ValueError: Python에서 압축을 풀 값이 충분하지 않음
-
Python에서
ValueError
란 무엇입니까? -
Python 사전에서
ValueError: not enough values to unpack
수정 -
Python에서
ValueError: not enough values to unpack
수정
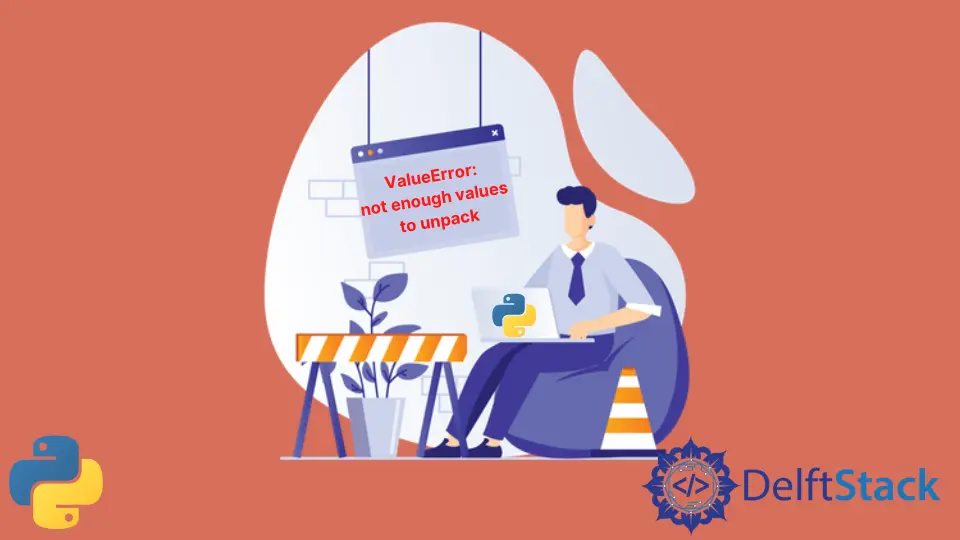
Python 프로그래밍으로 갓 손을 더럽힐 때 흔히 발생하는 오류이거나 때로는 더 많은 값을 제공하지만 해당 값을 포착하기 위한 더 적은 변수(컨테이너)를 제공하는 유형 오류일 수 있습니다. 또는 사전의 값이나 키를 반복하려고 하지만 둘 다 동시에 액세스하려고 할 때.
이 기사에서는 예제와 함께 각 시나리오를 자세히 살펴보겠지만 그 전에 Python에서 ValueError
가 무엇인지 알아보겠습니다.
Python에서 ValueError
란 무엇입니까?
ValueError
는 값의 수가 입력, 직접 할당 또는 배열을 통해 또는 제한된 값에 액세스하는 변수의 수와 일치하지 않을 때 발생하는 Python의 일반적인 예외입니다. ValueError
를 이해하기 위해 예를 들어 보겠습니다.
# this input statement expects three values as input
x, y, z = input("Enter values for x, y and z: ").split(",")
출력:
Enter values for x, y and z: 1,2
ValueError: not enough values to unpack (expected 3, got 2)
위의 예에서 입력 값을 포착하기 위한 세 개의 변수 x,y,z
가 있지만 ValueError
를 시연하기 위해 두 개의 입력 값을 제공합니다.
이제 input
문에는 3개의 값이 있으며 사용자 입력 값이 예상 조건을 충족하지 않기 때문에 ValueError: not enough values to unpack (expected 3, got 2)
가 발생합니다.
오류 자체는 자명합니다. 예상되는 값의 수가 3개라고 알려주지만 2개를 제공했습니다.
