How to Solve Quadratic Equations in Python
- Understanding Quadratic Equations
- Method 1: Using the Quadratic Formula
- Method 2: Using NumPy Library
- Method 3: Using SymPy for Symbolic Mathematics
- Conclusion
- FAQ
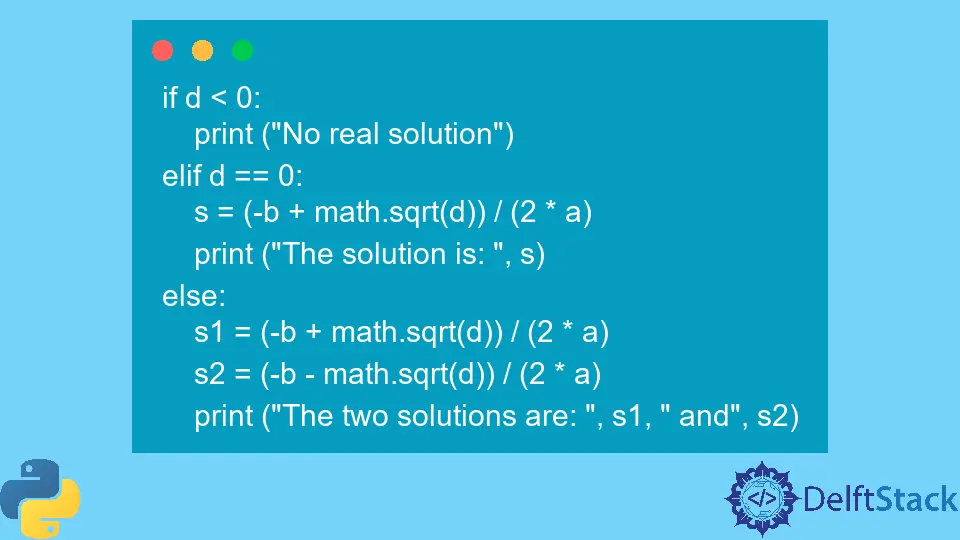
Quadratic equations are fundamental in algebra, appearing in various fields such as physics, engineering, and economics. If you’ve ever found yourself needing to solve these equations, Python offers a straightforward way to do so.
In this tutorial, we’ll explore how to solve quadratic equations using Python, leveraging its powerful libraries and built-in functions. Whether you’re a beginner or an experienced programmer, this guide will walk you through different methods to tackle quadratic equations effectively. By the end, you’ll have a solid understanding of how to implement these solutions in your own projects.
Understanding Quadratic Equations
Before diving into the code, let’s revisit what a quadratic equation is. A quadratic equation is typically expressed in the standard form:
[ ax^2 + bx + c = 0 ]
Here, ( a ), ( b ), and ( c ) are constants, and ( x ) represents the variable. The solutions to this equation can be found using the quadratic formula:
[ x = \frac{{-b \pm \sqrt{{b^2 - 4ac}}}}{2a} ]
This formula provides the roots of the equation, which can be real or complex numbers depending on the value of the discriminant (( b^2 - 4ac )). Now, let’s explore how to implement this in Python.
Method 1: Using the Quadratic Formula
The simplest way to solve a quadratic equation in Python is by directly implementing the quadratic formula. This method is straightforward and effective for most cases.
import cmath
def solve_quadratic(a, b, c):
d = (b**2) - (4*a*c)
root1 = (-b + cmath.sqrt(d)) / (2 * a)
root2 = (-b - cmath.sqrt(d)) / (2 * a)
return root1, root2
a = 1
b = 5
c = 6
solutions = solve_quadratic(a, b, c)
Output:
((-2+0j), (-3+0j))
In this code, we first import the cmath
library, which allows us to handle complex numbers. The function solve_quadratic
takes three parameters: ( a ), ( b ), and ( c ). We calculate the discriminant ( d ) and then find the two roots using the quadratic formula. The roots are returned as a tuple. For the given values of ( a ), ( b ), and ( c ), the output shows the two real roots of the equation.
Method 2: Using NumPy Library
Another effective way to solve quadratic equations in Python is by utilizing the NumPy library, which provides a more efficient and concise approach.
import numpy as np
def solve_quadratic_numpy(a, b, c):
coefficients = [a, b, c]
roots = np.roots(coefficients)
return roots
a = 1
b = 5
c = 6
solutions = solve_quadratic_numpy(a, b, c)
Output:
[-2. -3.]
In this approach, we use the numpy.roots
function, which takes a list of coefficients and returns the roots of the polynomial. This method is particularly useful when dealing with higher-order polynomials as well. The output shows the roots of the quadratic equation, which are both real numbers in this case. Using NumPy can significantly speed up calculations, especially for large datasets or complex equations.
Method 3: Using SymPy for Symbolic Mathematics
If you’re interested in symbolic computation, the SymPy library provides a robust way to solve quadratic equations. This method is particularly useful for obtaining exact solutions rather than numerical approximations.
from sympy import symbols, Eq, solve
def solve_quadratic_sympy(a, b, c):
x = symbols('x')
equation = Eq(a*x**2 + b*x + c, 0)
roots = solve(equation, x)
return roots
a = 1
b = 5
c = 6
solutions = solve_quadratic_sympy(a, b, c)
Output:
[-3, -2]
Here, we first import the necessary functions from the SymPy library. We define the variable ( x ) and construct the equation using Eq
. The solve
function then finds the roots of the equation. The output displays the exact roots of the quadratic equation, which can be beneficial for further symbolic manipulation or analysis.
Conclusion
Solving quadratic equations in Python can be done in various ways, each suitable for different scenarios. Whether you choose to use the quadratic formula directly, leverage the power of NumPy for efficiency, or utilize SymPy for symbolic solutions, Python provides a versatile toolkit for handling these mathematical challenges. With the methods outlined in this tutorial, you can confidently solve quadratic equations and apply these techniques to more complex problems in your programming journey.
FAQ
-
What is a quadratic equation?
A quadratic equation is a polynomial equation of degree two, typically in the form ax^2 + bx + c = 0. -
Why should I use Python to solve quadratic equations?
Python offers powerful libraries and built-in functions that simplify the process of solving quadratic equations. -
What is the quadratic formula?
The quadratic formula is x = (-b ± sqrt(b^2 - 4ac)) / (2a), used to find the roots of a quadratic equation. -
Can I solve quadratic equations with complex roots in Python?
Yes, using the cmath library allows you to handle complex numbers when solving quadratic equations. -
Which library is best for solving quadratic equations in Python?
It depends on your needs. NumPy is great for numerical solutions, while SymPy is excellent for symbolic computations.