The send_keys() Function in Selenium Python
-
the
send_keys()
Function in Selenium Python -
Input Validation Using the
send_keys()
Function in Selenium Python -
Erase Texts Using the
send_keys()
Function in Selenium Python
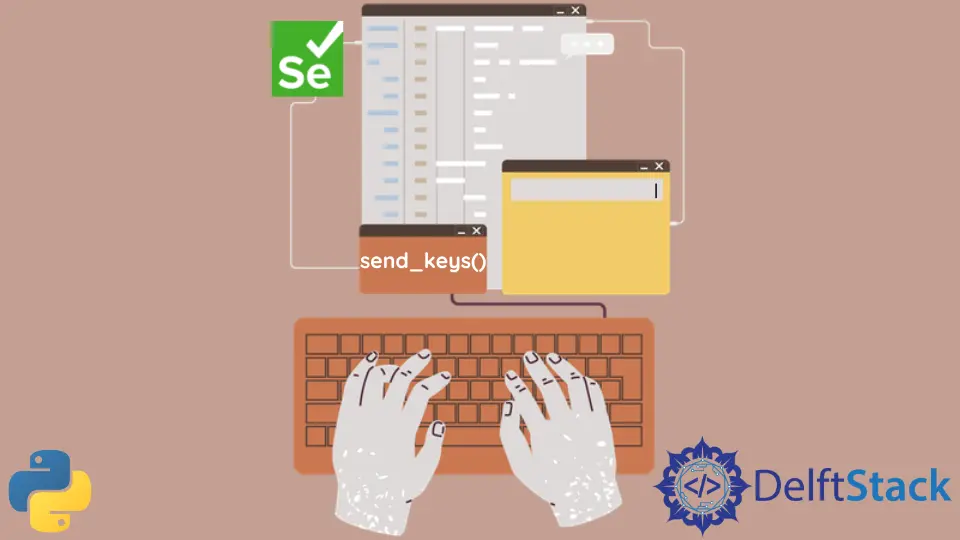
We will introduce the send_keys()
function in Selenium Python and demonstrate its uses.
Any application needs to go through some tests before getting a market. The application should first meet all the requirements associated with its name.
We should test applications comprehensively since no one predicts the exact input given to the application. Python Selenium can help us to test all the probable causes.
send_keys()
is a process in which keyboard inputs such as numbers, texts, and symbols are sent to the text boxes of an application. send_keys()
is a part of WebDriver, and each keyboard input is sent to this element.
the send_keys()
Function in Selenium Python
The first step involved in this method is to open the web application that we want to test. We can open the application using its URL.
Wait for the whole page to load successfully as the test fails if the application is a dynamic type, but the webpage is not fully loaded while applying the test.
The next step involves selecting and searching the desired element sent to the keyboard inputs. This element is usually a text box that allows the user to write within it.
The element can be a search tab or some form. We can use several options such as element ID, element name, and XPath to find the elements.
After selecting the elements, keyboard inputs are sent using the send_keys
method. We can find an element using the id, class name, or the xpath.
The syntax for each method is shown below.
# python
getElemById = driver.find_element_by_id("myId")
getElemByName = driver.find_element_by_name("myClass")
getElemByXPath = driver.find_element_by_xpath("//input[@id='myId']")
The main application of send_keys()
is testing the application for numerous inputs. However, it is important to learn about the two inputs commonly used for this method.
Input Validation Using the send_keys()
Function in Selenium Python
Most of the applications have a login form for which the user’s email address is widely used. Since we all know that each email address has a standard template starting with a username, includes the symbol @
, and followed by the domain name.
Now, we should add the process for validating the email address.
This will not allow the user to login into the application by writing an invalid email address to the login form. The validation can be confirmed by adding and checking the application by sending the inputs that do not follow the main template.
Additional validations can also get involved in this process. Since the password to log in to the application has a specific set of rules, this validation can be checked by sending inputs that do not follow the rules.
The search bar present in the application should also be checked. A search bar should show the relevant results to guide the user with the desired search element properly.
send_keys()
is a handy tool to check the expected results from the search bar.
Some web applications include special functions that should get checked before publishing the app. One example of a special function is Google Meet, which uses Ctrl+D to mute or unmute the microphone.
Example code:
# python
from selenium import webdriver
Chromedriver = webdriver.Chrome()
Chromedriver.get("https://www.google.com/")
getElemById = Chromedriver.find_element_by_name("gLFyf")
getElemById.send_keys("This is filed by Selenium send keys")
Output:
As you can see from the above example, with the help of the send_keys()
method of selenium, we can fill any input fields on any website.
Erase Texts Using the send_keys()
Function in Selenium Python
We can also edit and change the values of keys using the same send_keys()
function. Let’s go through an example in which we will change the value of the input, and then we will change it again.
In this example, we will send the key; after sleeping for 3 seconds, we will delete 3 letters from the key. And in the end, we will change the value again, as shown below.
Example code:
# python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
link = "https://www.google.com/"
chromeDriver = webdriver.Chrome("C:\chromedriver.exe")
driverOptions = webdriver.ChromeOptions()
windowSize = chromeDriver.get_window_size()
chromeDriver.get(link)
getElemByName = chromeDriver.find_element_by_name("gLFyf")
getElemByName.send_keys("For test purpose")
time.sleep(3)
getElemByName.send_keys(3 * Keys.BACKSPACE)
time.sleep(3)
getElemByName.send_keys("testing second key")
time.sleep(3)
getElemByName.clear()
time.sleep(3)
chromeDriver.quit()
First step:
Second step:
Third step:
Troubleshooting Issues:
- We should ensure that the chosen element is from the keyboard inputs.
- Identification of the element should be given with proper reference to avoid complications.
- Always follow the proper method: search the element, click on the element, and then send keys.
send_keys()
can fail when an element is not active or out of reach. Make sure to count these factors while working on a specific element.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python