ValueError
의 몇 가지 다른 일반적인 원인은 다음과 같습니다.
a, b, c = 3, 5 # ValueError: not enough values to unpack (expected 3, got 2)
a, b, c = 2 # ValueError: not enough values to unpack (expected 3, got 1)
# ValueError: not enough values to unpack (expected 4, got 3)
a, b, d, e = [1, 2, 3]
Python 사전에서 ValueError: not enough values to unpack
수정
Python에서 사전은 요소가 키-값 쌍에 있는 또 다른 데이터 구조이며, 모든 키는 해당 키에 대한 값을 가져야 하며 해당 키를 사용하여 값에 액세스할 수 있습니다.
Python의 사전 구문:
student = {"name": "Zeeshan Afridi", "degree": "BSSE", "section": "A"}
이것은 사전의 일반적인 구조입니다. 왼쪽의 값은 키이고 다른 값은 키의 값입니다.
우리는 keys()
, values()
, items()
등과 같은 Python용 함수를 지정했습니다. 그러나 이러한 함수는 사전을 반복하는 데 가장 일반적이고 유용한 함수입니다.
print("Keys of Student dictionary: ", student.keys())
print("Values of Student dictionary: ", student.values())
print("Items of Student dictionary: ", student.items())
출력:
Keys of Student dictionary: dict_keys(['name', 'degree', 'section'])
Values of Student dictionary: dict_values(['Zeeshan Afridi', 'BSSE', 'A'])
Items of Student dictionary: dict_items([('name', 'Zeeshan Afridi'), ('degree', 'BSSE'), ('section', 'A')])
Python 사전에서 ValueError: not enough values to unpack
이 발생하는 이유를 살펴보겠습니다.
student = {
# Keys : Values
"name": "Zeeshan Afridi",
"degree": "BSSE",
"section": "A",
}
# iterate user dictionary
for k, v, l in student.items(): # This statement throws an error
print("Key:", k)
print("Value:", str(v))
출력:
ValueError: not enough values to unpack (expected 3, got 2)
보시다시피 .items()
함수는 student
사전의 키와 값을 캐치할 두 개의 변수를 예상하기 때문에 위의 코드에서 오류가 발생했지만 우리는 k,v,l
세 개의 변수를 제공했습니다. .
따라서 student
사전에는 l
을 위한 공간이 없으며 ValueError: not enough values to unpack (expected 3, got 2)
가 발생합니다.
이를 수정하려면 사전의 변수를 수정해야 합니다.
for k, v in student.items():
이것은 Python에서 사전을 반복하는 올바른 명령문입니다.
Python에서 ValueError: not enough values to unpack
수정
Python에서 이러한 예외를 방지하려면 변수에 예상되는 값 수를 제공하고 양식이나 텍스트 필드에 데이터를 입력하는 동안 유용한 메시지를 표시해야 합니다.
그 외에도 try-catch
블록을 사용하여 프로그램이 충돌하기 전에 이러한 오류를 캡처할 수 있습니다.
Python에서 ValueError: not enough values to unpack
을 수정하는 방법을 이해합시다.
# User message --> Enter three numbers to multiply ::
x, y, z = input("Enter three numbers to multiply :: ").split(",")
# type casting x,y, and z
x = int(x)
y = int(y)
z = int(z)
prod = x * y * z
print("The product of x,y and z is :: ", prod)
출력:
Enter three numbers to multiply :: 2,2,2
The product of x,y and z is :: 8
이 예에서 input
문은 3개의 입력을 예상하고 예상 입력 수를 제공했으므로 ValueError
가 발생하지 않았습니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn관련 문장 - Python Error
- AttributeError 수정: Python에서 'generator' 객체에 'next' 속성이 없습니다.
- AttributeError 해결: 'list' 객체 속성 'append'는 읽기 전용입니다.
- AttributeError 해결: Python에서 'Nonetype' 객체에 'Group' 속성이 없습니다.
- AttributeError: 'Dict' 객체에 Python의 'Append' 속성이 없습니다.
- AttributeError: 'NoneType' 객체에 Python의 'Text' 속성이 없습니다.
- AttributeError: Int 객체에 속성이 없습니다.