How to Fix Python ValueError: No JSON Object Could Be Decoded
- Decode JSON Object in Python
- Decode JSON String Into a Python Object
- Encode Python Object Into a JSON String
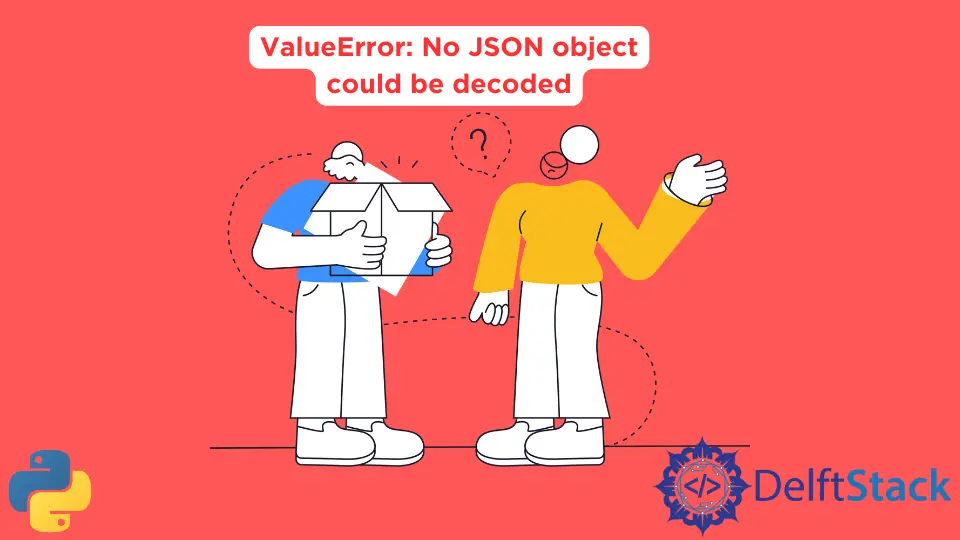
We will discuss the name error, how to encode a Python object into a JSON, and decode an adjacent string into a Python object. We also go to learn why we fail to parse JSON data.
Decode JSON Object in Python
Let’s get started by importing the json
module, and for this session, we are planning to encode and decode Python objects into adjacent text. So we will switch to the next line and define a variable that will store an entire little string where we will have some key-value pairs.
When we print this, we see it is printed as we defined in the variable, meaning it is a string.
Code:
import json
Sample_json = '{"Employee_Name":"Garry","Employee_Age":29}'
print(Sample_json)
# We can see the type of this Sample_json using the type() function
print(type(Sample_json))
Output:
{"Employee_Name":"Garry","Employee_Age":29}
<class 'str'>
Decode JSON String Into a Python Object
Now we needed to decode it into a Python object, and it exactly converts into a Python dictionary. We will use the json.loads()
method to decode adjacent strings, and at the same time, we will also print the type of object.
import json
Sample_obj = json.loads(Sample_json)
print(Sample_obj)
print(type(Sample_obj))
Output:
{'Employee_Name': 'Garry', 'Employee_Age': 29}
<class 'dict'>
Encode Python Object Into a JSON String
Now we have seen a JSON string can be decoded into a Python dictionary object with the help of the json.loads()
method. Let’s have another example where we will convert a Python object or how to encode a Python object into a JSON string.
Let’s define one more object called Sample_json2
, which will be a dictionary. To convert that into JSON, we use the json.dumps()
method.
Then we will provide the object we want to encode into a JSON string. Now we can see the type of output that the dumps()
method generated, and we can see that its type is str
(string).
Code:
import json
Sample_json2 = {"Employee_Name": "Garry", "Employee_Age": 29}
print(Sample_json2)
print(type(Sample_json2))
temp = json.dumps(Sample_json2)
print(temp)
print(type(temp))
Output:
{'Employee_Name': 'Garry', 'Employee_Age': 29}
<class 'dict'>
{"Employee_Name": "Garry", "Employee_Age": 29}
<class 'str'>
The dumps()
method encodes a Python object into an adjacent string, and the loads()
method decodes a JSON string into a Python object. If we follow this approach, we will not get the value error we sometimes get when we parse the JSON data.
There could be many reasons to be the failure to parse JSON data in python, one of them is sometimes we are trying to decode an empty string or empty file, or maybe we are giving the wrong path of the JSON file.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python