How to Fix ValueError: Not Enough Values to Unpack in Python
-
What Is a
ValueError
in Python -
Fix
ValueError: not enough values to unpack
in Python Dictionaries -
Fix
ValueError: not enough values to unpack
in Python
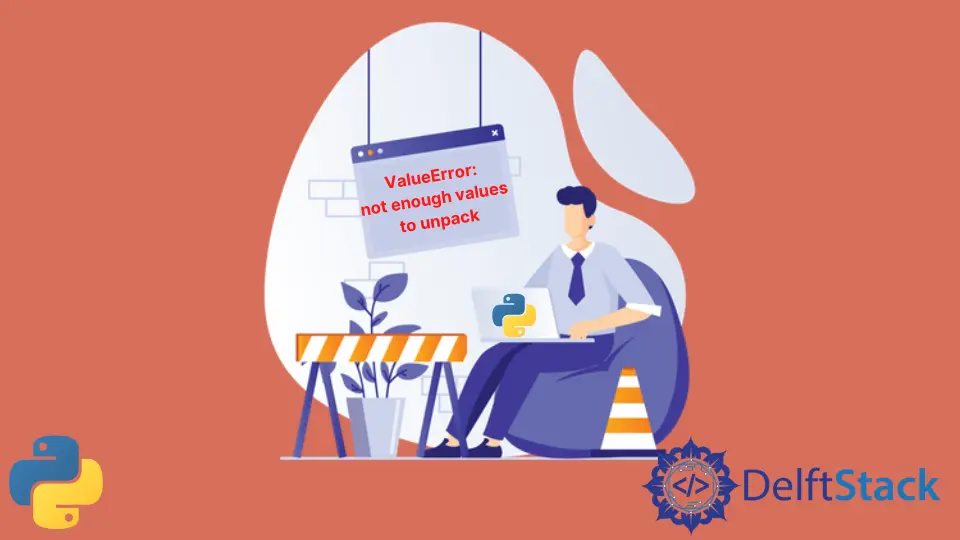
It is a common error when you are freshly getting your hands dirty with Python programming, or sometimes it could be a type error that you provide more values but fewer variables (containers) to catch those values. Or when you try to iterate over a dictionary’s values or keys but try to access both simultaneously.
This article will look at each scenario in detail along with examples but before that, let’s understand what a ValueError
is in Python.
What Is a ValueError
in Python
A ValueError
is a common exception in Python that occurs when the number of values doesn’t match the number of variables either taking input, direct assignment or through an array or accessing restricted values. To understand the ValueError
, let’s take an example.
# this input statement expects three values as input
x, y, z = input("Enter values for x, y and z: ").split(",")
Output:
Enter values for x, y and z: 1,2
ValueError: not enough values to unpack (expected 3, got 2)
In the above example, we have three variables x,y,z
to catch the input values, but we provide two input values to demonstrate the ValueError
.
Now the input
statement has three values, and since the user input values do not meet the expected condition, it throws the ValueError: not enough values to unpack (expected 3, got 2)
.
The error itself is self-explanatory; it tells us that the expected number of values is three, but you have provided 2.
A few other common causes of ValueError
could be the following.
a, b, c = 3, 5 # ValueError: not enough values to unpack (expected 3, got 2)
a, b, c = 2 # ValueError: not enough values to unpack (expected 3, got 1)
# ValueError: not enough values to unpack (expected 4, got 3)
a, b, d, e = [1, 2, 3]
Fix ValueError: not enough values to unpack
in Python Dictionaries
In Python, a dictionary is another data structure whose elements are in key-value pairs, every key should have a corresponding value against the key, and you can access the values with their keys.
Syntax of a dictionary in Python:
student = {"name": "Zeeshan Afridi", "degree": "BSSE", "section": "A"}
This is the general structure of a dictionary; the values of the left are keys, whereas the others are values of the keys.
We have specified functions for Python, dictionaries like keys()
, values()
, items()
, etc. But these are the most common and useful functions to loop through a dictionary.
print("Keys of Student dictionary: ", student.keys())
print("Values of Student dictionary: ", student.values())
print("Items of Student dictionary: ", student.items())
Output:
Keys of Student dictionary: dict_keys(['name', 'degree', 'section'])
Values of Student dictionary: dict_values(['Zeeshan Afridi', 'BSSE', 'A'])
Items of Student dictionary: dict_items([('name', 'Zeeshan Afridi'), ('degree', 'BSSE'), ('section', 'A')])
Let’s see why the ValueError: not enough values to unpack
occurs in Python dictionaries.
student = {
# Keys : Values
"name": "Zeeshan Afridi",
"degree": "BSSE",
"section": "A",
}
# iterate user dictionary
for k, v, l in student.items(): # This statement throws an error
print("Key:", k)
print("Value:", str(v))
Output:
ValueError: not enough values to unpack (expected 3, got 2)
As you can see, the above code has thrown an error because the .items()
functions expect two variables to catch the keys and values of the student
dictionary, but we have provided three variables k,v,l
.
So there isn’t any space for l
in the student
dictionary, and it throws the ValueError: not enough values to unpack (expected 3, got 2)
.
To fix this, you need to fix the variables of the dictionary.
for k, v in student.items():
This is the correct statement to iterate over a dictionary in Python.
Fix ValueError: not enough values to unpack
in Python
To avoid such exceptions in Python, you should provide the expected number of values to the variables and display useful messages to guide yours during entering data into forms or any text fields.
In addition to that, you can use try-catch
blocks to capture such errors before crashing your programs.
Let’s understand how to fix the ValueError: not enough values to unpack
in Python.
# User message --> Enter three numbers to multiply ::
x, y, z = input("Enter three numbers to multiply :: ").split(",")
# type casting x,y, and z
x = int(x)
y = int(y)
z = int(z)
prod = x * y * z
print("The product of x,y and z is :: ", prod)
Output:
Enter three numbers to multiply :: 2,2,2
The product of x,y and z is :: 8
Here in this example, the input
statement expects three inputs, and we have provided the expected number of inputs, so it didn’t throw any ValueError
.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python