How to Sort Date and Time in Python
- Sort Date and Time in Python
-
Sort Dates Using the
sorted()
Method -
Sort Times Using the
sorted()
Method -
Sort Both Date and Time Using the
sorted()
Method - Conclusion
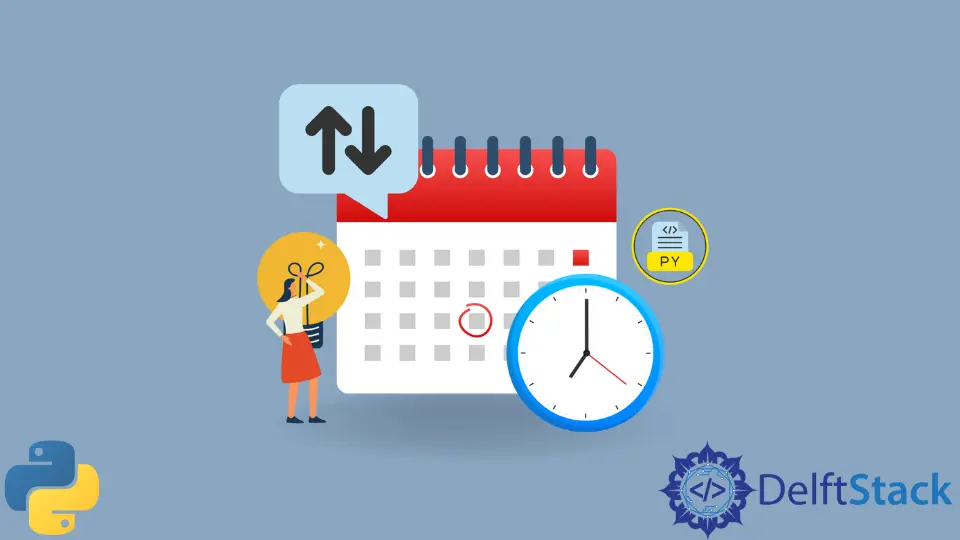
Python is a high-level and interpreted programming language that programmers use worldwide. It is most famous for object-oriented programming.
We can use Python in different IT sectors related to Artificial Intelligence, Machine Learning, web development, and data analysis. Another reason why Python is popular and practical is that it has many in-built libraries and modules.
This article will teach us how to sort dates and times using Python. We will also learn the datetime
module and the sorted
method.
Sort Date and Time in Python
One feature we can do using Python is sorting dates and times. There may be cases where we need to sort some given dates and times.
For example, if there is a list of different dates and times and we need to set them in ascending or descending order, we can sort them using Python.
the datetime
Module in Python
Before anything, there should be dates and times to sort. Python provides us with the datetime
module to work with dates and times easily.
There are six main classes under the datetime
module: date
, time
, datetime
, timedelta
, tzinfo
, and timezone
.
the sorted()
Method
sorted()
is an in-built function in Python that we can use to sort elements in a list. The syntax for the sorted()
method is below.
sorted(iterable, key=key, reverse=reverse)
Here the iterable
means the sequence or iterators we need to sort. It can be a tuple, a list, or a dictionary.
The key
and reverse
are optional values we can give to the sorted
function.
If we want to decide the sorting order, the key
is a function we can execute to achieve it. The default value is none
.
The reverse
is a Boolean that considers true
and false
values. If we set true
as the value, we will sort in descending order, and if we set false
as the value, we will sort the elements in ascending order.
The default value of the reverse
is false
.
We can use this method to sort dates and times. Let’s see how we can do that.
Sort Dates Using the sorted()
Method
As the first step, we should import the datetime
method from the datetime
module since we are working with the date and time.
from datetime import datetime
Then we can define a list with some random dates.
dateList = ["2022-10-07", "2022-10-04", "2022-10-31", "2022-10-01"]
Here the dates we have added have the same year and month but different days. Let’s try to sort them and have the output.
print(sorted(dateList))
We have used the sorted()
method inside the print()
function in the above statement. Also, we can assign that method to a variable and print it.
sortedDateList = sorted(dateList)
print(sortedDateList)
Both statements give us the same output. For this example, we use the first method.
Full code:
from datetime import datetime
dateList = ["2022-10-07", "2022-10-04", "2022-10-31", "2022-10-01"]
print(sorted(dateList))
Output:
As you can see, the dates have been sorted in ascending order.
Let’s sort them in descending order by adding the reverse
attribute with true
as the value.
print(sorted(dateList, reverse=True))
After running the code, we will get a result like the one below.
As in the above image, we can have sorted dates in descending order.
Now let’s try this again with different years and months.
dateList = ["2022-10-07", "2021-10-07", "2021-09-07", "2020-10-07", "2020-10-01"]
Then we can sort them and print them as we did earlier.
print(sorted(dateList))
Now we will get a result like in the below image.
Sort Times Using the sorted()
Method
Earlier, we tried to sort dates. Now let’s try to sort different times using this method.
Let’s import the datetime
method from the datetime
module.
from datetime import datetime
Then we can make a list with different times.
timeList = ["14:00:00", "02:00:00", "10:00:00", "23:00:00", "05:00:00"]
Now let’s try to sort and print them.
print(sorted(timeList))
Output:
As the output, we will get the time list in ascending order. Like we did earlier, we can also sort the times in descending order.
print("\n", sorted(timeList, reverse=True))
Output:
As shown below, let’s change the times with different minutes and seconds.
timeList = ["14:03:29", "02:24:23", "10:02:59", "02:23:24", "10:03:00"]
print(sorted(timeList))
When we run the code, we will get the expected result below.
Sort Both Date and Time Using the sorted()
Method
In previous steps, we sorted dates and times separately. Now let’s try to sort both dates and times together.
Let’s create a new list as dateTimeList
. Then we can add some dates along with different times, as shown in the below code block.
dateTimeList = [
"2022-10-07 14:03:29",
"2022-10-08 02:01:23",
"2022-10-07 10:02:59",
"2022-10-07 02:01:24",
]
As you can see, there are four dates and different times. Let’s see whether we can get the sorted list through this method.
print(sorted(dateTimeList))
Output:
As in the above image, we will get a sorted list of the dates and times.
Conclusion
In this tutorial, we learned a Python technique: the sorted()
method to sort the date and time.
As the first step, we should import the datetime
module, and from that, we should also import the datetime
method. Only then can we work with the date and time.
Using the sorted()
method, we created lists with different dates and times and sorted them as examples to understand the concept. There are other methods to sort dates and times, but this is a simple way that Python provides us.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.