How to Read First Line of a File in Python
-
Read the First Line of a File in Python Using the
readline()
Method -
Read the First Line of a File in Python Using a
for
Loop -
Read the First Line of a File in Python Using the
readlines()
Method -
Read the First Line of a File in Python Using the
read()
Method -
Read the First Line of a Text File in Python Using Context Managers and
readline()
- Conclusion
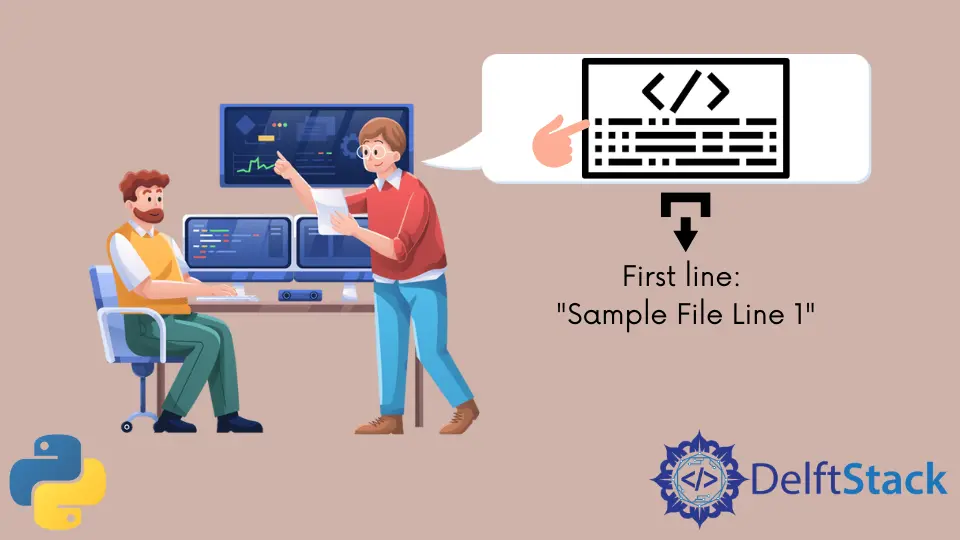
Reading the first line of a text file is a fundamental task in Python, and there are multiple methods to achieve this. Each method offers a different approach, providing flexibility and options based on the requirements of your program.
In this article, we will explore several methods to read the first line of a text file.
First, let’s create a text file (e.g., example.txt
) and add some content to it. For demonstration purposes, let’s assume our file contains the following content:
This is the first line of the text file.
This is the second line.
And this is the third line.
Read the First Line of a File in Python Using the readline()
Method
The readline()
method reads a single line from the file and advances the file pointer to the next line. By calling this method once, we can read the first line of the text file.
See the example code below to read the first line of a text file:
# File path
filename = "example.txt"
# Open the file
with open(filename, "r") as file:
# Read the first line and strip any leading or trailing whitespace
first_line = file.readline().strip()
# Print the first line
print("The first line of the file is:", first_line)
Output:
The first line of the file is: This is the first line of the text file.
In this example code:
-
First, we use the
with open(...)
statement to open the file in read mode. -
file.readline()
is used to read the first line of the file. -
strip()
is used to remove any leading or trailing whitespace, including newline characters. -
The first line is then printed to the console.
Read the First Line of a File in Python Using a for
Loop
We can also use a for
loop to iterate through the file line by line and stop after reading the first line.
Below is an example code on how we can use this to read the first line of the text file:
# File path
filename = "example.txt"
# Open the file
with open(filename, "r") as file:
# Iterate through the file line by line
for line in file:
# Strip any leading or trailing whitespace
first_line = line.strip()
# Print the first line
print("The first line of the file is:", first_line)
# Break the loop after reading the first line
break
Output:
The first line of the file is: This is the first line of the text file.
In this example code:
-
We use the
with open(...)
statement to open the file in read mode. -
The
for
loop iterates through each line in the file. -
line.strip()
is used to remove any leading or trailing whitespace, including newline characters. -
We print the first line and then break the loop to stop reading after the first line.
Read the First Line of a File in Python Using the readlines()
Method
The readlines()
method in Python reads all the lines of a file and returns them as a list of strings. To read only the first line, we can read the first element of this list.
Here’s an example of how we can read the first line of the text file using the readlines()
method:
# Specify the file path
file_path = "example.txt"
# Open the file in read mode and read the first line
with open(file_path, "r") as file:
first_line = file.readlines()[0]
# Print the first line
print("First line of the file:", first_line)
Output:
The first line of the file is: This is the first line of the text file.
In this example code:
-
We define the
file_path
variable to store the path to the desired text file. -
Then, we use the
open()
function to open the file in read mode ('r'
), returning a file object. -
We use the
readlines()
method to read all lines of the file and convert them into a list. -
Extract the first element of the list to obtain the first line.
Read the First Line of a File in Python Using the read()
Method
The read()
method in Python is typically used to read a specified number of bytes from a file, or if no size is specified, it will read the entire file. To read only the first line, we can specify the number of characters to read or stop reading at the first newline character.
Here’s how we can read the first line of the text file using the read()
method:
# Specify the file path
file_path = "example.txt"
# Open the file in read mode and read the first line
with open(file_path, "r") as file:
first_line = file.read().split("\n")[0]
# Print the first line
print("First line of the file:", first_line)
Output:
The first line of the file is: This is the first line of the text file.
In this example code:
-
First, we define the file path to store the path to the desired text file.
-
We use the
open()
function to open the file in read mode ('r'
), returning a file object. -
Then, we use the
read()
method to read the content of the file as a string. -
Finally, we split the content using newline (
'\n'
) and extracted the first element to obtain the first line.
Read the First Line of a Text File in Python Using Context Managers and readline()
Python’s with
statement, in combination with context managers, provides a convenient way to open a file, read its content, and automatically close the file when done. Let’s use a context manager to read the first line of a text file.
Here’s a step-by-step guide to reading the first line of the text file using a context manager and the readline()
method:
# File path
filename = "example.txt"
# Open the file using a context manager
with open(filename, "r") as file:
# Read the first line and strip any leading or trailing whitespace
first_line = file.readline().strip()
# Print the first line
print("The first line of the file is:", first_line)
In this example:
-
We used the
with open(...)
statement to open the file in read mode. -
file.readline()
is used to read the first line of the file. -
strip()
is used to remove any leading or trailing whitespace, including newline characters. -
The first line is then printed to the console.
Save the Python script to a file (e.g., read_first_line.py
) and run it using the command:
python read_first_line.py
Output:
The first line of the file is: This is the first line of the text file.
Conclusion
Reading the first line of a text file in Python can be achieved through various methods, each offering its advantages. The choice of method depends on the specific use case and preferences of the developer.
By understanding these methods, you can efficiently read the first line of a text file and integrate this functionality into your Python programs.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn