How to Remove NaN From List in Python
-
Remove
NaN
From the List in Python Using themath.isnan()
Method -
Remove
NaN
From the List in Python Using thenumpy.isnan()
Method -
Remove
NaN
From the List of Strings in Python -
Remove
NaN
From the List in Python Using thepandas.isnull()
Method - Conclusion
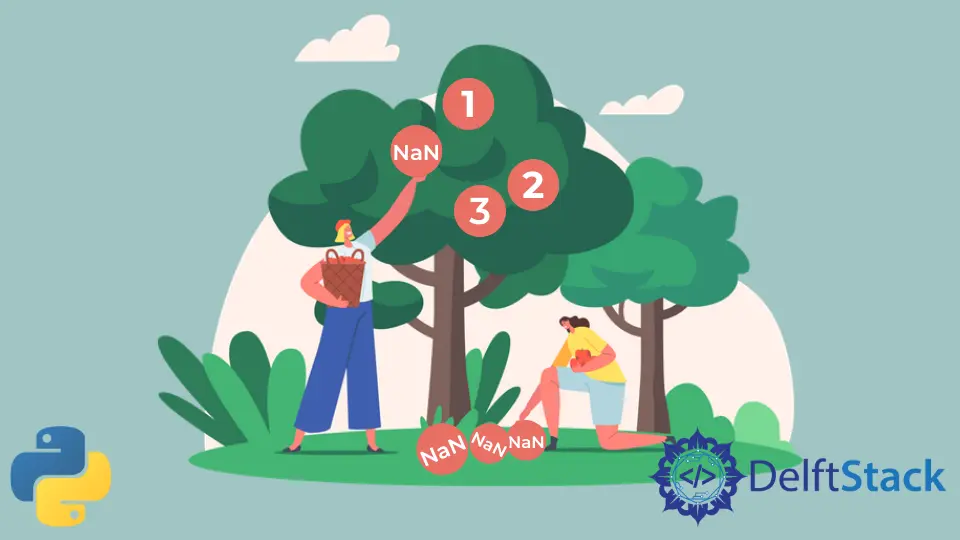
Data preprocessing is a crucial step in data analysis and manipulation. Often, datasets contain missing or invalid data, represented by NaN
(Not-a-Number) values.
Python offers various methods to effectively handle and remove NaN
values from lists. In this article, we explore different methods used in this chat session to clean data and ensure its quality.
By the end of this article, you’ll be equipped with the knowledge to tackle missing or invalid data, regardless of your data type or complexity.
Remove NaN
From the List in Python Using the math.isnan()
Method
You can remove NaN
values from a list using the math.isnan()
function, which allows you to check for NaN
values and filter them out effectively. Its syntax is straightforward:
math.isnan(x)
x
: This is the value you want to check. It can be any numeric or non-numeric value (e.g., a float, integer, or even a string that represents a number).
The math.isnan()
function returns True
if the value x
is NaN
; otherwise, it returns False
.
Before you can use the math.isnan()
method, you need to import the math
module. This module provides various mathematical functions, including isnan()
, which checks if a given value is NaN
.
Here’s how you can import the math
module:
import math
To remove NaN
values from a list, you can use list comprehension in combination with the math.isnan()
method.
Here are the steps to remove NaN
from a list in Python using the math.isnan()
method:
-
Import the
math
module.import math
-
Define your original list containing
NaN
values.original_list = [1, 2, float("nan"), 4, float("nan")]
-
Use list comprehension to create a new list without
NaN
values.cleaned_list = [x for x in original_list if not math.isnan(x)]
The list comprehension iterates through each element
x
in theoriginal_list
and only includes elements wheremath.isnan(x)
returnsFalse
. The result is acleaned_list
without anyNaN
values.
Let’s illustrate the process with an example:
import math
original_list = [1, 2, float("nan"), 4, float("nan")]
cleaned_list = [x for x in original_list if not math.isnan(x)]
print(cleaned_list)
Output:
[1, 2, 4]
When you run this code, the cleaned_list
will only contain valid numeric values, and any NaN
values will be removed.
Remove NaN
From the List in Python Using the numpy.isnan()
Method
To clean up your data and remove the NaN
values from a list, you can also utilize the powerful NumPy library. NumPy provides an efficient method called numpy.isnan()
to identify and remove NaN
values in arrays or lists.
Its syntax is as follows:
numpy.isnan(x)
x
: This is the value or array you want to check forNaN
. It can be a single numeric value, a NumPy array, or a list of values.
The numpy.isnan()
function returns True
for NaN
values and False
for non-NaN
values. If you apply it to an array or list, it returns a Boolean array with True
at positions where NaN
values are present.
Before you can use the numpy.isnan()
method, you need to make sure you have the NumPy library installed. You can install it using pip
:
pip install numpy
Then, you need to import the NumPy library in your Python script:
import numpy as np
Here’s how you can use it to remove NaN
values from a list:
-
Import NumPy and create your original list containing
NaN
values:import numpy as np original_list = [1, 2, np.nan, 4, np.nan]
-
Use the
numpy.isnan()
method to create a mask ofNaN
values:nan_mask = np.isnan(original_list)
-
Apply the mask to the original list to create a new list without
NaN
values:cleaned_list = np.array(original_list)[~nan_mask].tolist()
In the above code, we first use
np.isnan()
to create a Boolean mask, which containsTrue
forNaN
values andFalse
for non-NaN
values. Then, we use this mask to filter outNaN
values from the original list, resulting in acleaned_list
.
Let’s illustrate the process with an example:
import numpy as np
original_list = [1, 2, np.nan, 4, np.nan]
nan_mask = np.isnan(original_list)
cleaned_list = np.array(original_list)[~nan_mask].tolist()
print(cleaned_list)
The output of this code will be the cleaned_list
, which is a list containing only valid numeric values (i.e., NaN
values have been removed).
The output, when you run this code, will be:
[1.0, 2.0, 4.0]
The NaN
values (represented by np.nan
) have been successfully removed from the original_list
, leaving only the valid numeric values in the cleaned_list
.
Remove NaN
From the List of Strings in Python
When your list contains a mix of numeric and string values, it’s essential to handle any NaN
values consistently.
Once you convert the list to a string data type, NaN
values are no longer represented as float('nan')
. Instead, they become string values equal to 'nan'
.
To remove these 'nan'
strings, you can compare each element in the list to the string 'nan'
.
To remove 'nan'
strings from a list of strings, first, convert each element to a string data type before comparing the list elements to 'nan'
. This ensures that both numeric and string values are treated uniformly.
mylist = [1, 2, "nan", 8, 6, 4, "nan"]
mylist = [str(x) for x in mylist]
Here, we use a list comprehension to iterate through each element and convert it to a string.
Then, use list comprehension to create a new list that excludes the 'nan'
strings by comparing each element to the string 'nan'
.
newlist = [x for x in mylist if x != "nan"]
This list comprehension checks each element (x
) in the mylist
and includes it in the newlist
only if it is not equal to "nan"
.
Let’s illustrate the process with an example:
mylist = [1, 2, "nan", 8, 6, 4, "nan"]
mylist = [str(x) for x in mylist]
newlist = [x for x in mylist if x != "nan"]
print(mylist)
print(newlist)
The output of this code will be:
['1', '2', 'nan', '8', '6', '4', 'nan']
['1', '2', '8', '6', '4']
Here, you’ll see that the first list (mylist
) shows the original list with elements converted to strings. It includes the string nan
.
Then, the second list (newlist
) is the modified list after removing the string nan
. It contains only the valid numeric and string values without any occurrences of nan
.
Remove NaN
From the List in Python Using the pandas.isnull()
Method
Python’s pandas.isnull()
method is a function used for detecting missing or invalid data. What makes this method especially versatile is its ability to handle various data types, including string data, making it a robust solution for data preprocessing tasks.
The syntax of the pandas.isnull()
method is straightforward:
pandas.isnull(obj)
obj
: Represents the input scalar or array-like object to be tested forNaN
values.
The method returns True
if the value in obj
is NaN
, None
, or NaT
, and False
otherwise.
To remove NaN
values from a Python list, first, you need to import the Pandas library to access the pandas.isnull()
method:
import pandas as pd
This ensures you have the necessary library for data preprocessing.
Next, create your original list, which may contain NaN
values. This list can contain diverse data types, including numeric and string values:
mylist = [1, 2, float("nan"), 8, float("nan"), 4, float("nan")]
print(mylist)
Here, the list contains a mixture of numeric and NaN
values represented as float("nan")
.
Now, use list comprehension with the pandas.isnull()
method to create a new list that excludes the NaN
values. This method effectively identifies and removes NaN
values, making it suitable for handling diverse data types:
newlist = [x for x in mylist if pd.isnull(x) == False]
print(newlist)
In this line, x
represents each element in the mylist
. The condition pd.isnull(x) == False
checks if x
is not NaN
, and if it’s not, the element is included in the newlist
.
Here’s the complete working code for this example:
import pandas as pd
mylist = [1, 2, float("nan"), 8, float("nan"), 4, float("nan")]
print("Original List:")
print(mylist)
newlist = [x for x in mylist if pd.isnull(x) == False]
print("List without NaN values:")
print(newlist)
When you run this code, it will print the original list and then the modified list without the NaN
values.
Here’s what the output will look like:
Original List:
[1, 2, nan, 8, nan, 4, nan]
List without NaN values:
[1, 2, 8, 4]
The NaN
values have been successfully removed from the list, leaving only the valid numeric values.
Handling NaN
and 'nan'
Values
Suppose you have a list that may contain various data types, and you want to remove both NaN
and 'nan'
values. In this case, the pandas.isnull()
method can handle diverse data types, including string data:
mylist = ["John", 23, "nan", "New York", float("nan")]
print(mylist)
newlist = [x for x in mylist if pd.isnull(x) == False and x != "nan"]
print(newlist)
The pandas.isnull()
method can effectively identify and remove both NaN
and 'nan'
values, resulting in a clean newlist
.
Here’s the complete working code for the second example:
import pandas as pd
mylist = ["John", 23, "nan", "New York", float("nan")]
print("Original List:")
print(mylist)
newlist = [x for x in mylist if pd.isnull(x) == False and x != "nan"]
print("List without NaN and 'nan' values:")
print(newlist)
Here’s what the output will look like:
Original List:
['John', 23, 'nan', 'New York', nan]
List without NaN and 'nan' values:
['John', 23, 'New York']
The NaN
and 'nan'
values have been successfully removed from the list, leaving only the valid data.
Conclusion
Ensuring data quality is paramount in data analysis and manipulation. Handling NaN
values is a fundamental aspect of this process.
In this article, we’ve explored several methods to remove NaN
values from lists in Python: math.isnan()
, numpy.isnan()
, list comprehension, and pandas.isnull()
. Each method provides a unique solution suitable for different data types and scenarios.
Whether you’re working with purely numeric data, mixed data types, or string data, these methods offer flexibility and efficiency in cleaning and preprocessing your data. By mastering these techniques, you can ensure the integrity of your datasets and make them ready for in-depth analysis and further processing.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python