorted section. This continues until the entire list is sorted. While selection sort is not the most efficient for large datasets, it is an excellent educational tool for understanding the principles of sorting algorithms.
Implementing Selection Sort in Python
Now that we have a basic understanding of selection sort, let’s dive into how to implement it in Python. Below is a simple implementation of the selection sort algorithm.
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_index = i
for j in range(i + 1, n):
if arr[j] < arr[min_index]:
min_index = j
arr[i], arr[min_index] = arr[min_index], arr[i]
return arr
numbers = [64, 25, 12, 22, 11]
sorted_numbers = selection_sort(numbers)
print(sorted_numbers)
Output:
[11, 12, 22, 25, 64]
In this code, we define a function called selection_sort
, which takes a list arr
as an argument. We first determine the length of the list and then use a nested loop to find the minimum element in the unsorted section. The outer loop iterates through each element, while the inner loop looks for the smallest element. Once found, we swap it with the first element of the unsorted section. This process continues until the entire list is sorted. The result is a sorted list, which we print at the end.
Analyzing the Time Complexity
Understanding the time complexity of selection sort is crucial for evaluating its efficiency. The selection sort algorithm has a time complexity of O(n^2) in the worst and average cases. This is because for each element, we have to look through the remaining unsorted elements to find the minimum.
In the best-case scenario, where the list is already sorted, the time complexity remains O(n^2) because selection sort does not take advantage of pre-sorted lists. While this makes selection sort inefficient for large datasets, it does have the advantage of being easy to implement and understand.
The space complexity of selection sort is O(1), as it requires a constant amount of additional memory space for variables used in the sorting process. This makes it a suitable choice when memory usage is a concern, despite its slower performance compared to more advanced sorting algorithms like quicksort or mergesort.
Conclusion
In summary, the selection sort algorithm is a simple yet effective way to sort a list in Python. Its straightforward implementation allows beginners to grasp the concept of sorting algorithms easily. While it’s not the most efficient for large datasets, understanding its mechanics and time complexities can provide valuable insights into algorithm design. By practicing selection sort, you’ll build a solid foundation for tackling more complex sorting algorithms in the future.
FAQ
-
What is the main advantage of using selection sort?
Selection sort is easy to understand and implement, making it a great educational tool for beginners. -
Is selection sort efficient for large datasets?
No, selection sort has a time complexity of O(n^2), which makes it inefficient for large datasets compared to more advanced sorting algorithms. -
Can selection sort be used for sorting in descending order?
Yes, by modifying the comparison in the inner loop, selection sort can be easily adapted to sort in descending order. -
How does selection sort compare to bubble sort?
Both algorithms have a time complexity of O(n^2), but selection sort generally performs fewer swaps than bubble sort, making it slightly more efficient. -
Where is selection sort commonly used?
Selection sort is often used in educational settings to teach sorting concepts and is suitable for small datasets where simplicity is preferred over performance.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn